I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
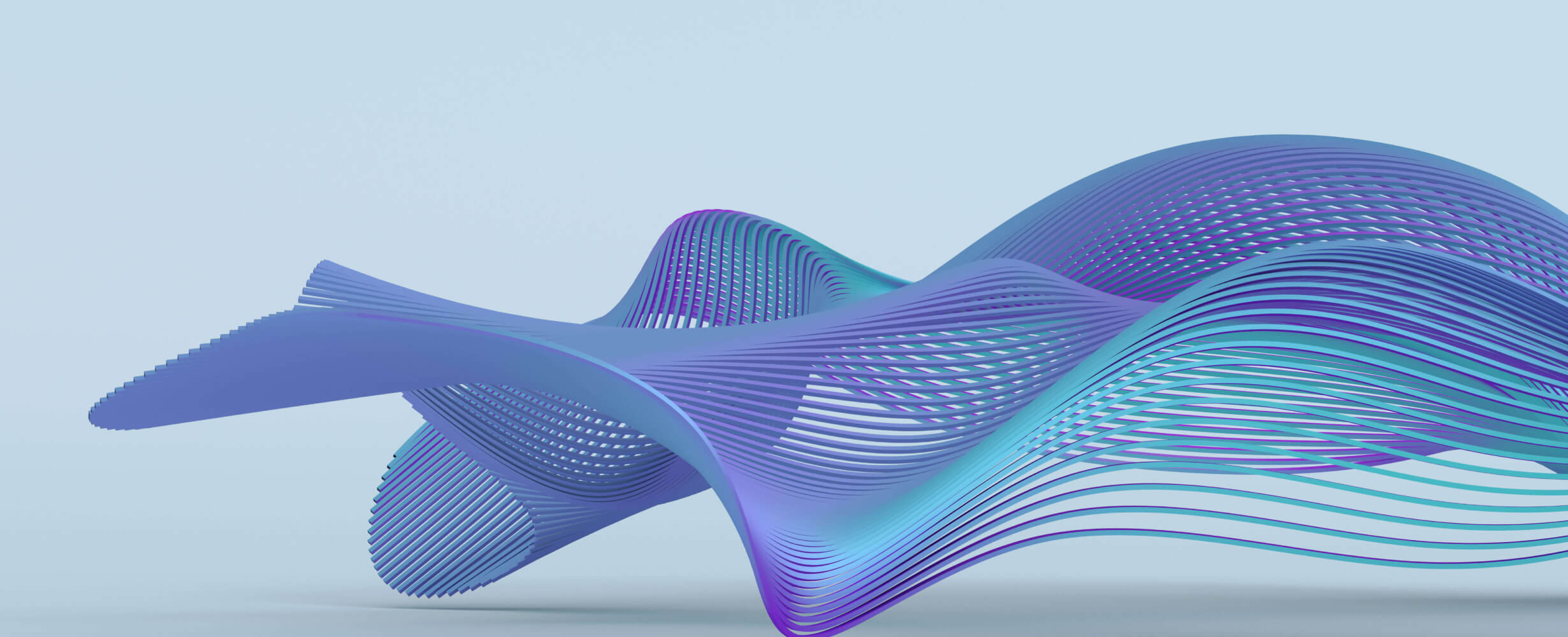
Mini-Blockchain
A simple blockchain implementation in Rust featuring SHA-256 hashing, CLI interface, and MCP server integration. Demonstrates core blockchain concepts through block creation and chain validation while exposing blockchain functionalities over a network.
3 years
Works with Finder
1
Github Watches
1
Github Forks
0
Github Stars
Blockchain CLI in Rust
This repository contains a simple blockchain demonstration implemented in Rust. The project illustrates core Rust principles through a minimal command-line application and integrates an MCP server to expose blockchain functionalities over a network.
Overview
The application implements a basic blockchain where each block includes:
- Index: The block's position in the chain.
- Timestamp: The creation time in ISO 8601 format.
- Data: User-supplied content.
- Previous Hash: The SHA-256 hash of the previous block.
- Current Hash: The SHA-256 hash computed from the block's contents.
A menu-driven CLI lets you:
- Add a new block.
- Print the current blockchain.
- Validate the blockchain's integrity.
Technologies
- Rust: A systems programming language focused on safety and performance.
- sha2: For computing SHA-256 hashes.
- chrono: For accurate timestamping using UTC time.
- serde & serde_json: For JSON serialization (used in MCP communication).
Getting Started
Prerequisites
Build and Run
To create and run the project using Cargo, execute in your terminal:
cargo new blockchain_app
cd blockchain_app && cargo run
Note: If you cloned this repository, simply navigate into the project folder and run cargo run
.
Usage
When you run the application, the CLI displays the following options:
- Add Block: Input data to create a new block.
- Print Blockchain: Display all blocks in the blockchain.
- Validate Blockchain: Verify that each block is properly linked.
- Exit: Close the application.
MCP Server Integration
The project includes an MCP server that runs concurrently with the CLI. It listens on 127.0.0.1:7878
and allows external clients to interact with the blockchain using JSON commands.
Available MCP Commands
-
latest_block: Retrieves the latest block in the blockchain.
-
Example:
echo '{"command": "latest_block"}' | nc 127.0.0.1 7878
-
Sample Output:
{"status":"ok","message":"Latest block retrieved","block":{"index":0,"timestamp":"2025-02-26T10:06:59.931506+00:00","data":"Genesis Block","previous_hash":"0","hash":"f465878acf7be88a124fd7ebc9a3640793612023828d9af8ad237eefd4a1a22e"}}
-
Example:
-
add_block: Adds a new block with the provided data.
-
Example:
echo '{"command": "add_block", "data": "sample transaction"}' | nc 127.0.0.1 7878
-
Sample Output:
{"status":"ok","message":"Block added","block":null}
-
Example:
Testing the MCP Server
-
Run the Application:
Open a terminal, navigate to the project directory (blockchain_app
), and run:cargo run
You will see the CLI menu and a message indicating that the MCP server is listening on
127.0.0.1:7878
. -
Query from a Separate Terminal:
Open a new terminal window and test the MCP commands using netcat (nc
). For example:- To retrieve the latest block:
echo '{"command": "latest_block"}' | nc 127.0.0.1 7878
- To add a new block:
echo '{"command": "add_block", "data": "sample transaction"}' | nc 127.0.0.1 7878
- To retrieve the latest block:
Note: The MCP server runs in a separate thread alongside the CLI, so ensure you use a different terminal window for these tests.
Summary
This project demonstrates how to combine blockchain logic with an externally accessible MCP server in Rust. It serves as a practical example for networked tool integration.
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
A geek-themed horoscope generator blending Bitcoin prices, tech jargon, and astrological whimsy.
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
Mirror ofhttps://github.com/agentience/practices_mcp_server
Mirror ofhttps://github.com/bitrefill/bitrefill-mcp-server
Reviews
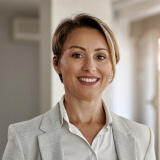
user_bweNrX59
Mini-Blockchain by FaustoS88 is an outstanding blockchain implementation for educational purposes. It simplifies complex blockchain concepts and is perfect for those looking to grasp the basics. The detailed documentation and well-structured codebase make it accessible even to beginners. Highly recommend checking it out on GitHub!