I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
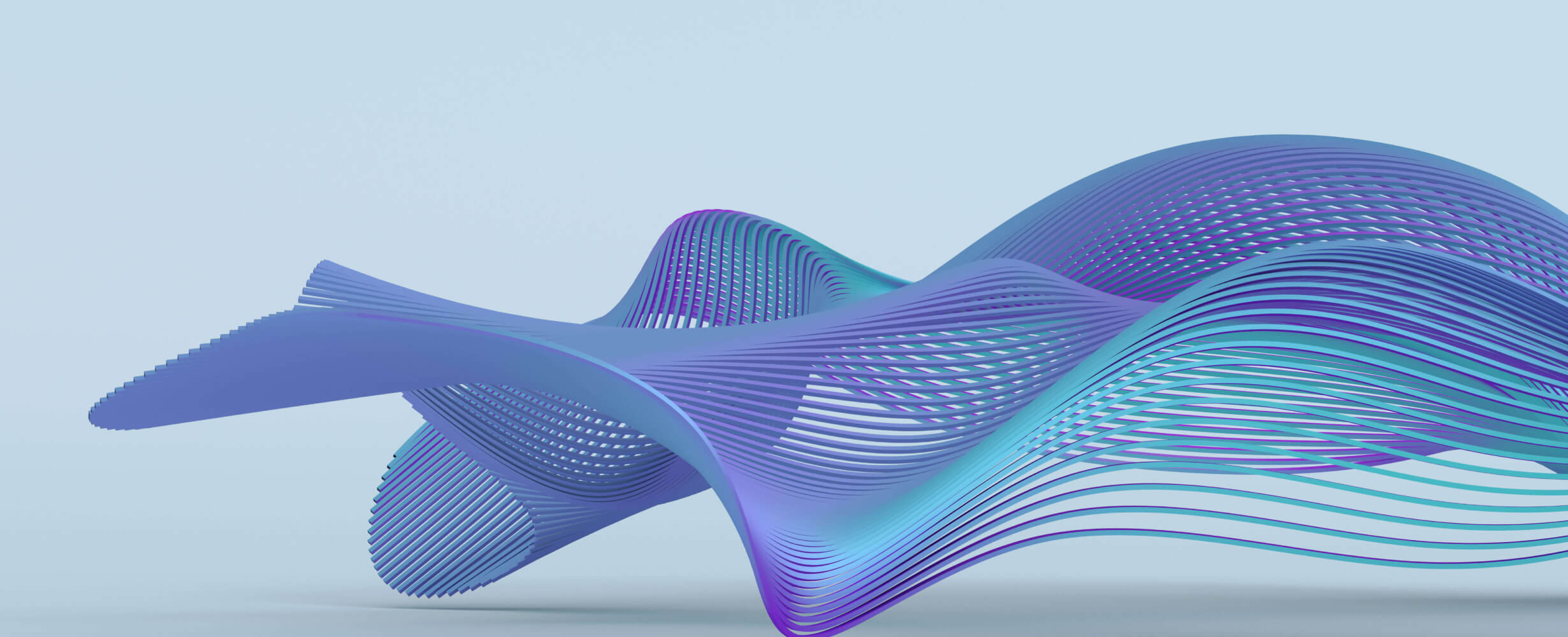
MCP-TS-Simple-Template
用于构建模型上下文协议(MCP)服务器的轻巧,可用的打字机模板。该模板提供了必不可少的脚手架来创建可以与大型语言模型无缝集成的自定义AI工具。
1
Github Watches
2
Github Forks
3
Github Stars
MCP TypeScript Simple Template
A simple TypeScript template for building Model Context Protocol (MCP) servers. This project provides a foundation for creating custom MCP tools that can be integrated with AI systems.
Overview
This template implements a basic MCP server with a sample BMI calculator tool. It demonstrates how to:
- Set up an MCP server in TypeScript
- Define and implement MCP tools with input validation using Zod
- Connect the server to standard I/O for communication
Prerequisites
- Node.js (v20 or higher recommended)
- npm or yarn
Installation
- Clone this repository
- Install dependencies:
npm install
Project Structure
-
index.ts
- Main server implementation with sample tool -
package.json
- Project dependencies and scripts -
tsconfig.json
- TypeScript configuration
Usage
Building and Running
Build and start the server:
npm start
This will compile the TypeScript code and start the MCP server.
Development
For development, you can:
- Modify
index.ts
to add your own tools - Run the build command to compile:
npm run build
Creating Custom Tools
To create a new tool, follow this pattern in index.ts
:
server.tool(
"your-tool-name",
{
// Define input schema using Zod
paramName: z.string(),
// Add more parameters as needed
},
async ({ paramName }) => ({
content: [{
type: "text",
text: "Your tool's response"
}]
})
);
Dependencies
-
@modelcontextprotocol/sdk
- Core MCP SDK -
zod
- Schema validation -
dotenv
- Environment variable management -
typescript
- TypeScript compiler
License
ISC
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
Take an adjectivised noun, and create images making it progressively more adjective!
Reviews
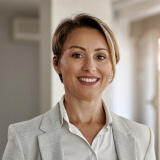
user_83dhyPBh
I recently started using the mcp-ts-simple-template by ChenReuven and I'm thoroughly impressed! The template is straightforward and easy to use, making TypeScript project setup a breeze. The comprehensive welcome information ensures a smooth start, and the provided starting URL is incredibly helpful for swift project initialization. A must-have for any TypeScript developer looking for simplicity and efficiency. Highly recommended!