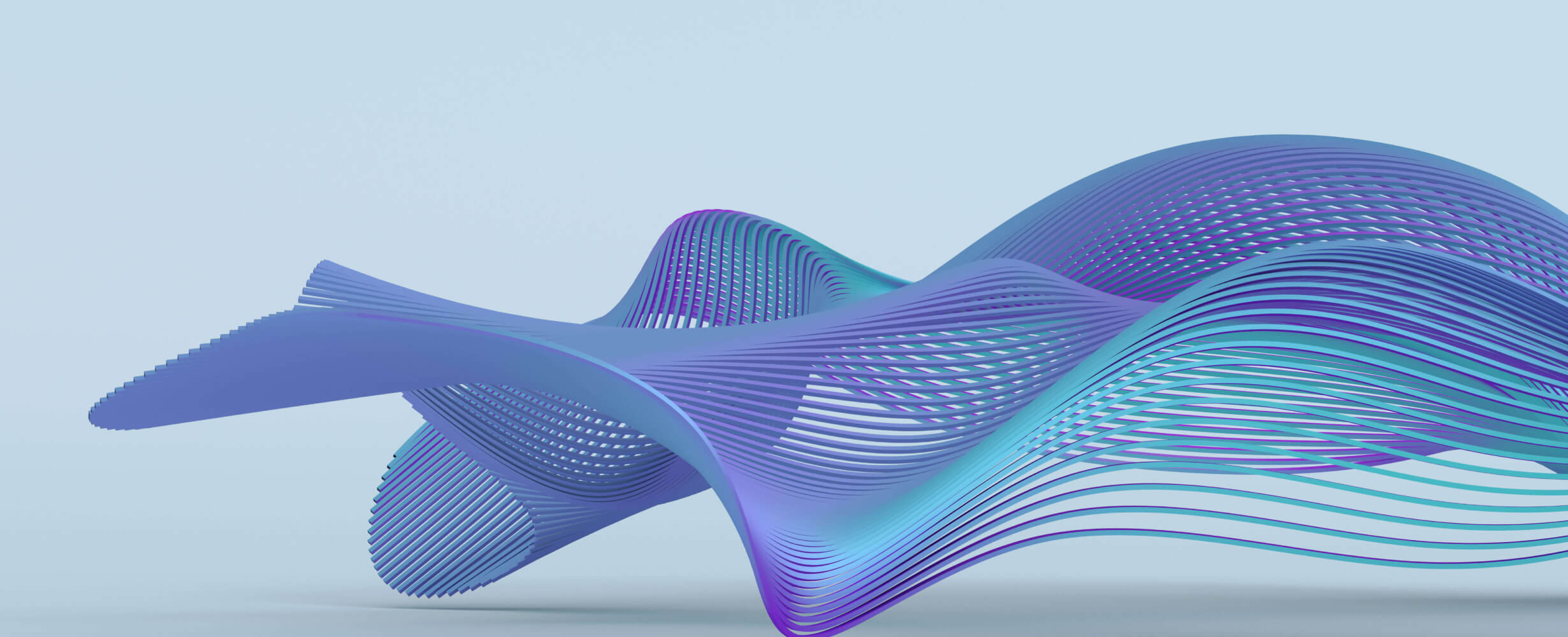
Openalgo-MCP
Documentación
3 years
Works with Finder
0
Github Watches
0
Github Forks
0
Github Stars
OpenAlgo MCP - AI Trading Assistant
An AI-powered trading assistant platform for OpenAlgo, leveraging Machine Conversation Protocol (MCP) and Large Language Models to provide intelligent trading capabilities.
Overview
OpenAlgo MCP integrates the powerful OpenAlgo trading platform with advanced AI capabilities through:
- An MCP server that exposes OpenAlgo API functions as tools for AI interaction
- An intelligent client application providing a conversational interface for trading
This bridge between OpenAlgo's trading capabilities and AI allows for a natural language interface to complex trading operations, making algorithmic trading more accessible to users of all technical backgrounds.
Key Features
Comprehensive Trading Capabilities
- Order Management: Place, modify, and cancel orders with support for various order types (market, limit, stop-loss)
- Advanced Order Types: Basket orders, split orders, and smart orders with position sizing
- Market Data Access: Real-time quotes, market depth, and historical data
- Portfolio Management: Track holdings, positions, order books, and trade history
- Account Information: Monitor funds, margins, and trading limits
Intelligent Symbol Format Handling
- Smart parsing and formatting of instrument symbols across exchanges
- Support for equity, futures, and options symbology
- Built-in knowledge of common indices and exchange-specific formats
AI-Powered Trading Assistant
- Natural language interface for all trading operations
- Contextual understanding of trading terminology and concepts
- Guided assistance for complex trading operations
- Real-time data presentation in human-readable formats
Project Structure
openalgo-mcp/
├── .env # Common environment configuration
├── .env.example # Example configuration template
├── requirements.txt # Common dependencies for both client and server
├── LICENSE # MIT License
├── server/ # MCP Server implementation
│ ├── server.py # OpenAlgo MCP server code
└── client/ # Client implementation
├── trading_agent.py # AI assistant client code
Installation Guide
Prerequisites
- Python 3.9+ installed
- OpenAlgo platform installed and configured
- OpenAI API key (for the client component)
Step 1: Clone the Repository
git clone https://github.com/marketcalls/openalgo-mcp.git
cd openalgo-mcp/mcpserver
Step 2: Set Up Environment
# Create and activate virtual environment
python -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
# Install dependencies
pip install -r requirements.txt
Step 3: Configure Environment Variables
# Copy example environment file
cp .env.example .env
# Edit the .env file with your API keys and settings
# vim .env or use any text editor
Usage
Starting the MCP Server
cd server
python server.py
The server supports the following options:
-
--api-key
: OpenAlgo API key (alternative to setting in .env) -
--host
: OpenAlgo API host URL (default: http://127.0.0.1:5000) -
--port
: Server port (default: 8001) -
--mode
: Server transport mode - 'stdio' or 'sse' (default: sse)
Starting the Trading Assistant Client
cd client
python trading_agent.py
The client supports these options:
-
--host
: MCP server host (default: from .env) -
--port
: MCP server port (default: from .env) -
--model
: OpenAI model to use (default: from .env)
Configuration
The project uses a unified configuration approach with environment variables:
- Common configuration is stored in the root
.env
file - Component-specific configuration can be set in
server/.env
orclient/.env
- Common settings will be loaded first, then possibly overridden by component-specific settings
Required API Keys
-
OpenAlgo API Key - Set in
.env
asOPENALGO_API_KEY
- Required for accessing the OpenAlgo trading platform
- Obtain from your OpenAlgo account dashboard
-
OpenAI API Key - Set in
.env
asOPENAI_API_KEY
(for the client only)- Required for the AI assistant capabilities
- Obtain from OpenAI Platform
Technical Capabilities
The OpenAlgo MCP implementation provides comprehensive API coverage including:
-
Order Management:
-
place_order
: Standard order placement -
modify_order
: Order modification with parameter validation -
cancel_order
: Order cancellation by ID
-
-
Advanced Order Types:
-
place_basket_order
: Place multiple orders simultaneously -
place_split_order
: Split large orders into smaller chunks -
place_smart_order
: Position-aware order placement
-
-
Market Data:
-
get_quote
: Latest market quotes -
get_depth
: Order book depth data -
get_history
: Historical price data with various timeframes
-
-
Account Information:
-
get_funds
: Available funds and margin -
get_holdings
: Portfolio holdings -
get_position_book
,get_order_book
,get_trade_book
: Trading records
-
-
Symbol Information:
-
get_symbol_metadata
: Detailed symbol information -
get_all_tickers
: Available trading symbols -
get_intervals
: Supported timeframes for historical data
-
The implementation uses FastMCP with SSE (Server-Sent Events) transport for real-time communication and includes proper error handling, logging, and parameter validation.
Server Implementation Details
The OpenAlgo MCP Server is built using the FastMCP library and exposes OpenAlgo trading functionality through a comprehensive set of tools. It uses Server-Sent Events (SSE) as the primary transport mechanism for real-time communication.
Server Architecture
- Framework: Uses FastMCP with Starlette for the web server
- Transport: Server-Sent Events (SSE) for real-time bidirectional communication
- API Client: Wraps the OpenAlgo API with appropriate error handling and logging
- Configuration: Uses environment variables with command-line override capabilities
Available API Tools
The server exposes over 15 trading-related tools, including:
- Order Management: place_order, modify_order, cancel_order, get_order_status
- Advanced Orders: place_basket_order, place_split_order, place_smart_order
- Market Data: get_quote, get_depth, get_history, get_intervals
- Account Information: get_funds, get_holdings, get_position_book, get_order_book, get_trade_book
- Symbol Information: get_symbol_metadata, get_all_tickers
Client Implementation Details
The Trading Assistant client provides a user-friendly interface to interact with the OpenAlgo platform through natural language. It uses OpenAI's language models to interpret user commands and invoke the appropriate trading functions.
Client Architecture
- Framework: Uses Agno agent framework with OpenAI Chat models
- UI: Rich console interface with custom styling for an enhanced terminal experience
- Symbol Helper: Built-in utilities for correct symbol formatting across exchanges
- Error Handling: Comprehensive exception handling with user-friendly feedback
Trading Assistant Capabilities
- Natural Language Interface: Understands trading terminology and concepts
- Symbol Format Assistance: Helps construct proper symbol formats for equities, futures, and options
- Data Presentation: Formats market data in clean, readable formats
- Contextual Awareness: Maintains conversation history to provide contextual responses
Troubleshooting Guide
Common Issues
Connection Issues
If you're having trouble connecting to the MCP server:
-
Verify the server is running:
cd server python server.py
You should see output indicating the server is running on the configured port.
-
Check environment variables:
- Ensure
MCP_HOST
andMCP_PORT
in.env
match the server's configuration - Verify that
SERVER_PORT
is the same asMCP_PORT
- Ensure
-
Test local connectivity:
- Try accessing
http://localhost:8001/sse
in your browser (replace 8001 with your configured port) - You should see a message indicating the endpoint is for SSE connections
- Try accessing
API Authentication Issues
If you see 403 Forbidden or authentication errors:
-
Check your API key:
- Verify your OpenAlgo API key in the
.env
file is correct and active - Ensure the API key has the necessary permissions for the operations you're trying to perform
- Verify your OpenAlgo API key in the
-
Verify API host:
- Make sure
OPENALGO_API_HOST
points to the correct endpoint - For testing, the default value
http://127.0.0.1:5000
should work if you're running OpenAlgo locally
- Make sure
Client Issues
-
Silent failures in the client:
- The client uses a SilentFilter for logging to provide a clean interface
- If you suspect issues, temporarily modify the logging configuration in
trading_agent.py
- Check that the OpenAI API key is valid if you experience model generation failures
Acknowledgements and Credits
This project is made possible by the following open-source projects and tools:
Core Technologies
-
OpenAlgo: The powerful trading platform that powers all trading operations in this project
-
Model Context Protocol (MCP): The communication protocol that enables AI agents to use tools and APIs
-
Agno: The agent framework used for building the trading assistant client
Inspiration
This project was inspired by Zerodha MCP, which pioneered the use of Machine Conversation Protocol for trading applications. The OpenAlgo MCP project adapts and extends this concept for the OpenAlgo trading platform, with a focus on enhanced symbol handling, comprehensive trading operations, and a more user-friendly interface.
Symbol Formatting Issues
If your symbol-related requests are failing:
-
Follow format guidelines:
- Equity symbols: Simple uppercase symbol (e.g.,
INFY
,SBIN
) - Futures:
[BaseSymbol][Year][Month][Date]FUT
(e.g.,BANKNIFTY24APR24FUT
) - Options:
[BaseSymbol][Date][Month][Year][Strike][OptionType]
(e.g.,NIFTY28MAR2420800CE
)
- Equity symbols: Simple uppercase symbol (e.g.,
-
Use the SymbolHelper class:
- The client includes formatting assistance methods that can help construct proper symbols
Debugging Mode
For more detailed logging, enable debugging in the .env
file:
SERVER_DEBUG=true
This will output additional information to help diagnose connection and API issues.
License
This project is licensed under the Apache-2.0 license - see the LICENSE file for details.
Acknowledgments
- This project was inspired by Zerodha MCP, which is licensed under the Apache License 2.0. While no code has been directly copied, this project builds upon the concept and architecture introduced by Zerodha MCP.
- OpenAlgo for the powerful trading platform
- MCP (Machine Conversation Protocol) for the communication framework
- AGNO for the AI agent infrastructure
相关推荐
🔥 1Panel proporciona una interfaz web intuitiva y un servidor MCP para administrar sitios web, archivos, contenedores, bases de datos y LLM en un servidor de Linux.
🧑🚀 全世界最好的 llM 资料总结(数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Resumen de los mejores recursos del mundo.
⛓️Rulego es un marco de motor de regla de orquestación de componentes de alta generación de alto rendimiento, de alto rendimiento y de alto rendimiento para GO.
Flock es una plataforma de bajo código de flujo de trabajo para construir rápidamente chatbots, trapo y coordinar equipos de múltiples agentes, impulsados por Langgraph, Langchain, Fastapi y Nextjs.
Traducción de papel científico en PDF con formatos preservados - 基于 Ai 完整保留排版的 PDF 文档全文双语翻译 , 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 等服务 等服务 等服务 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 cli/mcp/docker/zotero
Plataforma de automatización de flujo de trabajo de código justo con capacidades de IA nativas. Combine el edificio visual con código personalizado, auto-anfitrión o nube, más de 400 integraciones.
Cree fácilmente herramientas y agentes de LLM utilizando funciones Plain Bash/JavaScript/Python.
😎简单易用、🧩丰富生态 - 大模型原生即时通信机器人平台 | 适配 Qq / 微信(企业微信、个人微信) / 飞书 / 钉钉 / Discord / Telegram / Slack 等平台 | 支持 Chatgpt 、 Deepseek 、 DiFy 、 Claude 、 Gemini 、 Xai 、 PPIO 、 Ollama 、 LM Studio 、阿里云百炼、火山方舟、 Siliconflow 、 Qwen 、 Moonshot 、 Chatglm 、 SillyTraven 、 MCP 等 LLM 的机器人 / Agente | Plataforma de bots de mensajería instantánea basada en LLM, admite Discord, Telegram, WeChat, Lark, Dingtalk, QQ, Slack
Reviews
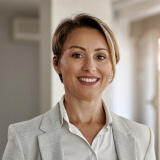
user_GqbeIg7O
I'm a dedicated user of openalgo-mcp by marketcalls and I'm genuinely impressed! The platform is intuitive and offers robust tools for market analysis. The seamless integration and comprehensive resources have significantly enhanced my trading strategies. Highly recommend this for anyone serious about financial market analysis and trading.
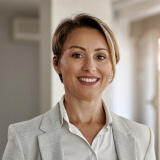
user_QwrpPhov
As a dedicated user of openalgo-mcp by marketcalls, I am thoroughly impressed by its robust algorithmic trading features. This tool has significantly enhanced my trading strategies with its user-friendly interface and comprehensive data analysis. I highly recommend openalgo-mcp to anyone involved in algorithmic trading.
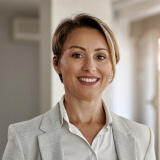
user_QZku5jqs
Openalgo-MCP by Marketcalls is an exceptional product for trading enthusiasts. As a loyal user, I appreciate its precision and user-friendly interface. The real-time data and efficient algorithms have significantly improved my trading strategies. Highly recommend it!
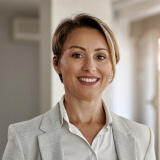
user_v7VhP0eJ
I've been using openalgo-mcp by marketcalls, and it has been an amazing experience. The platform is user-friendly and efficient, offering excellent tools for market analysis. It significantly simplifies trading strategies execution. Highly recommended for both beginners and experienced traders.
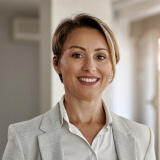
user_KY1Ornse
OpenAlgo-MCP is an exceptional tool for algorithmic trading enthusiasts. Created by Marketcalls, this product has streamlined my trading strategies significantly. The intuitive design and robust features make it a must-have for anyone serious about market analysis. Its integration capabilities and user-friendly interface have truly enhanced my trading performance. Highly recommend giving it a try!
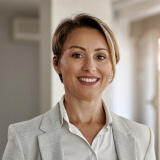
user_QawPS5C8
As a loyal user of openalgo-mcp by marketcalls, I am thoroughly impressed by its functionality and performance. This platform has revolutionized my trading strategy through its robust algorithmic features and user-friendly interface. The seamless start URL and welcoming information only add to the overall positive experience. Highly recommended for anyone serious about market trading!