I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
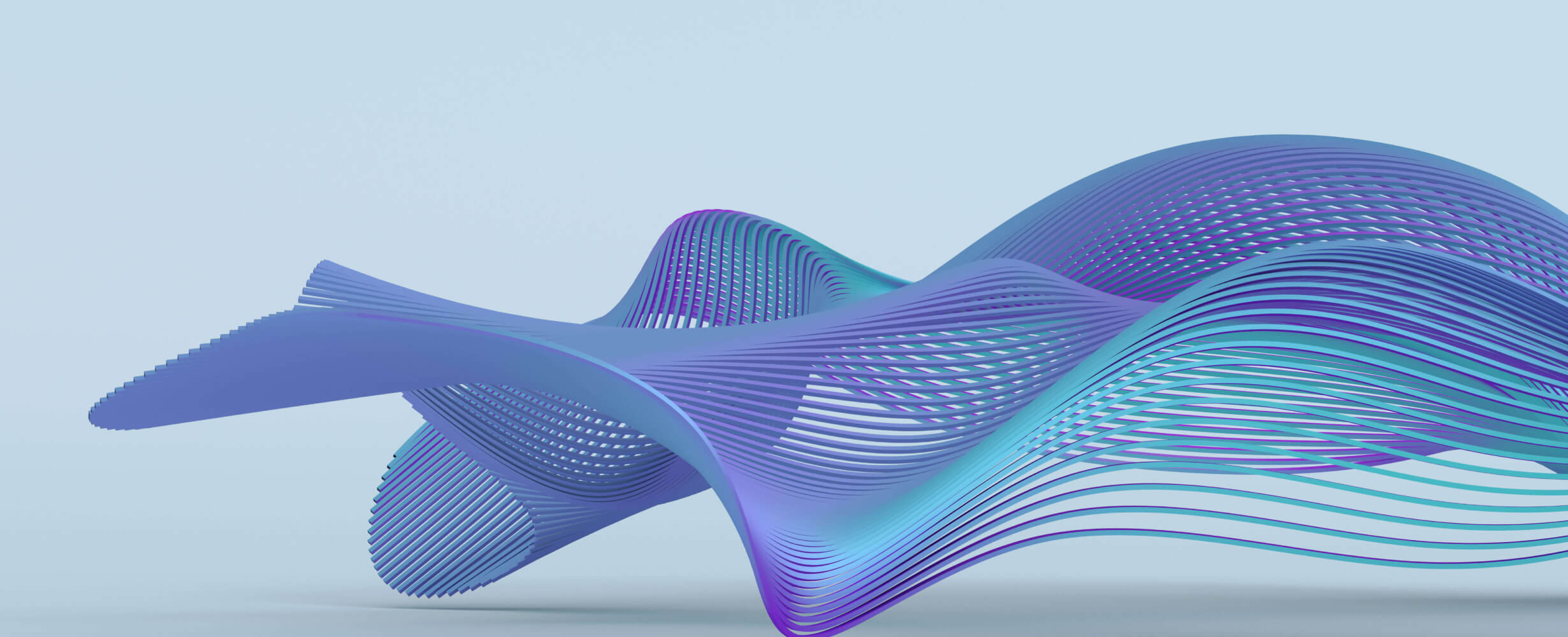
learn-mcp-by-building
Learn MCP by building from Scarch
1
Github Watches
1
Github Forks
0
Github Stars
Model Context Protocol (MCP) Implementation
This project implements the Model Context Protocol (MCP) for building AI tools. It provides a modular framework that can be used to create MCP-compatible servers and clients.
🔍 What is Model Context Protocol (MCP)?
The Model Context Protocol (MCP) is an open protocol that enables AI assistants to interact with external tools and data sources.
Key Features
- List available tools and their capabilities
- Call tools with parameters
- Handle errors in a consistent way
- Process tool results in a standardized format
📚 For a detailed overview, see MCP Notes.
✨ Features
Category | Features |
---|---|
Core | ✅ JSON-RPC 2.0 message handling ✅ Protocol initialization ✅ Capability negotiation |
Tools | ✅ Tool registration with JSON Schema ✅ Tool invocation and validation ✅ Standardized error handling |
Transport | ✅ STDIO support ✅ HTTP+SSE Support |
Testing | ✅ Test clients |
📁 Project Structure
src/
├── core/ # Core MCP server implementation
├── transports/ # Transport layer implementations (stdio, HTTP+SSE)
├── tools/ # Tool definitions and handlers
├── examples/ # Example servers and clients
│ └── public/ # Static files for HTTP server
└── index.js # Main entry point for the library
🚀 Getting Started
Prerequisites
- Node.js 20.x or later
- npm or pnpm
Installation
# Clone the repository
git clone https://github.com/AshikNesin/learn-mcp-by-building
cd learn-mcp-by-building
# Install dependencies
npm install
# or
pnpm install
🏃♂️ Running the Examples
STDIO Server and Client
Run the STDIO server:
npm run server:stdio
# or
node src/examples/stdio-server.js
Test with the STDIO client:
npm run client:stdio
# or
node src/examples/stdio-client.js
Run both together to see a complete test:
npm run test:stdio
# or
node src/examples/stdio-client.js | node src/examples/stdio-server.js
HTTP+SSE Server and Client
Run the HTTP+SSE server:
npm run server:sse
# or
node src/examples/http-sse-server.js --port 5000
Available options:
-
--port
: Port to listen on (default: 5000) -
--host
: Host to bind to (default: localhost) -
--path
: Endpoint path (default: /sse) -
--cors
: Enable CORS (default: true) -
--serve-static
: Serve static files from src/examples/public (default: true)
Test with the HTTP+SSE client:
npm run client:sse
# or
node src/examples/http-sse-client.js --server http://localhost:5000/sse
Once running, you can also access the web-based client interface in your browser at http://localhost:5000:
The interface provides a user-friendly way to interact with the MCP server, with a side-by-side layout showing the calculator controls and real-time logs.
🔍 Using the MCP Inspector
You can use the official MCP Inspector to debug the server:
npm run debug
The MCP Inspector provides a visual interface for monitoring and debugging MCP servers:
🧮 Calculator Tool
Operations
|
Parameters
|
Error Handling
|
🧑💻 Developing with the MCP Framework
Creating a New Server
import { McpServer } from '../core/index.js';
import { StdioTransport } from '../transports/index.js';
import { calculatorToolDefinition, handleCalculatorTool } from '../tools/index.js';
// Create server instance
const server = new McpServer(
{ name: 'my-server', version: '1.0.0' },
{ capabilities: { tools: { listChanged: true } } }
);
// Register tool handlers
server.setRequestHandler('tools/list', () => ({ tools: [calculatorToolDefinition] }));
server.setRequestHandler('tools/call', async (params) => {
if (params.name === 'calculator') {
return handleCalculatorTool(params.arguments);
}
throw new Error(`Tool ${params.name} not found`);
});
// Start the server
const transport = new StdioTransport();
server.connect(transport)
.then(() => console.error('Server ready!'));
Creating a New Tool
- Create a new file in
src/tools/
:
// src/tools/my-tool.js
export const myToolDefinition = {
name: 'my-tool',
description: 'Description of my tool',
inputSchema: {
type: 'object',
properties: {
// Define parameters
},
required: []
}
};
export async function handleMyTool(args) {
// Implement tool logic
return {
content: [
{
type: 'text',
text: 'Result from my tool'
}
]
};
}
- Export the tool in
src/tools/index.js
:
export * from './my-tool.js';
🛠️ Protocol Features
- ✅ Capability negotiation
- ✅ Tool list change notifications
- ✅ Standardized error handling
- ✅ JSON Schema validation
- ✅ Structured tool results
- ✅ Transport layer abstraction
📚 External Resources
📝 License
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
I find academic articles and books for research and literature reviews.
Emulating Dr. Jordan B. Peterson's style in providing life advice and insights.
Confidential guide on numerology and astrology, based of GG33 Public information
Your go-to expert in the Rust ecosystem, specializing in precise code interpretation, up-to-date crate version checking, and in-depth source code analysis. I offer accurate, context-aware insights for all your Rust programming questions.
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
Reviews
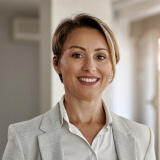
user_Hb9D1Yki
"Learn-mcp-by-building by AshikNesin is an exceptional educational resource for anyone interested in mastering MCP. The hands-on approach and comprehensive tutorials make learning both engaging and effective. I highly recommend it to both beginners and advanced users looking to expand their knowledge. Check it out on GitHub and start your learning journey today!"