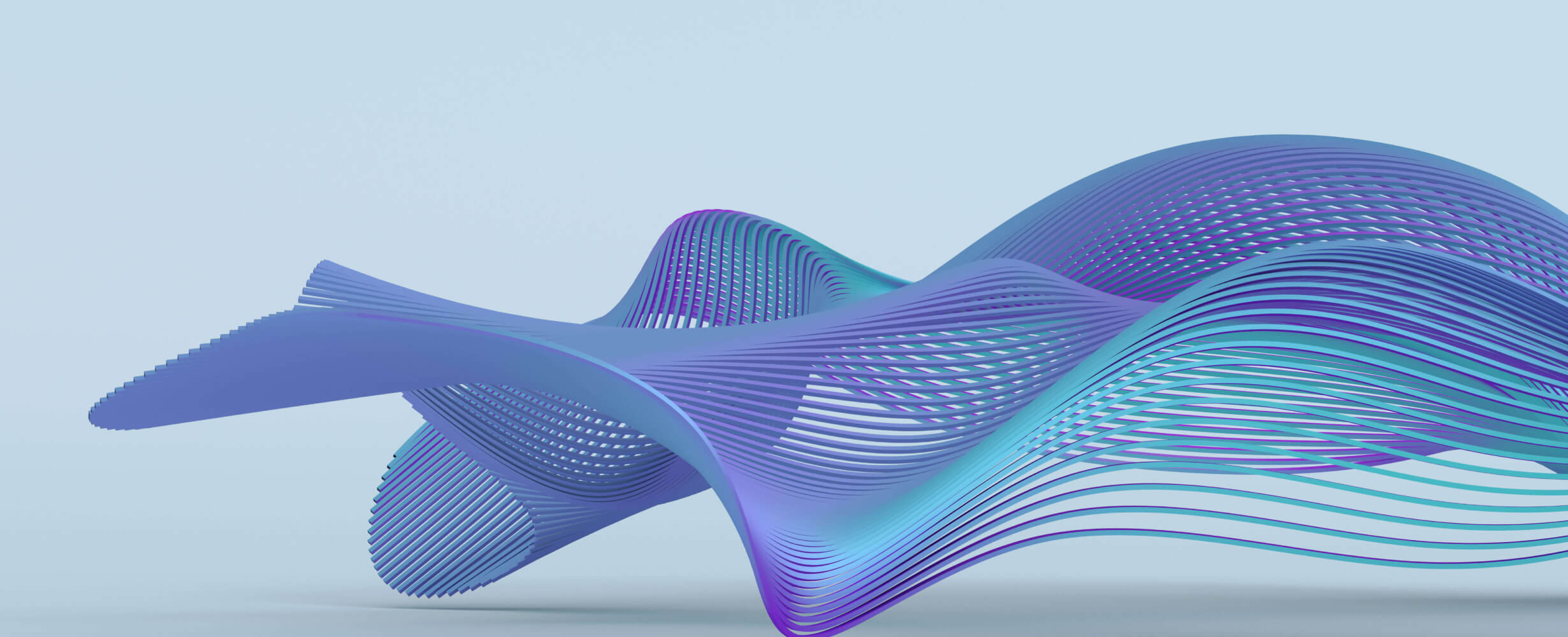
WooCommerce-MCP-server
1
Github Watches
0
Github Forks
1
Github Stars
WooCommerce MCP Server
A Model Context Protocol (MCP) server for integrating WooCommerce with Claude and other AI assistants.
Overview
This server provides tools for AI assistants to interact with a WooCommerce store, allowing them to:
- Fetch recent orders with optional filtering
- Retrieve detailed information about specific orders by ID
The server implements the Model Context Protocol specification, making it compatible with MCP-enabled AI assistants like Claude for Desktop.
Prerequisites
- Node.js v18 or higher
- A WooCommerce store with REST API access
- WooCommerce API credentials (consumer key and secret)
Installation
- Clone this repository:
git clone https://github.com/techspawn/woocommerce-mcp-server.git
cd woocommerce-mcp-server
- Install dependencies:
npm install
Configuration
You need to configure your WooCommerce API credentials. You can do this by:
- Setting environment variables when running the server:
WOOCOMMERCE_URL=https://your-store.com \
WOOCOMMERCE_CONSUMER_KEY=your-consumer-key \
WOOCOMMERCE_CONSUMER_SECRET=your-consumer-secret \
node index.js
- Or by editing the default values in the
index.js
file:
const woocommerceConfig = {
url: process.env.WOOCOMMERCE_URL || 'https://your-store.com',
consumerKey: process.env.WOOCOMMERCE_CONSUMER_KEY || 'your-consumer-key',
consumerSecret: process.env.WOOCOMMERCE_CONSUMER_SECRET || 'your-consumer-secret',
version: 'wc/v3'
};
Running the Server
To run the server directly:
node index.js
Or using the npm script:
npm start
Integration with Claude for Desktop
To connect this server to Claude for Desktop:
-
Make sure you have Claude for Desktop installed
-
Open your Claude Desktop configuration file located at:
- Windows:
%USERPROFILE%\AppData\Roaming\Claude\claude_desktop_config.json
- macOS:
~/Library/Application Support/Claude/claude_desktop_config.json
- Linux:
~/.config/Claude/claude_desktop_config.json
- Windows:
-
Add your WooCommerce MCP server configuration (create the file if it doesn't exist):
{
"mcpServers": {
"woocommerce": {
"command": "node",
"args": [
"/ABSOLUTE/PATH/TO/woocommerce-mcp-server/index.js"
],
"env": {
"WOOCOMMERCE_URL": "https://your-store.com",
"WOOCOMMERCE_CONSUMER_KEY": "your-consumer-key",
"WOOCOMMERCE_CONSUMER_SECRET": "your-consumer-secret"
}
}
}
}
- Save the file and restart Claude for Desktop
Available Tools
getRecentOrders
Fetches a list of recent orders from your WooCommerce store.
Parameters:
-
status
(optional): Filter orders by status (e.g., "processing", "completed", "on-hold") -
limit
(optional, default: 5): Number of orders to return
getOrderById
Retrieves detailed information about a specific order.
Parameters:
-
id
: The order ID to retrieve
Building Your Own MCP Server with JavaScript
This section provides a guide to creating your own MCP server using JavaScript/Node.js.
1. Set up a new project
mkdir my-mcp-server
cd my-mcp-server
npm init -y
2. Install dependencies
npm install @modelcontextprotocol/sdk axios zod
3. Create your server file (index.js)
Start with the basic structure:
import { McpServer } from "@modelcontextprotocol/sdk/server/mcp.js";
import { StdioServerTransport } from "@modelcontextprotocol/sdk/server/stdio.js";
import { z } from "zod";
// Initialize server
const server = new McpServer({
name: "My MCP Service",
version: "1.0.0",
});
// Define and register your tools
server.tool(
"myToolName",
{
// Define parameters using Zod schemas
param1: z.string().describe("Description of param1"),
param2: z.number().optional().describe("Optional parameter")
},
async ({ param1, param2 }) => {
// Tool implementation logic here
const result = `Processed ${param1} with value ${param2 || 'none'}`;
// Return result in the expected format
return {
content: [
{
type: "text",
text: result
}
]
};
}
);
// Connect the server
const transport = new StdioServerTransport();
await server.connect(transport);
4. Make your package.json ES module compatible
{
"type": "module",
"scripts": {
"start": "node index.js"
}
}
5. Define tools
MCP tools are defined with three components:
- Name: A unique identifier for the tool
- Parameters: Schema for input parameters (using Zod)
- Handler: Async function that processes the inputs and returns results
Example:
server.tool(
"calculateTotal",
{
items: z.array(
z.object({
name: z.string(),
price: z.number(),
quantity: z.number().int().positive()
})
).describe("Array of items to calculate total for")
},
async ({ items }) => {
const total = items.reduce((sum, item) => sum + (item.price * item.quantity), 0);
return {
content: [
{
type: "text",
text: `Total: $${total.toFixed(2)}`
}
]
};
}
);
6. Testing locally
You can test your MCP server locally using the stdio transport:
node index.js
7. Debugging tips
- Use
console.error()
for debugging, notconsole.log()
which interferes with stdio transport - Check the logs in Claude for Desktop for errors
- Ensure your tool handlers properly handle exceptions
Resources
License
MIT
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Descubra la colección más completa y actualizada de servidores MCP en el mercado. Este repositorio sirve como un centro centralizado, que ofrece un extenso catálogo de servidores MCP de código abierto y propietarios, completos con características, enlaces de documentación y colaboradores.
Manipulación basada en Micrypthon I2C del expansor GPIO de la serie MCP, derivada de AdaFruit_MCP230xx
Espejo dehttps: //github.com/agentience/practices_mcp_server
La aplicación AI de escritorio todo en uno y Docker con trapo incorporado, agentes de IA, creador de agentes sin código, compatibilidad de MCP y más.
Espejo de https: //github.com/bitrefill/bitrefill-mcp-server
Un poderoso complemento Neovim para administrar servidores MCP (protocolo de contexto del modelo)
Reviews
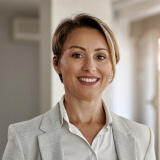
user_4nlny3u5
As a devoted user of the woocommerce-mcp-server, I find this plugin by opestro incredibly powerful and user-friendly. It's an essential tool for anyone managing a WooCommerce store, seamlessly integrating with multiple channels. The documentation provided on GitHub is thorough, making setup and customization a breeze. Highly recommend!