I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
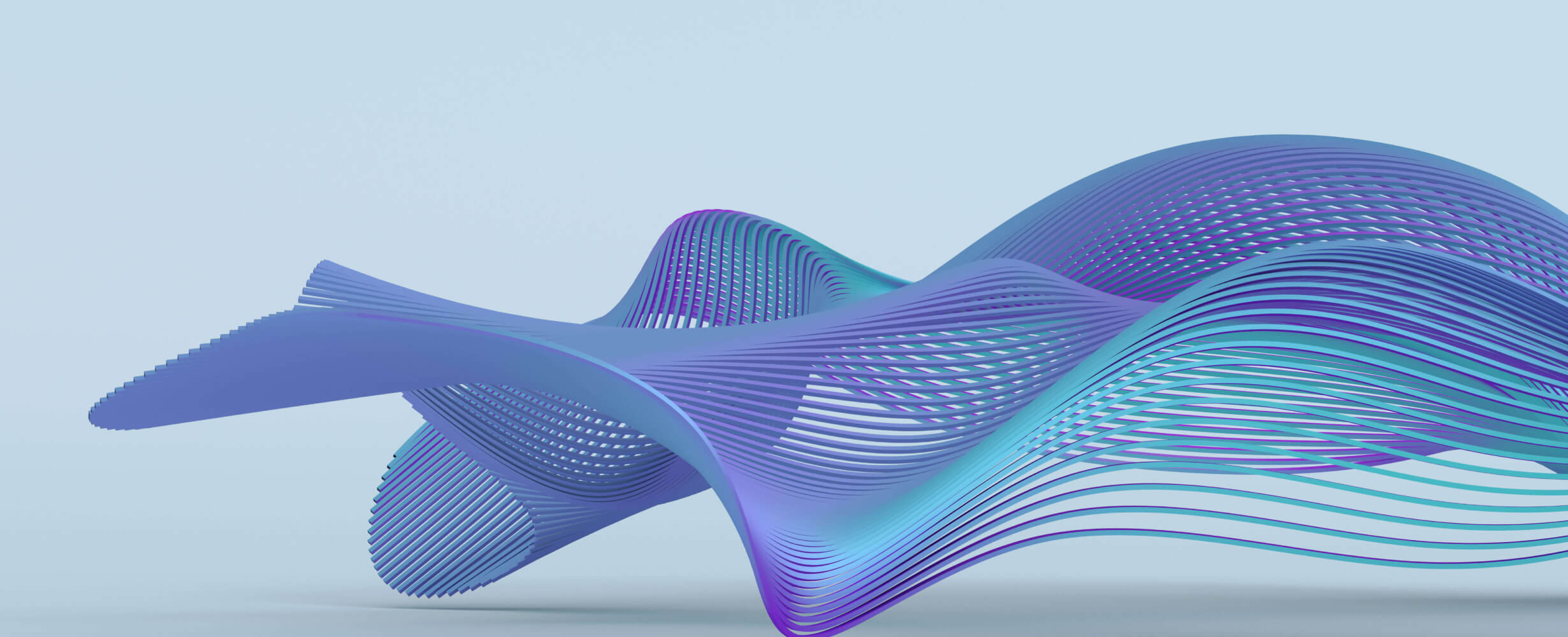
MCP检验客户
MCP测试客户端是模型上下文协议(MCP)服务器的打字稿测试实用程序。
3
Github Watches
1
Github Forks
9
Github Stars
MCP Test Client
A testing utility for Model Context Protocol (MCP) servers. This client helps you test MCP server implementations by providing a simple interface for making tool calls and validating responses.
Features
- Easy-to-use testing interface for MCP servers
- Built-in support for tool listing and tool calls
- Type-safe implementation using TypeScript
- Assertion utilities for validating server responses
- Mock calculator server implementation for examples
Installation
bun install mcp-test-client
Usage
Basic Example
import { MCPTestClient } from 'mcp-test-client';
describe('MCP Server Tests', () => {
let client: MCPTestClient;
beforeAll(async () => {
client = new MCPTestClient({
serverCommand: 'bun',
serverArgs: ['./path/to/your/server.ts'],
});
await client.init();
});
afterAll(async () => {
await client.cleanup();
});
test('should list available tools', async () => {
const tools = await client.listTools();
expect(tools).toContainEqual(
expect.objectContaining({
name: 'your-tool-name',
description: 'Your tool description',
})
);
});
test('should call a tool', async () => {
await client.assertToolCall(
'your-tool-name',
{ arg1: 'value1', arg2: 'value2' },
(result) => {
expect(result.content[0].text).toBe('expected result');
}
);
});
});
Calculator Server Example
The package includes a mock calculator server for testing and learning purposes:
import { MCPTestClient } from 'mcp-test-client';
describe('Calculator Server Tests', () => {
let client: MCPTestClient;
beforeAll(async () => {
client = new MCPTestClient({
serverCommand: 'bun',
serverArgs: ['./tests/mocks/calculator.ts'],
});
await client.init();
});
afterAll(async () => {
await client.cleanup();
});
test('should perform addition', async () => {
await client.assertToolCall(
'calculate',
{ operation: 'add', a: 5, b: 3 },
(result) => {
expect(result.content[0].text).toBe('8');
}
);
});
});
API Reference
MCPTestClient
Constructor
constructor(config: { serverCommand: string; serverArgs: string[] })
Methods
-
init(): Promise<void>
- Initialize the client and connect to the server -
listTools(): Promise<Tool[]>
- Get a list of available tools from the server -
callTool(toolName: string, args: Record<string, unknown>): Promise<ToolResult>
- Call a specific tool -
assertToolCall(toolName: string, args: Record<string, unknown>, assertion: (result: ToolResult) => void | Promise<void>): Promise<void>
- Call a tool and run assertions on the result -
cleanup(): Promise<void>
- Clean up resources and disconnect from the server
Development
Prerequisites
- Bun (v1.0.0 or higher)
Setup
- Clone the repository
git clone <repository-url>
cd mcp-test-client
- Install dependencies
bun install
- Run tests
bun test
License
MIT
Contributing
- Fork the repository
- Create your feature branch (
git checkout -b feature/amazing-feature
) - Commit your changes (
git commit -m 'Add some amazing feature'
) - Push to the branch (
git push origin feature/amazing-feature
) - Open a Pull Request
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
This GPT assists in finding a top-rated business CPA - local or virtual. We account for their qualifications, experience, testimonials and reviews. Business operators provide a short description of your business, services wanted, and city or state.
Confidential guide on numerology and astrology, based of GG33 Public information
Take an adjectivised noun, and create images making it progressively more adjective!
Reviews
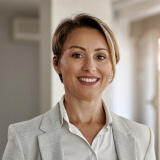
user_zaogvRkv
The Stay AI API MCP Server by mattcoatsworth is a game changer for developers looking to integrate AI capabilities seamlessly. It’s incredibly user-friendly, robust, and handles large data sets efficiently. I highly recommend it for anyone in need of a reliable server for AI projects. Check it out!