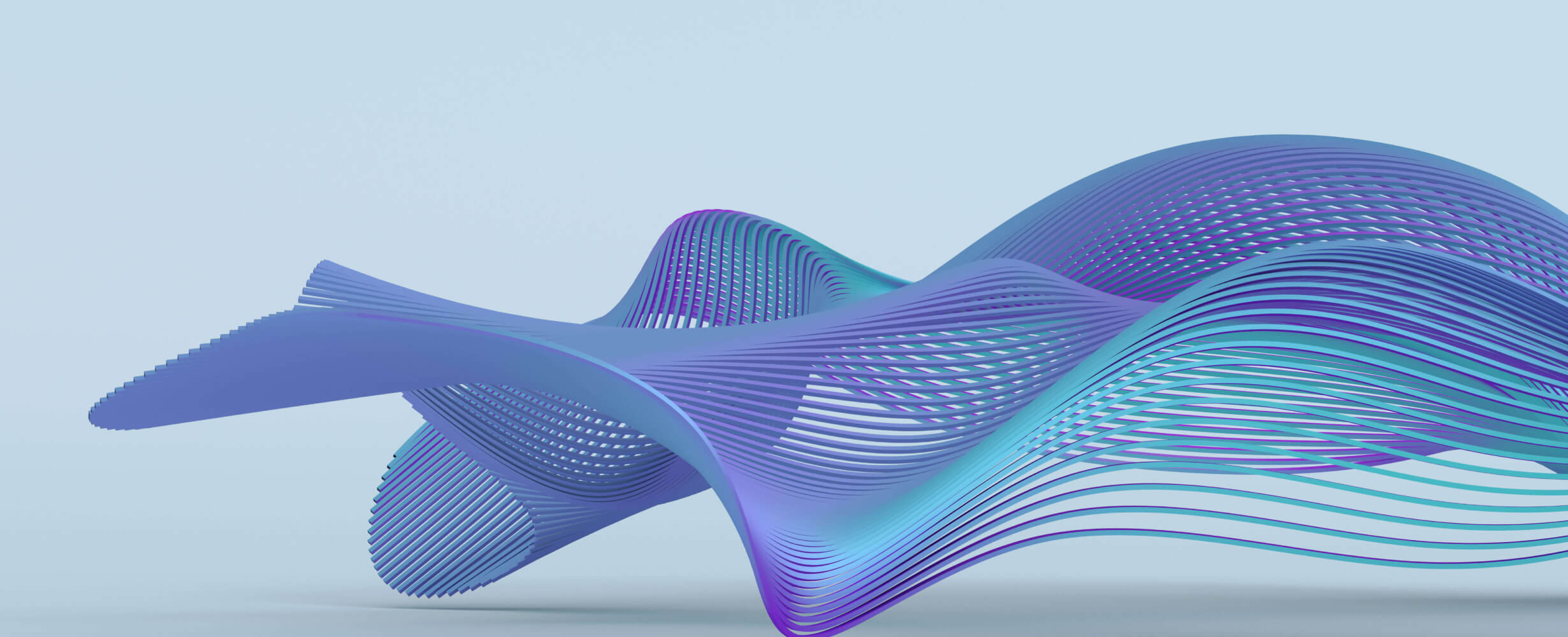
NestJS MCP Server Module
A NestJS module to effortlessly expose tools, resources, and prompts for AI, from your NestJS applications using the Model Context Protocol (MCP).
@rekog/mcp-nest
handles the complexity of setting up MCP servers. You define tools, resources, and prompts in a way that's familiar in NestJS and leverage the full power of dependency injection to utilize your existing services.
Features
- 🚀 HTTP+SSE and Streamable HTTP Transport
- 🔍 Automatic
tool
,resource
, andprompt
discovery and registration - 💯 Zod-based request validation
- 📊 Progress notifications
- 🔒 Guard-based authentication
Installation
npm install @rekog/mcp-nest @modelcontextprotocol/sdk zod
Quick Start
1. Import Module
// app.module.ts
import { Module } from '@nestjs/common';
import { McpModule } from '@rekog/mcp-nest';
import { GreetingTool } from './greeting.tool';
@Module({
imports: [
McpModule.forRoot({
name: 'my-mcp-server',
version: '1.0.0',
}),
],
providers: [GreetingTool],
})
export class AppModule {}
2. Define Tools and Resource
// greeting.tool.ts
import { Injectable } from '@nestjs/common';
import { Tool, Resource, Context } from '@rekog/mcp-nest';
import { z } from 'zod';
import { Progress } from '@modelcontextprotocol/sdk/types';
@Injectable()
export class GreetingTool {
constructor() {}
@Tool({
name: 'hello-world',
description:
'Returns a greeting and simulates a long operation with progress updates',
parameters: z.object({
name: z.string().default('World'),
}),
})
async sayHello({ name }, context: Context) {
const greeting = `Hello, ${name}!`;
const totalSteps = 5;
for (let i = 0; i < totalSteps; i++) {
await new Promise((resolve) => setTimeout(resolve, 500));
// Send a progress update.
await context.reportProgress({
progress: (i + 1) * 20,
total: 100,
} as Progress);
}
return {
content: [{ type: 'text', text: greeting }],
};
}
@Resource({
uri: 'mcp://hello-world/{userName}',
name: 'Hello World',
description: 'A simple greeting resource',
mimeType: 'text/plain',
})
// Different from the SDK, we put the parameters and URI in the same object.
async getCurrentSchema({ uri, userName }) {
return {
content: [
{
uri,
text: `User is ${userName}`,
mimeType: 'text/plain',
},
],
};
}
}
You are done!
API Endpoints
-
GET /sse
: SSE connection endpoint (Protected by guards if configured) -
POST /messages
: Tool execution endpoint (Protected by guards if configured)
Tips
It's possible to use the module with global prefix, but the recommended way is to exclude those endpoints with:
app.setGlobalPrefix('/api', { exclude: ['sse', 'messages'] });
Authentication
You can secure your MCP endpoints using standard NestJS Guards.
1. Create a Guard
Implement the CanActivate
interface. The guard should handle request validation (e.g., checking JWTs, API keys) and optionally attach user information to the request object.
Nothing special, check the NestJS documentation for more details.
2. Apply the Guard
Pass your guard(s) to the McpModule.forRoot
configuration. The guard(s) will be applied to both the /sse
and /messages
endpoints.
// app.module.ts
import { Module } from '@nestjs/common';
import { McpModule } from '@rekog/mcp-nest';
import { GreetingTool } from './greeting.tool';
import { AuthGuard } from './auth.guard';
@Module({
imports: [
McpModule.forRoot({
name: 'my-mcp-server',
version: '1.0.0',
guards: [AuthGuard], // Apply the guard here
}),
],
providers: [GreetingTool, AuthGuard], // Ensure the Guard is also provided
})
export class AppModule {}
That's it! The rest is the same as NestJS Guards.
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Siri Shortcut Finder – your go-to place for discovering amazing Siri Shortcuts with ease
Découvrez la collection la plus complète et la plus à jour de serveurs MCP sur le marché. Ce référentiel sert de centre centralisé, offrant un vaste catalogue de serveurs MCP open-source et propriétaires, avec des fonctionnalités, des liens de documentation et des contributeurs.
Manipulation basée sur Micropython I2C de l'exposition GPIO de la série MCP, dérivée d'Adafruit_MCP230XX
L'application tout-en-un desktop et Docker AI avec chiffon intégré, agents AI, constructeur d'agent sans code, compatibilité MCP, etc.
Un puissant plugin Neovim pour gérer les serveurs MCP (Protocole de contexte modèle)
MCP Server pour récupérer le contenu de la page Web à l'aide du navigateur sans tête du dramwright.
Pont entre les serveurs Olllama et MCP, permettant aux LLM locaux d'utiliser des outils de protocole de contexte de modèle
La communauté du curseur et de la planche à voile, recherchez des règles et des MCP
🧑🚀 全世界最好的 LLM 资料总结 (数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Résumé des meilleures ressources LLM du monde.
Plateforme d'automatisation de workflow à code équitable avec des capacités d'IA natives. Combinez le bâtiment visuel avec du code personnalisé, de l'auto-hôte ou du cloud, 400+ intégrations.
Une liste organisée des serveurs de protocole de contexte de modèle (MCP)
Reviews
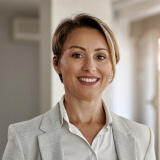
user_2w4m2csp
MCP-Nest by rekog-labs is a fantastic tool for building and managing microservices. Its efficient structure and support make the development process seamless. Highly recommended for developers looking for a robust solution to streamline their workflow. Check it out at https://github.com/rekog-labs/MCP-Nest.