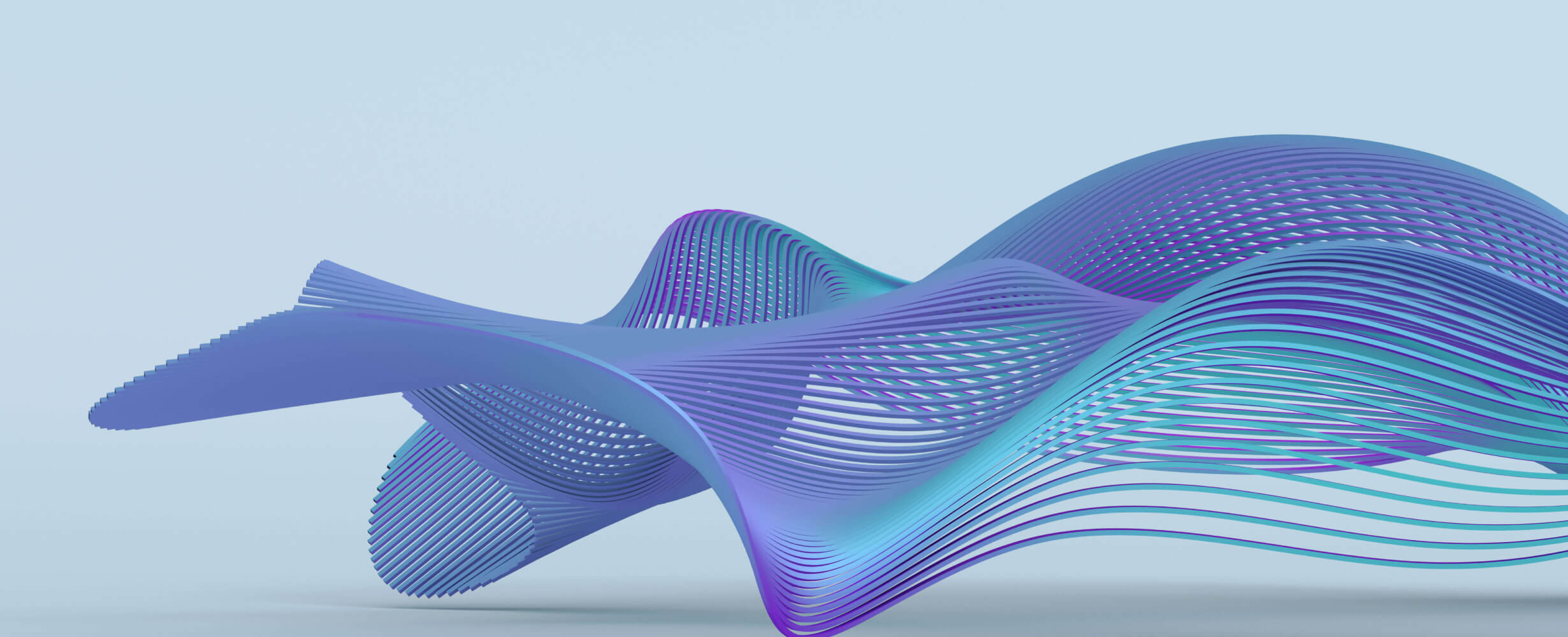
Supabase MCP Server
A Model Context Protocol (MCP) server that provides comprehensive tools for interacting with Supabase databases, storage, and edge functions. This server enables seamless integration between Supabase services and MCP-compatible applications.
Overview
The Supabase MCP server acts as a bridge between MCP clients and Supabase's suite of services, providing:
- Database operations with rich querying capabilities
- Storage management for files and assets
- Edge function invocation
- Project and organization management
- User authentication and management
- Role-based access control
Architecture
The server is built using TypeScript and follows a modular architecture:
supabase-server/
├── src/
│ ├── index.ts # Main server implementation
│ └── types/
│ └── supabase.d.ts # Type definitions
├── package.json
├── tsconfig.json
├── config.json.example # Example configuration file
└── .env.example # Environment variables template
Key Components
- Server Class: Implements the MCP server interface and handles all client requests
- Type Definitions: Comprehensive TypeScript definitions for all operations
- Environment Configuration: Secure configuration management via environment variables
- Error Handling: Robust error handling with detailed error messages
Prerequisites
- Node.js 16.x or higher
- A Supabase project with:
- Project URL
- Service Role Key (for admin operations)
- Access Token (for management operations)
- MCP-compatible client
Installation
Installing via Smithery
To install Supabase Server for Claude Desktop automatically via Smithery:
npx -y @smithery/cli install supabase-server --client claude
- Clone the repository:
git clone https://github.com/DynamicEndpoints/supabase-mcp.git
cd supabase-mcp
- Install dependencies:
npm install
- Create environment configuration:
cp .env.example .env
- Configure environment variables:
SUPABASE_URL=your_project_url_here
SUPABASE_KEY=your_service_role_key_here
SUPABASE_ACCESS_TOKEN=your_access_token_here # Required for management operations
- Create server configuration:
cp config.json.example config.json
- Build the server:
npm run build
Configuration
The server supports extensive configuration through both environment variables and a config.json file. Here's a detailed breakdown of the configuration options:
Server Configuration
{
"server": {
"name": "supabase-server", // Server name
"version": "0.1.0", // Server version
"port": 3000, // Port number (if running standalone)
"host": "localhost" // Host address (if running standalone)
}
}
Supabase Configuration
{
"supabase": {
"project": {
"url": "your_project_url",
"key": "your_service_role_key",
"accessToken": "your_access_token"
},
"storage": {
"defaultBucket": "public", // Default storage bucket
"maxFileSize": 52428800, // Max file size in bytes (50MB)
"allowedMimeTypes": [ // Allowed file types
"image/*",
"application/pdf",
"text/*"
]
},
"database": {
"maxConnections": 10, // Max DB connections
"timeout": 30000, // Query timeout in ms
"ssl": true // SSL connection
},
"auth": {
"autoConfirmUsers": false, // Auto-confirm new users
"disableSignup": false, // Disable public signups
"jwt": {
"expiresIn": "1h", // Token expiration
"algorithm": "HS256" // JWT algorithm
}
}
}
}
Logging Configuration
{
"logging": {
"level": "info", // Log level
"format": "json", // Log format
"outputs": ["console", "file"], // Output destinations
"file": {
"path": "logs/server.log", // Log file path
"maxSize": "10m", // Max file size
"maxFiles": 5 // Max number of files
}
}
}
Security Configuration
{
"security": {
"cors": {
"enabled": true,
"origins": ["*"],
"methods": ["GET", "POST", "PUT", "DELETE", "OPTIONS"],
"allowedHeaders": ["Content-Type", "Authorization"]
},
"rateLimit": {
"enabled": true,
"windowMs": 900000, // 15 minutes
"max": 100 // Max requests per window
}
}
}
Monitoring Configuration
{
"monitoring": {
"enabled": true,
"metrics": {
"collect": true,
"interval": 60000 // Collection interval in ms
},
"health": {
"enabled": true,
"path": "/health" // Health check endpoint
}
}
}
See config.json.example
for a complete example configuration file.
MCP Integration
Add the server to your MCP settings (cline_mcp_settings.json):
{
"mcpServers": {
"supabase": {
"command": "node",
"args": ["path/to/supabase-server/build/index.js"],
"env": {
"SUPABASE_URL": "your_project_url",
"SUPABASE_KEY": "your_service_role_key",
"SUPABASE_ACCESS_TOKEN": "your_access_token"
},
"config": "path/to/config.json" // Optional: path to configuration file
}
}
}
Available Tools
Database Operations
create_record
Create a new record in a table with support for returning specific fields.
{
table: string;
data: Record<string, any>;
returning?: string[];
}
Example:
{
table: "users",
data: {
name: "John Doe",
email: "john@example.com"
},
returning: ["id", "created_at"]
}
read_records
Read records with advanced filtering, joins, and field selection.
{
table: string;
select?: string[];
filter?: Record<string, any>;
joins?: Array<{
type?: 'inner' | 'left' | 'right' | 'full';
table: string;
on: string;
}>;
}
Example:
{
table: "posts",
select: ["id", "title", "user.name"],
filter: { published: true },
joins: [{
type: "left",
table: "users",
on: "posts.user_id=users.id"
}]
}
update_record
Update records with filtering and returning capabilities.
{
table: string;
data: Record<string, any>;
filter?: Record<string, any>;
returning?: string[];
}
Example:
{
table: "users",
data: { status: "active" },
filter: { email: "john@example.com" },
returning: ["id", "status", "updated_at"]
}
delete_record
Delete records with filtering and returning capabilities.
{
table: string;
filter?: Record<string, any>;
returning?: string[];
}
Example:
{
table: "posts",
filter: { status: "draft" },
returning: ["id", "title"]
}
Storage Operations
upload_file
Upload files to Supabase Storage with configurable options.
{
bucket: string;
path: string;
file: File | Blob;
options?: {
cacheControl?: string;
contentType?: string;
upsert?: boolean;
};
}
Example:
{
bucket: "avatars",
path: "users/123/profile.jpg",
file: imageBlob,
options: {
contentType: "image/jpeg",
upsert: true
}
}
download_file
Download files from Supabase Storage.
{
bucket: string;
path: string;
}
Example:
{
bucket: "documents",
path: "reports/annual-2023.pdf"
}
Edge Functions
invoke_function
Invoke Supabase Edge Functions with parameters and custom options.
{
function: string;
params?: Record<string, any>;
options?: {
headers?: Record<string, string>;
responseType?: 'json' | 'text' | 'arraybuffer';
};
}
Example:
{
function: "process-image",
params: {
url: "https://example.com/image.jpg",
width: 800
},
options: {
responseType: "json"
}
}
User Management
list_users
List users with pagination support.
{
page?: number;
per_page?: number;
}
create_user
Create a new user with metadata.
{
email: string;
password: string;
data?: Record<string, any>;
}
update_user
Update user details.
{
user_id: string;
email?: string;
password?: string;
data?: Record<string, any>;
}
delete_user
Delete a user.
{
user_id: string;
}
assign_user_role
Assign a role to a user.
{
user_id: string;
role: string;
}
remove_user_role
Remove a role from a user.
{
user_id: string;
role: string;
}
Error Handling
The server provides detailed error messages for common scenarios:
- Invalid parameters
- Authentication failures
- Permission issues
- Rate limiting
- Network errors
- Database constraints
Errors are returned in a standardized format:
{
code: ErrorCode;
message: string;
details?: any;
}
Development
Running Tests
npm test
Building
npm run build
Linting
npm run lint
Contributing
- Fork the repository
- Create a feature branch
- Commit your changes
- Push to the branch
- Create a Pull Request
License
MIT License - see LICENSE for details
Support
For support, please:
- Check the issues for existing problems/solutions
- Create a new issue with detailed reproduction steps
- Include relevant error messages and environment details
相关推荐
Confidential guide on numerology and astrology, based of GG33 Public information
Take an adjectivised noun, and create images making it progressively more adjective!
Siri Shortcut Finder – your go-to place for discovering amazing Siri Shortcuts with ease
Reviews
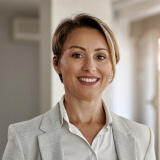
user_ODFn9gFr
Supabase-mcp by DynamicEndpoints is a game-changer for anyone using Multi-Cloud Platform solutions. The repository on GitHub is well-documented and the setup is straightforward, providing a seamless integration experience. The support from DynamicEndpoints has been phenomenal. Highly recommended for developers looking to enhance their projects with robust database solutions!