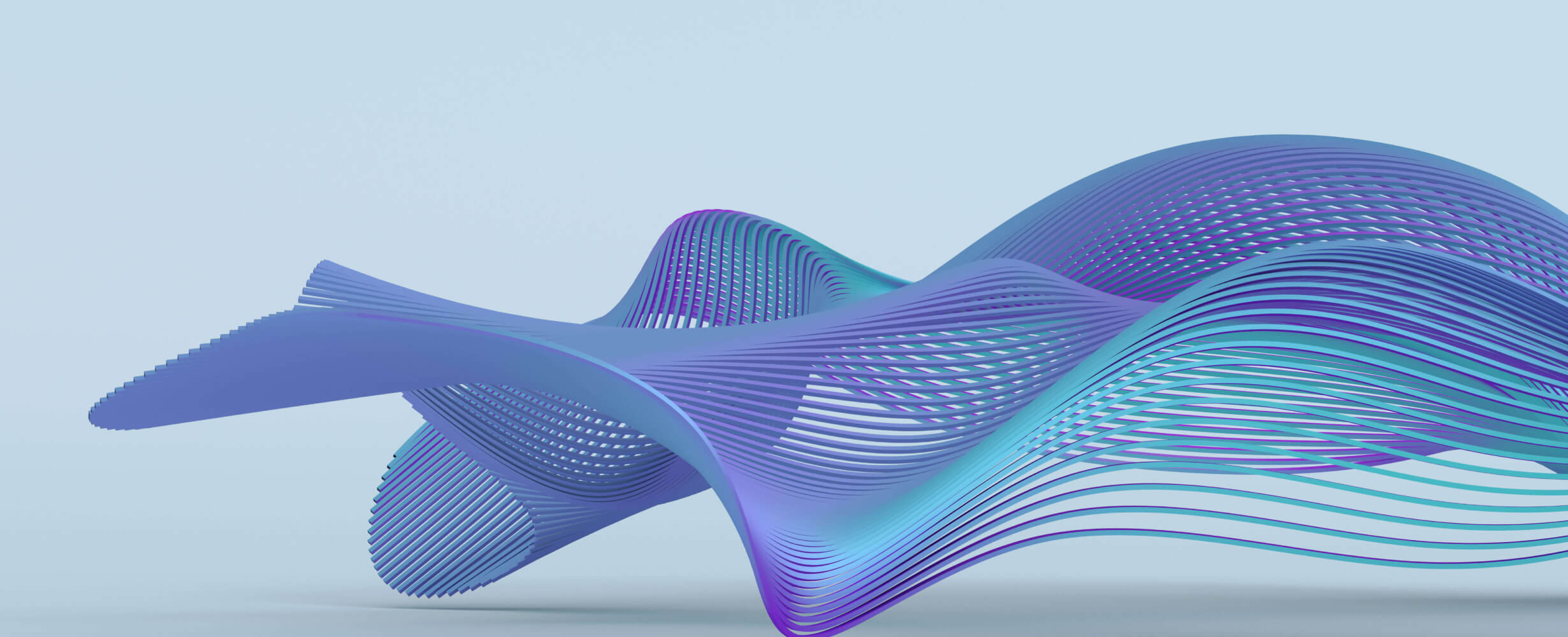
MCP_DART
MCP SDK的DART实施
3
Github Watches
7
Github Forks
25
Github Stars
MCP(Model Context Protocol) for Dart
Model Context Protocol (MCP) is an open protocol designed to enable seamless integration between LLM applications and external data sources and tools.
This library aims to provide a simple and intuitive way to implement MCP servers and clients in Dart, while adhering to the MCP protocol spec. The goal is to make this SDK as similar as possible to the official SDKs available in other languages, ensuring a consistent developer experience across platforms.
Requirements
- Dart SDK version ^3.0.0 or higher
Ensure you have the correct Dart SDK version installed. See https://dart.dev/get-dart for installation instructions.
Features
- Stdio support (Server and Client)
- SSE support (Server only for now)
- Tools
- Resources
- Prompts
- Sampling
- Roots
Getting started
Below code is the simplest way to start the MCP server.
import 'package:mcp_dart/mcp_dart.dart';
void main() async {
McpServer server = McpServer(
Implementation(name: "mcp-example-server", version: "1.0.0"),
options: ServerOptions(
capabilities: ServerCapabilities(
resources: ServerCapabilitiesResources(),
tools: ServerCapabilitiesTools(),
),
),
);
server.tool(
"calculate",
description: 'Perform basic arithmetic operations',
inputSchemaProperties: {
'operation': {
'type': 'string',
'enum': ['add', 'subtract', 'multiply', 'divide'],
},
'a': {'type': 'number'},
'b': {'type': 'number'},
},
callback: ({args, extra}) async {
final operation = args!['operation'];
final a = args['a'];
final b = args['b'];
return CallToolResult(
content: [
TextContent(
text: switch (operation) {
'add' => 'Result: ${a + b}',
'subtract' => 'Result: ${a - b}',
'multiply' => 'Result: ${a * b}',
'divide' => 'Result: ${a / b}',
_ => throw Exception('Invalid operation'),
},
),
],
);
},
);
server.connect(StdioServerTransport());
}
Usage
Once you compile your MCP server, you can compile the client using the below code.
dart compile exe example/server_stdio.dart -o ./server_stdio
Or just run it with JIT.
dart run example/server_stdio.dart
To configure it with the client (ex, Claude Desktop), you can use the below code.
{
"mcpServers": {
"calculator_jit": {
"command": "path/to/dart",
"args": [
"/path/to/server_stdio.dart"
]
},
"calculator_aot": {
"command": "path/to/compiled/server_stdio",
},
}
}
More examples
https://github.com/leehack/mcp_dart/tree/main/example
Credits
This library is inspired by the following projects:
相关推荐
Confidential guide on numerology and astrology, based of GG33 Public information
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Reviews
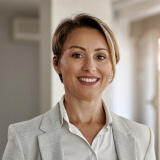
user_pRC1FAyT
I've been using mcp_dart by leehack for a while now, and it's truly a game-changer! The application's intuitive design and seamless integration are remarkable. It makes managing projects so much easier. The comprehensive documentation provided on the GitHub page is incredibly helpful. Highly recommend checking it out: https://github.com/leehack/mcp_dart