I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
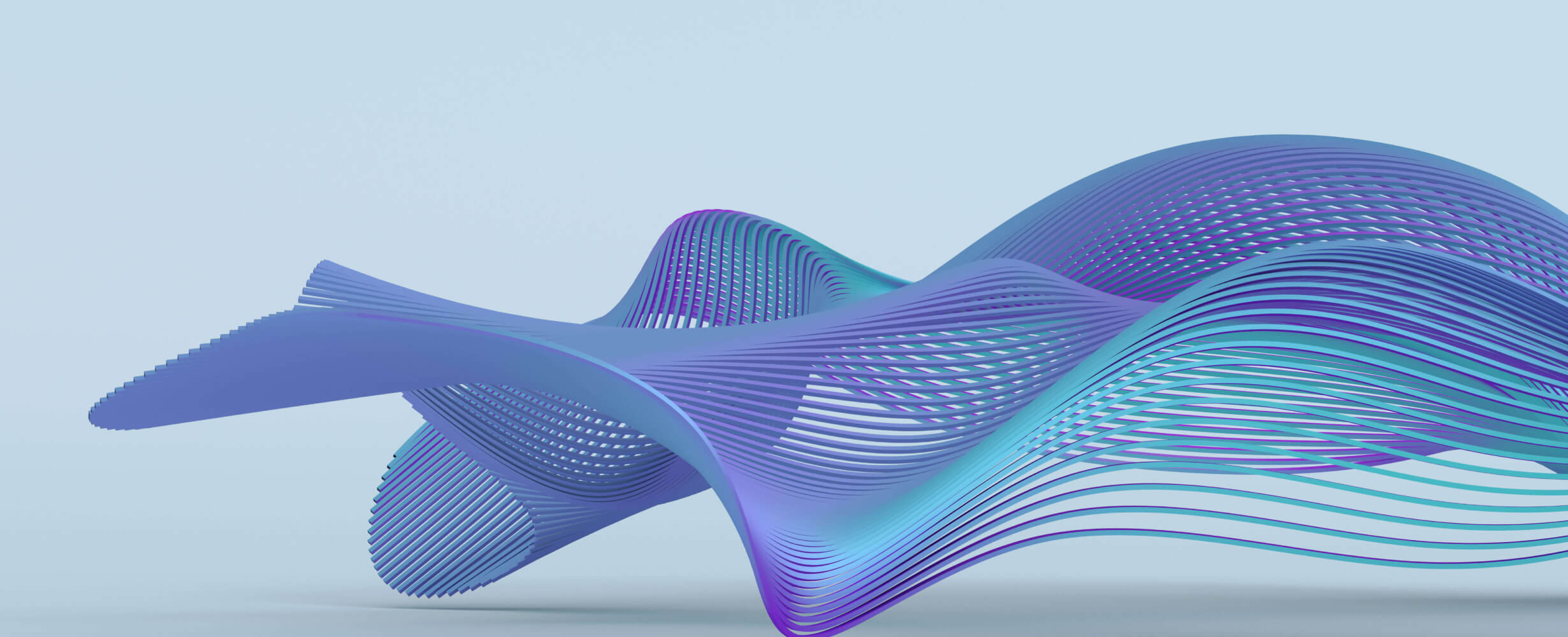
MCP-Server-Firebase
3 years
Works with Finder
1
Github Watches
1
Github Forks
0
Github Stars
MCP Server Firebase
這是一個基於 Firebase 的 Model Context Protocol (MCP) 服務器實現,提供兩種運行模式:
- 本地 Node.js 模式 (使用 Firebase Admin SDK)
- Cloudflare Workers 模式 (使用 Firebase REST API)
功能
- Firestore 資料庫操作 (讀取、寫入、更新、刪除文檔)
- 集合和子集合的列表查詢
- 支援過濾條件的查詢
必要條件
- Node.js 18 或更高版本
- Firebase 專案
- Firebase 服務帳號 (用於本地模式)
- Firebase Web API 密鑰 (用於 Cloudflare Workers 模式)
安裝
- 克隆此專案:
git clone https://github.com/[您的用戶名]/mcp-server-firebase.git
cd mcp-server-firebase
- 安裝依賴:
npm install
配置
本地模式配置 (Firebase Admin SDK)
-
從 Firebase 控制台下載服務帳號金鑰檔案:
- 前往 Firebase 控制台 > 專案設置 > 服務帳號 > 生成新的私鑰
-
將下載的 JSON 文件保存到專案目錄中 (檔名不重要,但請記住路徑)
-
設置環境變量:
export SERVICE_ACCOUNT_KEY_PATH="/path/to/your-service-account.json"
Cloudflare Workers 模式配置
-
更新 wrangler.toml 文件中的環境變量:
- FIREBASE_PROJECT_ID: 您的 Firebase 專案 ID
- FIREBASE_API_KEY: 您的 Firebase Web API 密鑰
- FIREBASE_AUTH_DOMAIN: 通常是
[PROJECT_ID].firebaseapp.com
-
或者使用 Cloudflare Secrets 設置環境變量:
npx wrangler secret put FIREBASE_PROJECT_ID
npx wrangler secret put FIREBASE_API_KEY
npx wrangler secret put FIREBASE_AUTH_DOMAIN
運行
本地模式 (Firebase Admin SDK)
# 編譯 TypeScript 並運行服務器
npm run dev
或使用提供的腳本設置環境變量並運行:
# 互動式設置 Firebase 環境變量
node setup-firebase-env.js
# 根據腳本提示運行後續命令
Cloudflare Workers 模式 (本地開發)
# 編譯 TypeScript 並在本地運行 Workers
npm run dev:worker
同時運行兩種模式
npm run dev:all
部署
部署到 Cloudflare Workers
# 部署到開發環境
npm run deploy:worker
# 部署到生產環境
npm run deploy:production
目錄結構
-
src/index.ts
- 本地 Node.js 模式的入口點 -
src/worker.ts
- Cloudflare Workers 模式的入口點 -
src/mcp/
- MCP 服務器實現代碼 -
src/lib/
- 公共工具函數
使用方法
連接到 MCP 服務器
在您的客戶端應用中,使用 MCP SDK 連接到服務器:
// 本地模式連接
const mcp = createMcpClient({
endpoint: "http://localhost:8765"
});
// Cloudflare Workers 模式連接
const mcp = createMcpClient({
endpoint: "https://your-worker.workers.dev"
});
使用 MCP 工具操作 Firestore
// 添加文檔
const result = await mcp.tools.mcp_firebase_mcp_firestore_add_document({
documentPath: "collection/documentId",
data: { field1: "value1", field2: 42 }
});
// 獲取文檔
const doc = await mcp.tools.mcp_firebase_mcp_firestore_get_document({
documentPath: "collection/documentId"
});
// 更新文檔
await mcp.tools.mcp_firebase_mcp_firestore_update_document({
documentPath: "collection/documentId",
data: { field1: "updated value" }
});
// 刪除文檔
await mcp.tools.mcp_firebase_mcp_firestore_delete_document({
documentPath: "collection/documentId"
});
// 列出集合中的文檔
const docs = await mcp.tools.mcp_firebase_mcp_firestore_list_documents({
collectionPath: "collection",
filters: [
{ field: "field2", operator: ">", value: 40 }
]
});
// 列出集合
const collections = await mcp.tools.mcp_firebase_mcp_firestore_list_collections({
parentPath: "collection/documentId" // 可選
});
安全注意事項
- 請勿將服務帳號金鑰或 API 密鑰提交到 Git 存儲庫
- 在部署前確保所有敏感信息已設置為環境變量或 Secrets
- 為 Firestore 設置適當的安全規則
注意事項
- src/worker.ts 是 Cloudflare Workers 部署版本
- src/index.ts 是本地運行版本
- 請確保 Firebase 服務帳號具有適當的權限
許可證
此專案使用 MIT 許可證。
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
A geek-themed horoscope generator blending Bitcoin prices, tech jargon, and astrological whimsy.
Therapist adept at identifying core issues and offering practical advice with images.
Reviews
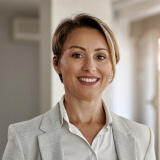
user_oHwLcrsL
I've been using mcp-server-firebase by sheep52031, and it's fantastic! The integration with Firebase is seamless, and the documentation provided on the GitHub page is thorough and easy to follow. This server application has dramatically improved my workflow and productivity. I highly recommend it to anyone looking for a reliable Firebase server solution. Sheep52031 has done an excellent job in crafting this tool!