I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
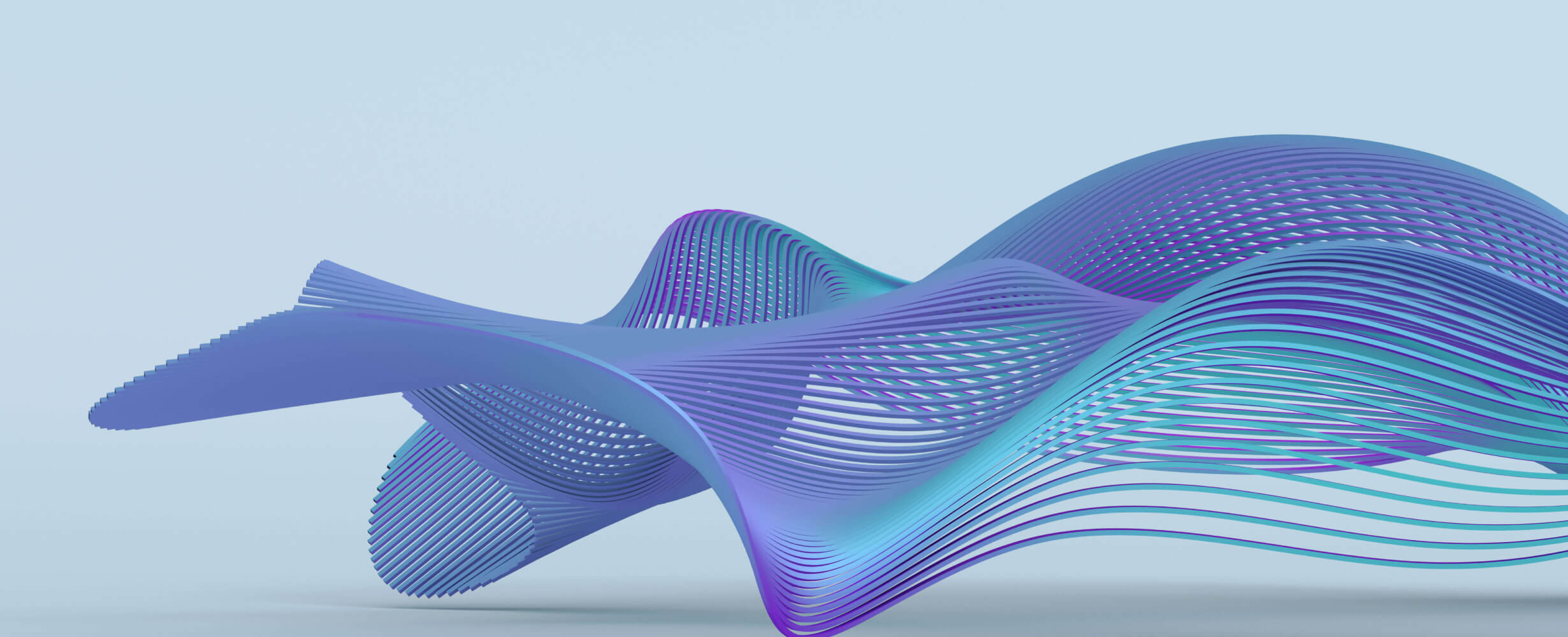
mcp-go-sdk
This SDK provides a Go implementation of the Machine Control Protocol (MCP), enabling bidirectional communication between clients and servers for tool execution, resource access, and prompt handling. Based onhttps://github.com/modelcontextprotocolSDK
1
Github Watches
1
Github Forks
1
Github Stars
MCP Go SDK
This SDK provides a Go implementation of the Model Context Protocol (MCP), enabling bidirectional communication between clients and servers for tool execution, resource access, and prompt handling.
Features
-
Transport Layer
- Multiple transport options (stdio, SSE, WebSocket)
- Bidirectional communication
- Configurable endpoints and settings
-
Server Implementation
- Tool registration and execution
- Resource pattern matching and access
- Prompt template rendering
- Session management
- Reflection-based handler invocation
-
Core Protocol Types
- JSON-RPC message handling
- MCP-specific types (tools, resources, prompts)
- Capabilities and initialization
Installation
go get github.com/SetiabudiResearch/mcp-go-sdk
Quick Start
Here's a simple example of creating an MCP server:
package main
import (
"context"
"log"
"github.com/SetiabudiResearch/mcp-go-sdk/pkg/mcp/server"
"github.com/SetiabudiResearch/mcp-go-sdk/pkg/mcp/transport"
)
func main() {
// Create a new server
srv := server.NewServer("Example Server")
// Add a tool
srv.AddTool("greet", func(name string) string {
return "Hello, " + name + "!"
}, "Greet a person")
// Create a session
session := server.NewSession(context.Background(), srv)
// Create and start a transport
t := transport.NewStdioTransport(session)
if err := t.Start(); err != nil {
log.Fatal(err)
}
}
Usage Guide
Creating a Server
// Create a new server with a name
srv := server.NewServer("My Server")
// Optionally configure server capabilities
srv.WithCapabilities(protocol.ServerCapabilities{
SupportsAsync: true,
})
Adding Tools
// Add a synchronous tool
srv.AddTool("myTool", func(arg1 string, arg2 int) (string, error) {
return fmt.Sprintf("Processed %s with %d", arg1, arg2), nil
}, "Tool description")
// Add an asynchronous tool
srv.AddAsyncTool("longRunningTool", func(params string) error {
// Long-running operation
return nil
}, "Async tool description")
Adding Resources
// Add a resource with pattern matching
srv.AddResource("files/{path}", func(path string) ([]byte, error) {
return ioutil.ReadFile(path)
}, "Access files")
// Resource patterns support multiple parameters
srv.AddResource("api/{version}/{endpoint}", func(version, endpoint string) (interface{}, error) {
return callAPI(version, endpoint)
}, "API access")
Adding Prompts
// Add a simple text prompt
srv.AddPrompt("confirm", func(action string) string {
return fmt.Sprintf("Are you sure you want to %s?", action)
}, "Confirmation prompt")
// Add a multi-message prompt
srv.AddPrompt("chat", func(context string) []protocol.PromptMessage {
return []protocol.PromptMessage{
{
Role: protocol.RoleAssistant,
Content: protocol.TextContent{
Type: "text",
Text: "How can I help you with " + context + "?",
},
},
}
}, "Chat prompt")
Transport Configuration
// Create a session
session := server.NewSession(context.Background(), srv)
// Stdio transport (for CLI applications)
t := transport.NewStdioTransport(session)
// WebSocket transport (for web applications)
t := transport.NewWebSocketTransport(session, transport.WithAddress(":8080"))
// Server-Sent Events transport (for web browsers)
t := transport.NewSSETransport(session, transport.WithAddress(":8080"))
// Start the transport
if err := t.Start(); err != nil {
log.Fatal(err)
}
Example Applications
See the examples directory for complete example applications:
- File Server: A complete file server implementation using MCP
- Calculator: A simple calculator service
- Chat Bot: An example chat bot using prompts
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
License
This project is licensed under the MIT License - see the LICENSE file for details.
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
This GPT assists in finding a top-rated business CPA - local or virtual. We account for their qualifications, experience, testimonials and reviews. Business operators provide a short description of your business, services wanted, and city or state.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
Mirror ofhttps://github.com/agentience/practices_mcp_server
Mirror ofhttps://github.com/bitrefill/bitrefill-mcp-server
Reviews
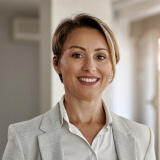
user_1DT7flKR
As a devoted user of the mcp-go-sdk, I highly recommend it for anyone working with Go. Created by SetiabudiResearch, this SDK simplifies interactions with MCP services and enhances development efficiency. The comprehensive documentation and clear welcome message make the onboarding process smooth and straightforward. Check it out at https://github.com/SetiabudiResearch/mcp-go-sdk.