I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
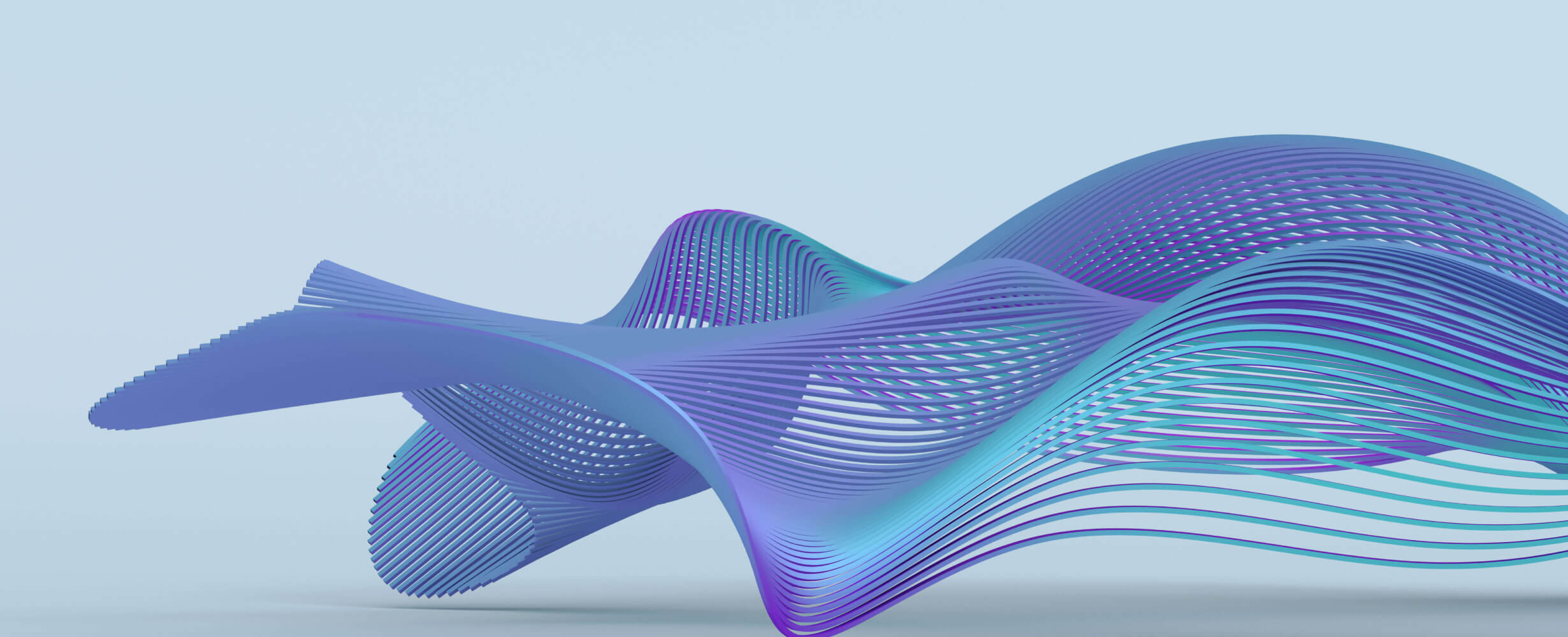
PHP-MCP服务器
基于php实现的MCP(模型控制协议)服务器框架,MCP服务,工具,提示,
2
Github Watches
2
Github Forks
9
Github Stars
PHP MCP Server
这是一个基于 PHP 实现的 MCP (Model Control Protocol) 服务器框架,支持通过注解优雅地定义 MCP 服务。
项目概述
本项目提供了一个完整的 MCP 服务器实现,特色功能:
- 基于注解的 MCP 服务定义
- 支持 Tool、Prompt、Resource 三种处理器
- 支持 Stdio、Sse 两种传输方式
- 支持 Swow 和 Swoole 两种环境
- 完整的日志系统
- Docker 支持
系统要求
- PHP >= 8.1
- Composer
- Swow 扩展 > 1.5 或 Swoole > 5.1
- Docker (可选)
快速开始
安装
# 1. 克隆项目
git clone https://github.com/he426100/php-mcp-server
cd php-mcp-server
# 2. 安装依赖
composer install
# 3. 可选,安装 Swow 扩展(如果没有)
./vendor/bin/swow-builder --install
关于 Swow 扩展的详细安装说明,请参考 Swow 官方文档
运行示例服务器
php bin/console mcp:test-server
通用命令参数
Parameter | Description | Default Value | Options |
---|---|---|---|
--transport | Transport type | stdio | stdio, sse |
--port | Port to listen on for SSE | 8000 |
注解使用指南
本框架提供三种核心注解用于定义 MCP 服务:
1. Tool 注解
用于定义工具类处理器:
use Mcp\Annotation\Tool;
class MyService {
#[Tool(
name: 'calculate-sum',
description: '计算两个数的和',
parameters: [
'num1' => [
'type' => 'number',
'description' => '第一个数字',
'required' => true
],
'num2' => [
'type' => 'number',
'description' => '第二个数字',
'required' => true
]
]
)]
public function sum(int $num1, int $num2): int
{
return $num1 + $num2;
}
}
2. Prompt 注解
用于定义提示模板处理器:
use Mcp\Annotation\Prompt;
class MyService {
#[Prompt(
name: 'greeting',
description: '生成问候语',
arguments: [
'name' => [
'description' => '要问候的人名',
'required' => true
]
]
)]
public function greeting(string $name): string
{
return "Hello, {$name}!";
}
}
3. Resource 注解
用于定义资源处理器:
use Mcp\Annotation\Resource;
class MyService {
#[Resource(
uri: 'example://greeting',
name: 'Greeting Text',
description: '问候语资源',
mimeType: 'text/plain'
)]
public function getGreeting(): string
{
return "Hello from MCP server!";
}
}
创建自定义服务
- 创建服务类:
namespace Your\Namespace;
use Mcp\Annotation\Tool;
use Mcp\Annotation\Prompt;
use Mcp\Annotation\Resource;
class CustomService
{
#[Tool(name: 'custom-tool', description: '自定义工具')]
public function customTool(): string
{
return "Custom tool result";
}
}
- 创建命令类:
namespace Your\Namespace\Command;
use He426100\McpServer\Command\AbstractMcpServerCommand;
use Your\Namespace\CustomService;
class CustomServerCommand extends AbstractMcpServerCommand
{
protected string $serverName = 'custom-server';
protected string $serviceClass = CustomService::class;
protected function configure(): void
{
parent::configure();
$this->setName('custom:server')
->setDescription('运行自定义 MCP 服务器');
}
}
注解参数说明
Tool 注解参数
参数 | 类型 | 说明 | 必填 |
---|---|---|---|
name | string | 工具名称 | 是 |
description | string | 工具描述 | 是 |
parameters | array | 参数定义 | 否 |
Prompt 注解参数
参数 | 类型 | 说明 | 必填 |
---|---|---|---|
name | string | 提示模板名称 | 是 |
description | string | 提示模板描述 | 是 |
arguments | array | 参数定义 | 否 |
Resource 注解参数
参数 | 类型 | 说明 | 必填 |
---|---|---|---|
uri | string | 资源URI | 是 |
name | string | 资源名称 | 是 |
description | string | 资源描述 | 是 |
mimeType | string | MIME类型 | 否 |
注解函数返回类型说明
Tool 注解函数支持的返回类型
返回类型 | 说明 | 转换结果 |
---|---|---|
TextContent/ImageContent/EmbeddedResource | 直接返回内容对象 | 原样保留 |
TextContent/ImageContent/EmbeddedResource 数组 | 内容对象数组 | 原样保留 |
ResourceContents | 资源内容对象 | 转换为 EmbeddedResource |
字符串或标量类型 | 如 string、int、float、bool | 转换为 TextContent |
null | 空值 | 转换为空字符串的 TextContent |
数组或对象 | 复杂数据结构 | 转换为 JSON 格式的 TextContent |
Prompt 注解函数支持的返回类型
返回类型 | 说明 | 转换结果 |
---|---|---|
PromptMessage | 消息对象 | 原样保留 |
PromptMessage 数组 | 消息对象数组 | 原样保留 |
Content 对象 | TextContent/ImageContent 等 | 转换为用户角色的 PromptMessage |
字符串或标量类型 | 如 string、int、float、bool | 转换为带 TextContent 的用户消息 |
null | 空值 | 转换为空内容的用户消息 |
数组或对象 | 复杂数据结构 | 转换为 JSON 格式的用户消息 |
Resource 注解函数支持的返回类型
返回类型 | 说明 | 转换结果 |
---|---|---|
TextResourceContents/BlobResourceContents | 资源内容对象 | 原样保留 |
ResourceContents 数组 | 资源内容对象数组 | 原样保留 |
字符串或可转字符串对象 | 文本内容 | 根据 MIME 类型转换为对应资源内容 |
null | 空值 | 转换为空的 TextResourceContents |
数组或对象 | 复杂数据结构 | 转换为 JSON 格式的资源内容 |
注意事项:
- 对于超过 2MB 的大文件内容会自动截断
- 文本类型 (text/*) 的 MIME 类型会使用 TextResourceContents
- 其他 MIME 类型会使用 BlobResourceContents
日志配置
服务器日志默认保存在 runtime/server_log.txt
,可通过继承 AbstractMcpServerCommand
修改:
protected string $logFilePath = '/custom/path/to/log.txt';
Docker 支持
构建并运行容器:
docker build -t php-mcp-server .
docker run -i --rm php-mcp-server
许可证
贡献
欢迎提交 Issue 和 Pull Request。
作者
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
Take an adjectivised noun, and create images making it progressively more adjective!
Reviews
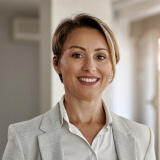
user_VicejDIO
Nmap MCP Server by imjdl is an exceptional tool for scanning and managing network vulnerabilities seamlessly. The interface is intuitive and user-friendly, making it easier even for beginners to navigate. Its robust functionality ensures comprehensive security assessments. Highly recommend! Check it out at: https://mcp.so/server/nmap-mcpserver/imjdl.