Create and Publish Business Websites in seconds. AI will gather all the details about your website and generate link to your website.
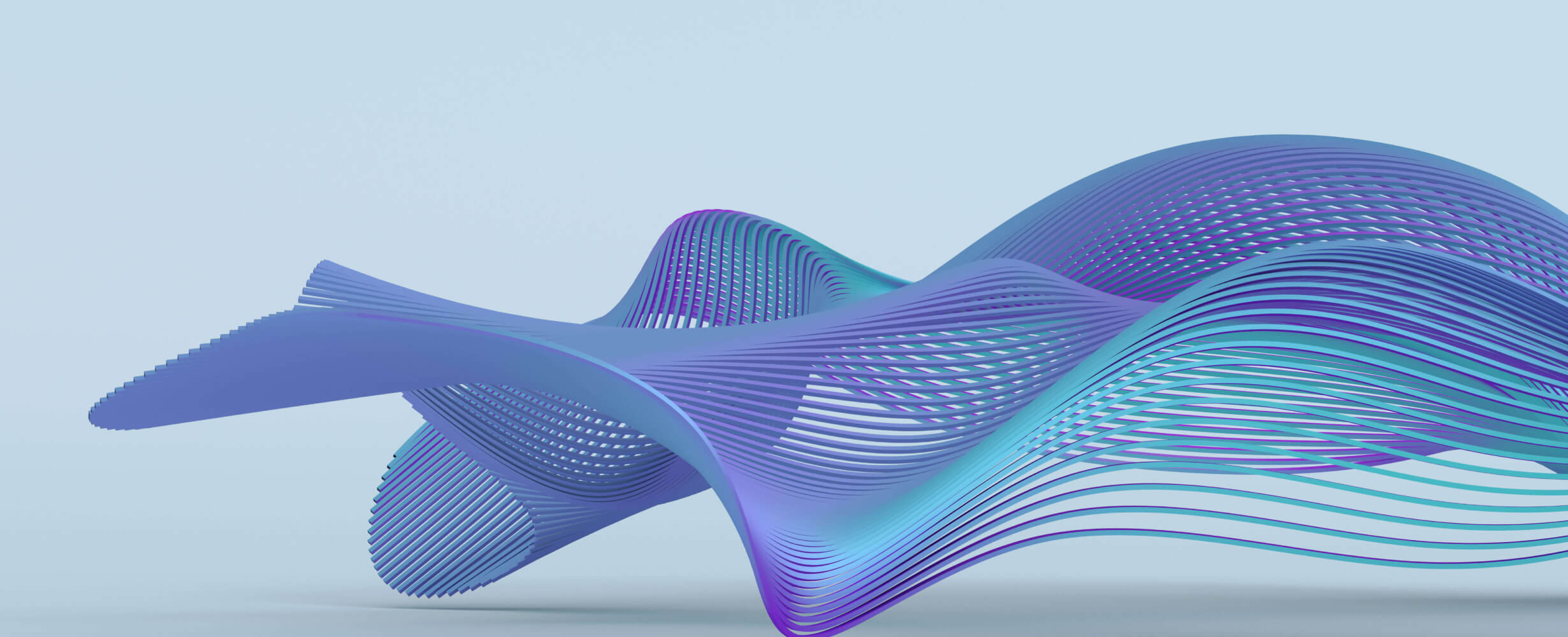
MCP AZD Template 
An MCP server that provides tools for working with Azure Developer CLI (azd) templates. This package helps with template validation, analysis, and creation while following best practices.
Installation
Global Installation
To use as a command-line tool:
npm install -g mcp-azd-template
Local Installation
For use in your project:
npm install mcp-azd-template
Usage
As a CLI Tool
After global installation, you can start the MCP server by running:
mcp-azd-template
This runs the server in stdio mode, which integrates with VS Code MCP extensions.
As an MCP Server in VS Code
Add to your VS Code settings.json:
"mcp": {
"servers": {
"azd-template-helper": {
"command": "mcp-azd-template"
}
}
}
Using with npx (No Installation Required)
You can use the package directly with npx without installing it:
"mcp": {
"servers": {
"azd-template-helper": {
"command": "npx",
"args": [
"-y",
"mcp-azd-template@latest"
]
}
}
}
Programmatic Usage
You can use the API programmatically in your JavaScript/TypeScript applications:
import { createServer, listTemplates, analyzeTemplate, validateTemplate } from 'mcp-azd-template';
import { McpServer } from '@modelcontextprotocol/sdk/server/mcp.js';
// Use individual functions directly
const templates = await listTemplates();
console.log(templates);
// Or create and use the MCP server
const server = createServer();
// Connect to your preferred transport...
// Or register tools on your existing MCP server
import { registerTools } from 'mcp-azd-template';
const myServer = new McpServer({ /* your config */ });
registerTools(myServer);
Example Prompts
When using this MCP server with AI assistants like GitHub Copilot, you can use the following example prompts:
Template Search
Search for Java Spring Boot templates in the Azure AI gallery
GitHub Actions Integration
You can integrate MCP AZD Template with GitHub Actions to automatically validate your Azure Developer CLI (azd) templates on pull requests or commits. This helps ensure your templates always comply with best practices before they're merged.
Setting up the GitHub Action
- Create a
.github/workflows
directory in your repository if it doesn't already exist - Add a new file named
validate-template.yml
with the following content:
name: Validate Azure Developer CLI Template
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
workflow_dispatch:
jobs:
validate:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
with:
fetch-depth: 0
- name: Setup Node.js
uses: actions/setup-node@v3
with:
node-version: '18'
cache: 'npm'
- name: Install Azure Developer CLI
run: |
curl -fsSL https://aka.ms/install-azd.sh | bash
- name: Install mcp-azd-template
run: npm install -g mcp-azd-template
- name: Validate AZD Template
run: mcp-azd-template validate-action "${{ github.workspace }}"
Advanced Configuration
Creating Detailed Issues Automatically
The workflow includes automatic creation of GitHub issues with detailed validation results when validation fails. The created issue will contain:
- Critical issues found in the template
- Warnings and recommendations for improvement
- The full validation output in a collapsible section
- Links to the specific commit and workflow run
This feature makes it easy for teams to track and address template issues without needing to dig through workflow logs:
# This step captures the validation output
- name: Run Validation and Capture Output
id: validate
run: |
# Run validation and capture output to a file
mcp-azd-template validate-action "${{ github.workspace }}" > validation_output.txt 2>&1
# Check if the validation failed and create a summary file for the issue
if [ $? -ne 0 ]; then
echo "::set-output name=status::failed"
cat validation_output.txt
else
echo "::set-output name=status::success"
fi
continue-on-error: true
# This step creates the detailed issue with validation results
- name: Create Issue with Validation Results
if: ${{ steps.validate.outputs.status == 'failed' && github.event_name == 'push' }}
uses: actions/github-script@v6
with:
github-token: ${{ secrets.GITHUB_TOKEN }}
script: |
const fs = require('fs');
// Read validation output file
let validationOutput = fs.readFileSync('validation_output.txt', 'utf8');
// Extract critical issues and warnings sections
const criticalIssuesMatch = validationOutput.match(/### ❌ Critical Issues\\n([\\s\\S]*?)(?=\\n###|$)/);
const warningsMatch = validationOutput.match(/### ⚠️ Warnings and Recommendations\\n([\\s\\S]*?)(?=\\n###|$)/);
// Format the issue body with validation results
await github.rest.issues.create({
owner: context.repo.owner,
repo: context.repo.repo,
title: 'AZD Template Validation Failed',
body: `# Template Validation Failed\\n\\nDetails of issues found in commit ${context.sha.substring(0, 7)}...`
});
CLI Usage for CI/CD
You can also run template validation directly in any CI/CD environment:
# Install globally
npm install -g mcp-azd-template
# Run validation (exits with code 1 if validation fails)
mcp-azd-template validate-action /path/to/template
Sample Prompts
Find Next.js starter templates from both the AI gallery and azd CLI
Look for container-based microservices templates with Go support
Search for Azure Functions templates with Python and OpenAI integration
Find templates that use Azure Container Apps and Kubernetes
Search for templates with CI/CD pipeline examples
Template Analysis
Can you analyze my current Azure Developer CLI template and provide feedback?
Review this azd template in my current directory and tell me what needs improvement.
Template Validation
Validate this azd template against best practices.
Check if my azd template follows Microsoft's recommended structure and security practices.
Template Creation
Create a new Azure Function app template using TypeScript.
I need a starter template for a containerized web app using Python. Can you create one?
Help me scaffold an azd template for a .NET API with all the required files.
Template Listing
Show me available azd templates I can use as references.
What are the official Azure Developer CLI templates available?
Troubleshooting
My azd template is missing documentation. What specific sections should I add?
How do I fix the security warnings in my template's validation report?
Features
The package provides the following tools:
1. Search Templates
Search Azure Developer CLI (azd) templates and Azure AI gallery:
- Search local azd CLI templates
- Search Azure AI gallery templates
- Filter by language, architecture, or keywords
- Get detailed template information
2. List Templates
Lists all available Azure Developer CLI (azd) templates.
3. Analyze Template
Analyzes an Azure Developer CLI (azd) template directory and provides insights:
- Structure validation
- Configuration analysis
- Best practice recommendations
4. Validate Template
Performs a comprehensive validation of an azd template with detailed checks for:
- Documentation completeness
- Infrastructure configuration
- Security settings
- Development environment setup
- GitHub workflow configuration
5. Create Template
Creates a new Azure Developer CLI template with best practices built-in:
- Supports multiple languages (TypeScript, Python, Java, .NET)
- Various architectures (web, API, function app, container)
- Includes necessary configuration files
Azure YAML Validation
Validates an azure.yaml file against the known schema:
- Checks for required fields and correct structure
- Validates service configurations
- Provides best practice recommendations
- Works with standalone files or within templates
Example usage:
// Validate a specific azure.yaml file
await validateAzureYaml('/path/to/azure.yaml');
// Validate azure.yaml in current directory
await validateAzureYaml();
Or use it through MCP server:
Can you validate my azure.yaml file?
Requirements
- Node.js 18+
- Azure Developer CLI (azd) installed
License
MIT
相关推荐
You're in a stone cell – can you get out? A classic choose-your-adventure interactive fiction game, based on a meticulously-crafted playbook. With a medieval fantasy setting, infinite choices and outcomes, and dice!
Best-in-class AI domain names scoring engine and availability checker. Brandability, domain worth, root keywords and more.
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Dify is an open-source LLM app development platform. Dify's intuitive interface combines AI workflow, RAG pipeline, agent capabilities, model management, observability features and more, letting you quickly go from prototype to production.
🔥 1Panel provides an intuitive web interface and MCP Server to manage websites, files, containers, databases, and LLMs on a Linux server.
an easy-to-use dynamic service discovery, configuration and service management platform for building AI cloud native applications.
⛓️RuleGo is a lightweight, high-performance, embedded, next-generation component orchestration rule engine framework for Go.
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/MCP/Docker/Zotero
Run existing Model Context Protocol (MCP) stdio-based servers in AWS Lambda functions
A plugin-based gateway that orchestrates other MCPs and allows developers to build upon it enterprise-grade agents.
Reviews
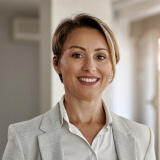
user_OrqAPCHD
I recently tried out the mcp-azd-template by spboyer and it exceeded my expectations. The template is incredibly user-friendly and streamlined for efficiency. It offers clear, concise instructions, making deployment a breeze even for those new to MCP applications. Highly recommend for anyone looking to optimize their project setup!
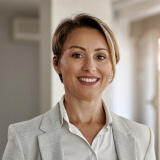
user_YRaq1GK9
The mcp-azd-template by spboyer is a fantastic tool for anyone looking to streamline their application development process. With its user-friendly interface and comprehensive features, it significantly simplifies complex tasks. The seamless integration and efficient performance make it a must-have for developers. Highly recommend!
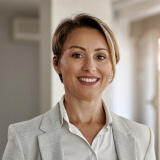
user_HuD9BKxK
I'm a huge fan of the mcp-azd-template by spboyer! This template is incredibly well-structured and makes setting up new projects a breeze. The clear organization and ease of use have significantly improved my workflow. Highly recommended for anyone looking to streamline their development process!
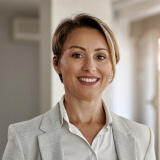
user_8KBKWcxA
The mcp-azd-template by spboyer is a fantastic tool that streamlined my development process. Its seamless integration and user-friendly setup make it a must-have for any developer. The template's detailed guidelines and comprehensive structure significantly reduce setup time, allowing me to focus on building and scaling my projects efficiently. Highly recommended!
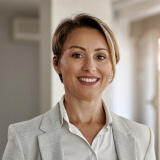
user_sesueMDF
The mcp-azd-template by spboyer is a fantastic resource for anyone working with Azure Development. It's incredibly user-friendly and offers a seamless start into your projects. The well-documented template saves a lot of time and ensures best practices. Highly recommend it to all developers!
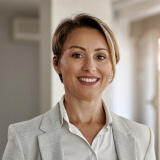
user_ui410He2
I have been using the mcp-azd-template by spboyer and it's an outstanding resource for Azure development. It simplifies the setup process and provides a solid foundation which saves a lot of time. The integration is seamless and the template is well-structured. Highly recommend this for anyone working with Azure!
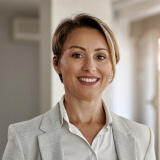
user_GozQwjJj
The mcp-azd-template by spboyer is an exceptional tool for initializing Azure development projects. It's user-friendly, well-documented, and saves a lot of setup time. Highly recommended for anyone looking to streamline their cloud application development process.
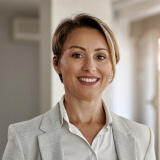
user_O76BiPvP
As an avid user of MCP applications, I am thoroughly impressed with the mcp-azd-template by spboyer. This template has streamlined my workflow significantly, and the ease of customization makes it perfect for various projects. The detailed welcome information and straightforward start URL show the author's commitment to user experience. Highly recommended for anyone looking for a reliable and efficient template!
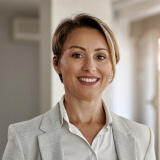
user_3m5I1Wef
The mcp-azd-template by spboyer is a truly remarkable tool for developers. Its comprehensive and user-friendly design makes it a breeze to deploy and manage your Azure environments efficiently. I highly recommend it for anyone looking to streamline their cloud deployment process. The support and documentation provided are top-notch, further enhancing the overall experience. Five stars!