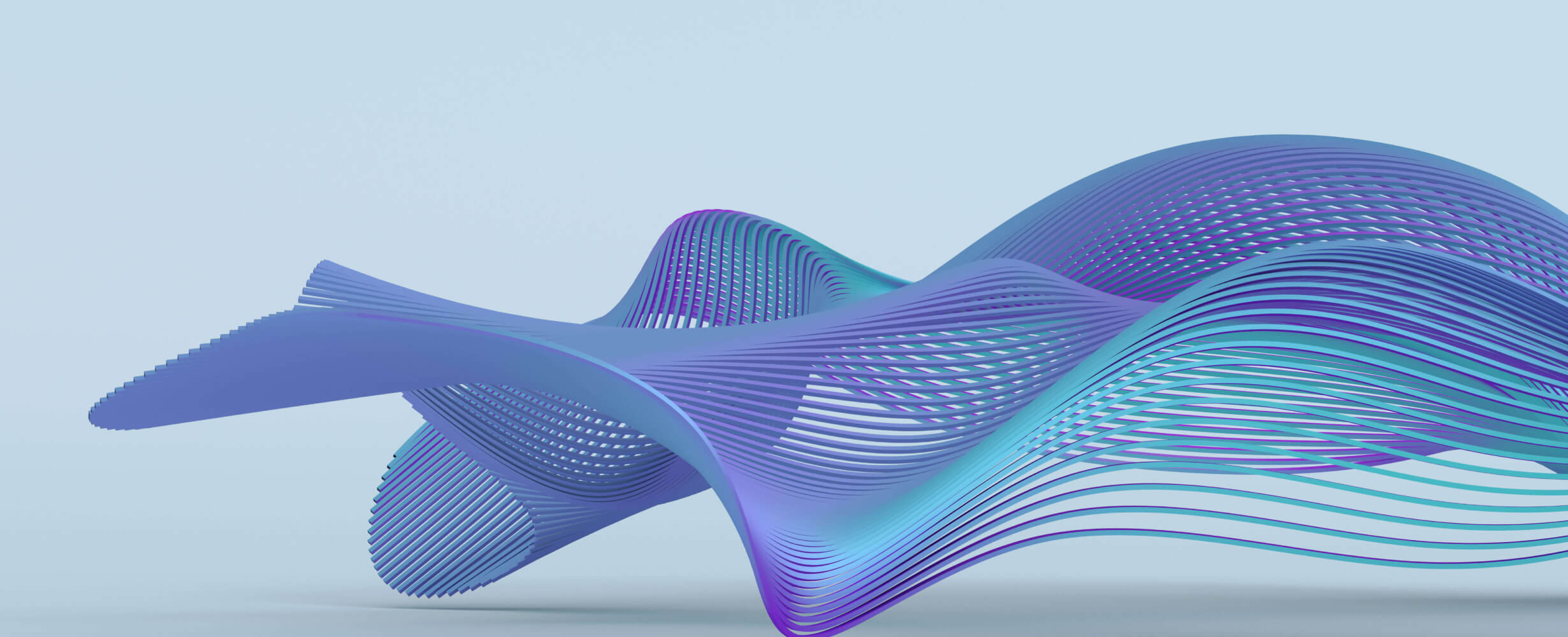
Unitymcp
Unity编辑器与模型上下文协议(MCP)的集成使Claude这样的AI助手与Unity项目互动。具有带有可扩展命令处理程序架构,TCP/IP通信和动态插件发现的打字稿MCP服务器和C#Unity插件。
24
Github Watches
0
Github Forks
24
Github Stars
Unity MCP 統合フレームワーク
Unity と Model Context Protocol (MCP) を統合するための拡張可能なフレームワークです。このフレームワークにより、Claude などの AI 言語モデルがスケーラブルなハンドラーアーキテクチャを通じて Unity エディタと直接対話することができます。
🌟 特徴
- 拡張可能なプラグインアーキテクチャ: カスタムハンドラーを作成・登録して機能を拡張
- 完全なMCP統合: コマンド・リソース・プロンプトの全MCP基本機能をサポート
- TypeScript & C# サポート: サーバーコンポーネントは TypeScript、Unity コンポーネントは C#
- エディタ統合: カスタマイズ可能な設定を持つエディタツールとして動作
- 自動検出: 各種ハンドラーの自動検出と登録
- 通信: Unity と外部 AI サービス間の TCP/IP ベースの通信
📋 必要条件
- Unity 2022.3.22f1 以上(Unity6 にも対応)
- 2022.3.22f1, 2023.2.19f1, 6000.0.35f1で動作確認
- .NET/C# 9.0
- Node.js 18.0.0 以上と npm(TypeScript サーバー用)
- Node.js 公式サイトからインストールしてください
🚀 はじめに
インストール方法
- Unity パッケージマネージャーを使用してインストール:
- パッケージマネージャーを開く (Window > Package Manager)
- 「+」ボタンをクリック
- 「Add package from git URL...」を選択
- 入力:
https://github.com/isuzu-shiranui/UnityMCP.git?path=jp.shiranui-isuzu.unity-mcp
クイックセットアップ
- Unity を開き、Edit > Preferences > Unity MCP に移動
- 接続設定 (ホストとポート) を構成
- 「Connect」ボタンをクリックして接続の待ち受けを開始
Claude Desktop との連携
- リリースページから最新のZIPファイルをダウンロードして解凍します
-
build/index.js
ファイルのフルパスを控えておきます - Claude Desktop の設定ファイル
claude_desktop_config.json
を開きます - 以下の内容を追加して保存します:
{
"mcpServers": {
"unity-mcp": {
"command": "node",
"args": [
"path/to/index.js"
]
}
}
}
※ path/to/index.js
は実際のパスに置き換えてください(Windowsの場合はバックスラッシュをエスケープ"\\"するか、フォワードスラッシュ"/"を使用)
🔌 アーキテクチャ
Unity MCP フレームワークは主に 2 つのコンポーネントで構成されています:
1. Unity C# プラグイン
- McpServer: TCP 接続をリッスンしコマンドをルーティングするコアサーバー
- IMcpCommandHandler: カスタムコマンドハンドラーを作成するためのインターフェース
- IMcpResourceHandler: データ提供リソースを作成するためのインターフェース
- McpSettings: プラグイン設定を管理
- McpServiceManager: サービス管理のための依存性注入システム
- McpHandlerDiscovery: 各種ハンドラーを自動検出して登録
2. TypeScript MCP クライアント
- HandlerAdapter: 各種ハンドラーを MCP SDK に適応させる
- HandlerDiscovery: ハンドラー実装の検出と登録
- UnityConnection: Unity との TCP/IP 通信を管理
- BaseCommandHandler: コマンドハンドラー実装のベースクラス
- BaseResourceHandler: リソースハンドラー実装のベースクラス
- BasePromptHandler: プロンプトハンドラー実装のベースクラス
📄 MCP ハンドラータイプ
Unity MCPでは、Model Context Protocol (MCP) に基づく以下の3種類のハンドラータイプをサポートしています:
1. コマンドハンドラー(Tools)
- 用途: アクションを実行するためのツール(Unity側で何かを実行させる)
- 制御: モデル制御型 - AIモデルが自動的に呼び出せる
- 実装: IMcpCommandHandler インターフェースを実装
2. リソースハンドラー(Resources)
- 用途: Unity内のデータにアクセスするためのリソース(情報提供)
- 制御: アプリケーション制御型 - クライアントアプリが使用を決定
- 実装: IMcpResourceHandler インターフェースを実装
3. プロンプトハンドラー(Prompts)
- 用途: 再利用可能なプロンプトテンプレートやワークフロー
- 制御: ユーザー制御型 - ユーザーが明示的に選択して使用
- 実装: IPromptHandler インターフェースを実装(TypeScript側のみ)
🔬 サンプルコード
パッケージには以下のサンプルが含まれています:
-
Unity MCP Handler Samples
- C#実装のサンプルコード
- そのままプロジェクトにインポートして使用可能
-
Unity MCP Handler Samples JavaScript
- JavaScript実装のサンプルコード
- この中のJSファイルは
build/handlers
ディレクトリにコピーして使用してください
⚠️ 注意: サンプルコードには任意コード実行機能が含まれています。本番環境での使用には十分注意してください。
サンプルのインポート方法:
- Unity パッケージマネージャーで本パッケージを選択
- 「Samples」タブをクリック
- 必要なサンプルの「Import」ボタンをクリック
🛠️ カスタムハンドラーの作成
コマンドハンドラー (C#)
IMcpCommandHandler
を実装する新しいクラスを作成:
using Newtonsoft.Json.Linq;
using UnityMCP.Editor.Core;
namespace YourNamespace.Handlers
{
internal sealed class YourCommandHandler : IMcpCommandHandler
{
public string CommandPrefix => "yourprefix";
public string Description => "ハンドラーの説明";
public JObject Execute(string action, JObject parameters)
{
// コマンドロジックを実装
if (action == "yourAction")
{
// パラメータを使って何かを実行
return new JObject
{
["success"] = true,
["result"] = "結果データ"
};
}
return new JObject
{
["success"] = false,
["error"] = $"不明なアクション: {action}"
};
}
}
}
リソースハンドラー (C#)
IMcpResourceHandler
を実装する新しいクラスを作成:
using Newtonsoft.Json.Linq;
using UnityMCP.Editor.Resources;
namespace YourNamespace.Resources
{
internal sealed class YourResourceHandler : IMcpResourceHandler
{
public string ResourceName => "yourresource";
public string Description => "リソースの説明";
public string ResourceUri => "unity://yourresource";
public JObject FetchResource(JObject parameters)
{
// リソースデータを取得する処理を実装
var data = new JArray();
// 何かデータを取得・加工してJArrayに追加
data.Add(new JObject
{
["name"] = "項目1",
["value"] = "値1"
});
return new JObject
{
["success"] = true,
["items"] = data
};
}
}
}
コマンドハンドラー (TypeScript)
BaseCommandHandler
を拡張して新しいハンドラーを作成:
import { IMcpToolDefinition } from "../core/interfaces/ICommandHandler.js";
import { JObject } from "../types/index.js";
import { z } from "zod";
import { BaseCommandHandler } from "../core/BaseCommandHandler.js";
export class YourCommandHandler extends BaseCommandHandler {
public get commandPrefix(): string {
return "yourprefix";
}
public get description(): string {
return "ハンドラーの説明";
}
public getToolDefinitions(): Map<string, IMcpToolDefinition> {
const tools = new Map<string, IMcpToolDefinition>();
// ツールを定義
tools.set("yourprefix_yourAction", {
description: "アクションの説明",
parameterSchema: {
param1: z.string().describe("パラメータの説明"),
param2: z.number().optional().describe("オプションパラメータ")
},
annotations: {
title: "ツールのタイトル",
readOnlyHint: true,
openWorldHint: false
}
});
return tools;
}
protected async executeCommand(action: string, parameters: JObject): Promise<JObject> {
// コマンドロジックを実装
// リクエストを Unity に転送
return await this.sendUnityRequest(
`${this.commandPrefix}.${action}`,
parameters
);
}
}
リソースハンドラー (TypeScript)
BaseResourceHandler
を拡張して新しいリソースハンドラーを作成:
import { BaseResourceHandler } from "../core/BaseResourceHandler.js";
import { JObject } from "../types/index.js";
import { URL } from "url";
export class YourResourceHandler extends BaseResourceHandler {
public get resourceName(): string {
return "yourresource";
}
public get description(): string {
return "リソースの説明";
}
public get resourceUriTemplate(): string {
return "unity://yourresource";
}
protected async fetchResourceData(uri: URL, parameters?: JObject): Promise<JObject> {
// リクエストパラメータを処理
const param1 = parameters?.param1 as string;
// Unityにリクエストを送信
const response = await this.sendUnityRequest("yourresource.get", {
param1: param1
});
if (!response.success) {
throw new Error(response.error as string || "リソース取得に失敗しました");
}
// 応答データを整形して返す
return {
items: response.items || []
};
}
}
プロンプトハンドラー (TypeScript)
BasePromptHandler
を拡張して新しいプロンプトハンドラーを作成:
import { BasePromptHandler } from "../core/BasePromptHandler.js";
import { IMcpPromptDefinition } from "../core/interfaces/IPromptHandler.js";
import { z } from "zod";
export class YourPromptHandler extends BasePromptHandler {
public get promptName(): string {
return "yourprompt";
}
public get description(): string {
return "プロンプトの説明";
}
public getPromptDefinitions(): Map<string, IMcpPromptDefinition> {
const prompts = new Map<string, IMcpPromptDefinition>();
// プロンプト定義を登録
prompts.set("analyze-component", {
description: "Unityコンポーネントを分析する",
template: "以下のUnityコンポーネントを詳細に分析し、改善点を提案してください:\n\n```csharp\n{code}\n```",
additionalProperties: {
code: z.string().describe("分析対象のC#コード")
}
});
return prompts;
}
}
注意: C#側のIMcpCommandHandler
またはIMcpResourceHandler
を実装したクラスはプロジェクト内のどこに配置しても、アセンブリ検索によって自動的に検出・登録されます。TypeScript側も同様にhandlers
ディレクトリに配置することで自動検出されます。
🔄 通信フロー
- Claude (または他の AI) がMCPのいずれかの機能(ツール/リソース/プロンプト)を呼び出す
- TypeScript サーバーが TCP 経由で Unity にリクエストを転送
- Unity の McpServer がリクエストを受信し、適切なハンドラーを見つける
- ハンドラーが Unity のメインスレッドでリクエストを処理
- 結果が TCP 接続を通じて TypeScript サーバーに戻される
- TypeScript サーバーが結果をフォーマットして Claude に返す
⚙️ 設定
Unity 設定
Edit > Preferences > Unity MCP から設定にアクセス:
- Host: サーバーをバインドする IP アドレス (デフォルト: 127.0.0.1)
- Port: リッスンするポート (デフォルト: 27182)
- UDP Discovery: TypeScriptサーバーの自動検出を有効化
- Auto-start on Launch: Unity 起動時に自動的にサーバーを開始
- Auto-restart on Play Mode Change: プレイモードの開始/終了時にサーバーを再起動
- Detailed Logs: デバッグ用の詳細ログを有効化
TypeScript 設定
TypeScript サーバーの環境変数:
-
MCP_HOST
: Unity サーバーホスト (デフォルト: 127.0.0.1) -
MCP_PORT
: Unity サーバーポート (デフォルト: 27182)
🔍 トラブルシューティング
一般的な問題
-
接続エラー
- Unity側のファイアウォール設定を確認
- ポート番号が正しく設定されているか確認
- 別のプロセスが同じポートを使用していないか確認
-
ハンドラーが登録されない
- ハンドラークラスが正しいインターフェースを実装しているか確認
- C#ハンドラーがpublicまたはinternalアクセスレベルか確認
- Unity側でログを確認し登録プロセスでエラーが発生していないか確認
-
リソースが見つからない
- リソース名とURIが一致しているか確認
- リソースハンドラーが正しく有効化されているか確認
ログの確認
- Unity Console: McpServerからのログメッセージを確認
- TypeScriptサーバー: コンソール出力でMCP Inspectorなどを用いて通信エラーを確認
📚 組み込みハンドラー
Unity (C#)
- MenuItemCommandHandler: Unity エディタのメニュー項目を実行
- ConsoleCommandHandler: Unity コンソールログ操作
- AssembliesResourceHandler: アセンブリ情報の取得
- PackagesResourceHandler: パッケージ情報の取得
TypeScript
- MenuItemCommandHandler: メニュー項目実行
- ConsoleCommandHandler: コンソールログ操作
- AssemblyResourceHandler: アセンブリ情報の取得
- PackageResourceHandler: パッケージ情報の取得
📖 外部リソース
⚠️ セキュリティに関する注意
- 信頼できないハンドラーを実行しない: 第三者が作成したハンドラーコードは、事前にセキュリティレビューを行ってから使用してください。
-
コード実行権限を制限: 特に
code_execute
コマンドを含むハンドラーは任意コード実行可能なため、運用環境では無効化を検討してください。
📄 ライセンス
このプロジェクトは MIT ライセンスの下で提供されています - 詳細はライセンスファイルを参照してください。
Shiranui-Isuzu いすず
相关推荐
AI's query engine - Platform for building AI that can answer questions over large scale federated data. - The only MCP Server you'll ever need
Reviews
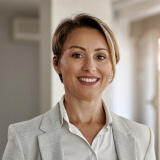
user_dwX8ROwh
UnityMCP by isuzu-shiranui is a fantastic application for managing multiple Unity projects seamlessly. Its intuitive interface and robust features make development more efficient and organized. Highly recommend it to all Unity developers looking for a reliable project management tool!
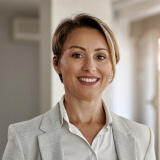
user_F3tyWbMO
UnityMCP has drastically improved my project management efficiency. It's an incredibly user-friendly platform and the seamless integration with my current workflow is a game-changer. Kudos to isuzu-shiranui for creating such a robust and intuitive tool. Highly recommend it for anyone looking to streamline their project management processes!
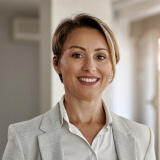
user_1bADu8HO
UnityMCP is an exceptional tool for anyone serious about managed care plans. The intuitive interface and robust set of features make overseeing complex operations seamless. Isuzu-shiranui has done an outstanding job creating a product that's both powerful and user-friendly. Highly recommended for anyone in the healthcare management field!
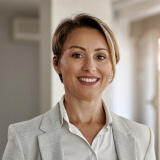
user_pP6oIWeS
UnityMCP by isuzu-shiranui is a remarkably efficient tool that has significantly enhanced my development workflow. Its user-friendly interface and seamless integration capabilities make it an indispensable asset for any project. I highly recommend UnityMCP to both novice and experienced developers looking to streamline their processes and improve productivity.
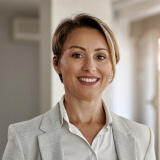
user_MyNuri98
UnityMCP is an exceptional tool created by isuzu-shiranui. It streamlines my workflow with its intuitive interface and robust features. Highly recommended for anyone looking to optimize their Unity projects.
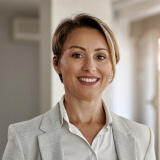
user_PtS8vli7
UnityMCP is an exceptional application developed by isuzu-shiranui that offers an intuitive and user-friendly interface. As a loyal user, I appreciate its comprehensive features and consistent performance. This tool has significantly streamlined my workflow, making complex tasks easier to manage. The seamless integration and regular updates ensure a reliable experience, making UnityMCP a must-have for anyone in need of a robust management solution. Highly recommended!
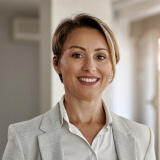
user_fIqm5mSN
As a devoted user of UnityMCP, created by isuzu-shiranui, I must say this app is a game-changer for anyone working with MCP applications. The intuitive interface and robust features make it incredibly easy to manage and enhance the performance of your projects. Highly recommend it for all developers!
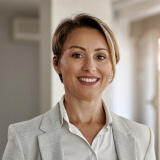
user_RzhtL35R
UnityMCP by isuzu-shiranui is a game-changer for Unity developers! The seamless integration and user-friendly interface make managing complex projects a breeze. The product's efficiency in handling multiple aspects of game development has significantly boosted my productivity and creativity. With UnityMCP, I can focus more on creating rather than managing. Highly recommend it to anyone serious about game development!
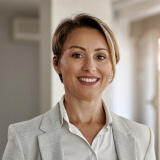
user_DPcaDLan
UnityMCP is an outstanding tool for optimizing my workflow. Developed by isuzu-shiranui, it integrates seamlessly with my projects and enhances productivity. Highly recommended for anyone in need of an efficient management tool.
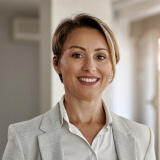
user_DC9bzHEM
As a dedicated user of UnityMCP, I must say it’s a phenomenal tool authored by isuzu-shiranui. This product has seamlessly integrated into my workflow, significantly boosting productivity and enhancing my project management capabilities. Highly recommended for anyone serious about leveraging MCP applications efficiently!
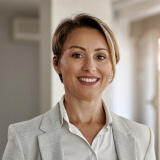
user_FNpIMzl3
UnityMCP is an incredibly efficient and user-friendly platform, created by the talented isuzu-shiranui. It excels in providing a seamless experience for managing and deploying multiple applications. The intuitive interface and powerful features make it indispensable for any developer. Highly recommended for anyone looking to streamline their workflow!
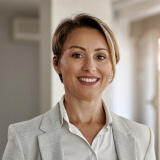
user_Mu2M5GCg
UnityMCP by isuzu-shiranui is an excellent tool for enhancing Unity projects. As a loyal MCP application user, I find it incredibly intuitive and efficient. It seamlessly integrates with various components, streamlining my workflow significantly. If you're looking to boost your Unity development process, UnityMCP is definitely worth exploring. Highly recommend!
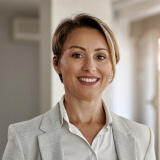
user_GNTac0tJ
UnityMCP is an exceptional tool for managing Unity development projects. Its interface is user-friendly, which allows for seamless integration and efficient workflow. Developed by isuzu-shiranui, this application significantly enhances productivity and project organization. Highly recommended for anyone serious about optimizing their Unity pipeline!