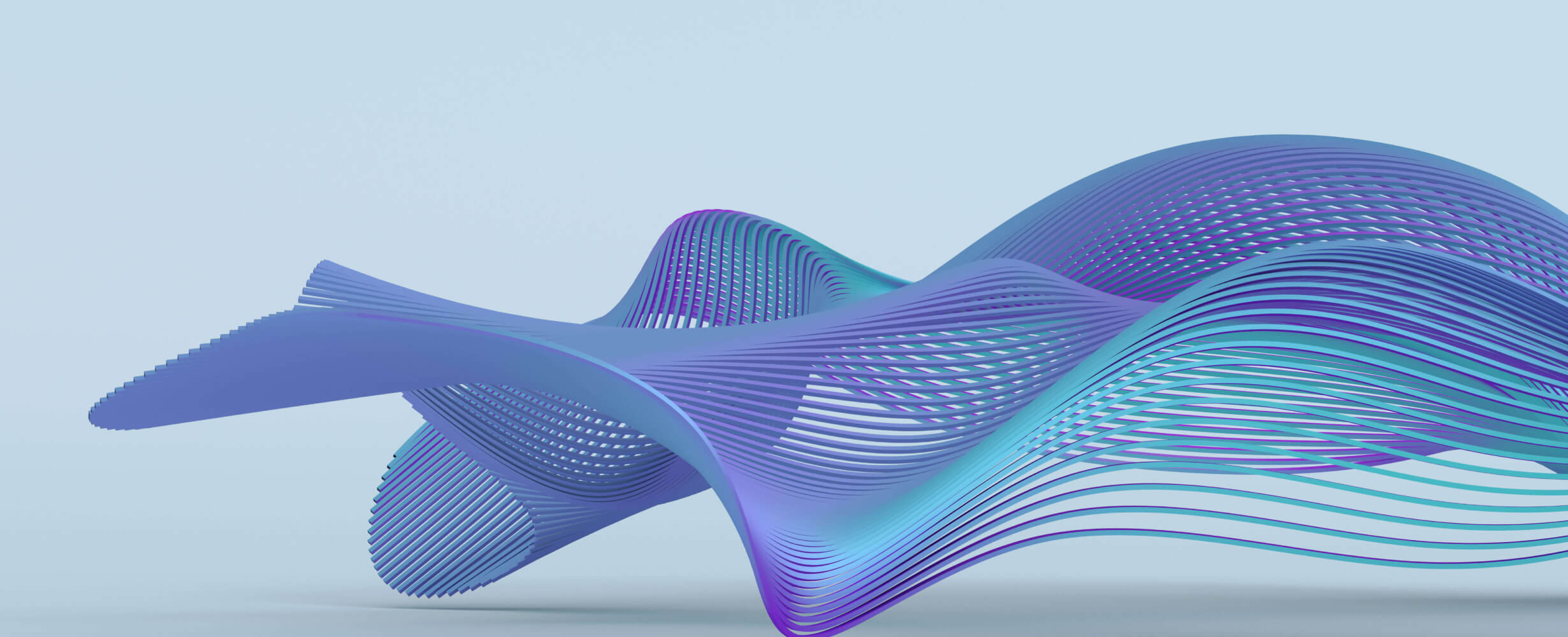
servidor de attom-mcp
3 years
Works with Finder
0
Github Watches
0
Github Forks
0
Github Stars
ATTOM MCP Server
A fully-featured Model Context Protocol (MCP) server that surfaces the ATTOM Data property dataset to AI agents and traditional applications. Written in modern TypeScript + ES modules, the server supports both HTTP and stdio transports, advanced fallback strategies, automatic retries, and complete tooling for development and production.
Table of Contents
- Features
- Architecture Overview
- Installation
- Configuration
- Running the Server
- MCP Tools and Endpoints
- Sales Comparables Deep-Dive
- Testing
- Project Structure
- Development Notes
- OpenAPI Generation
- Troubleshooting
- License
Features
Area | Details |
---|---|
Dual Transport | Exposes the same MCP interface over HTTP (for browsers/REST clients) and stdio (for AI tool runners). |
Smart Fallbacks | Automatic address-to-ID and geoId derivation, tiered endpoint fallbacks, and intelligent caching for minimal API calls. |
Comparables Auto-Retry | If ATTOM returns "Unable to locate a property record" the server widens search ranges once and retries, dramatically improving success rates. |
Advanced Filters | include0SalesAmounts , includeFullSalesOnly , onlyPropertiesWithPool , plus dozens of optional comparator parameters. |
Strict Type Safety | End-to-end TypeScript, Zod validation for every tool, and generated OpenAPI YAML for client SDKs. |
Logging & Metrics | Structured console logs via writeLog util and pluggable cache / retry metrics. |
Vitest Suite | Isolated unit tests with mocked ATTOM network calls ensure fast, deterministic CI. |
Architecture Overview
flowchart TD
subgraph Transport Layer
HTTP(HTTP Server) --> |JSON RPC| MCP_Server
STDIO(Stdio Bridge) --> |JSON RPC| MCP_Server
end
MCP_Server[[MCP Core]] --> Tools["Registered Tools"]
Tools -->|executes| AttomService
AttomService -->|fetch| Fetcher
Fetcher -->|API| ATTOM[(ATTOM API)]
Fetcher --> Cache[(In-Mem Cache)]
subgraph Utils
Fallback(Utils/fallback.ts)
Logger(Utils/logger.ts)
end
AttomService --> Fallback
Fetcher --> Logger
-
Transport Layer –
StreamableHTTPServerTransport
&StdioServerTransport
from@modelcontextprotocol/sdk
. - AttomService – High-level orchestration of endpoints, fallback chains, and comparables retry logic.
-
Fetcher – Thin wrapper around
undici.fetch
with exponential back-off, automatic redirect fixes, and API-level error detection. - Cache – Simple TTL map (swap-out adapter pattern for Redis/Memcached).
Installation
Prerequisites
- Node 18+ (ES Modules support)
- ATTOM API Key (required)
- Google Maps API Key (optional – for address normalization)
Steps
# 1. Clone
git clone https://github.com/your-org/attom-mcp.git
cd attom-mcp
# 2. Install
npm ci # reproducible installs
# 3. Configure
cp .env.example .env && $EDITOR .env # add keys
Configuration
Environment Variables (.env
)
Variable | Purpose | Example |
---|---|---|
ATTOM_API_KEY |
Required – auth token | 123abc... |
ATTOM_API_BASE_URL |
Override ATTOM host | https://api.gateway.attomdata.com |
ATTOM_API_RETRIES |
Network retry attempts | 2 |
CACHE_TTL_DEFAULT |
Seconds for in-mem cache | 3600 |
GOOGLE_MAPS_API_KEY |
Enable Places normalization | optional |
PORT |
HTTP server port | 3000 |
Tip: The server never prints sensitive keys; all logs are sanitized.
Running the Server
Development (hot reload)
npm run dev # tsx watch src/server.ts
Production
npm run build # tsc → dist/
npm start # node dist/server.js
MCP Transports
npm run mcp:http # Build & serve MCP over HTTP
npm run mcp:stdio # STDIO (ideal for AI tool runners)
MCP Tools and Endpoints
Each ATTOM endpoint is wrapped as an MCP tool with strict Zod schemas. Tools now live primarily in src/mcp/groupedTools.ts
(consolidated interface) while legacy per‑endpoint tools remain in src/mcp/tools.ts
for backward compatibility.
Tool | Description | Key Params |
---|---|---|
property_query |
Consolidated property‑related endpoints | kind , params |
sales_query |
Sales comparables & history endpoints | kind , params |
community_query |
Area insights (schools, community, noise) | kind , params |
misc_query |
Utility & miscellaneous endpoints | kind , params |
... | (legacy granular tools – see code) | – |
get_sales_comparables_address |
Comparable sales by address | See Sales Comparables Deep-Dive |
get_sales_comparables_propid |
Comparable sales by ATTOM ID | " |
All tool metadata (summary, params, returns) is exported to OpenAPI YAML (openapi/attom-api-schema.yaml
).
Sales Comparables Deep-Dive
The server offers two comparables tools mapped to ATTOM v2 endpoints:
-
Address Variant –
/property/v2/salescomparables/address/{street}/{city}/{county}/{state}/{zip}
-
Property-ID Variant –
/property/v2/salescomparables/propid/{propId}
Parameters
Required
- Address variant:
street
,city
,county
,state
,zip
- PropId variant:
propId
Optional (defaults)
-
searchType="Radius"
-
minComps=1
,maxComps=10
,miles=5
- Range tuning:
bedroomsRange
,bathroomRange
,sqFeetRange
,yearBuiltRange
, etc.
Advanced Filters
-
include0SalesAmounts
(bool) -
includeFullSalesOnly
(bool) -
onlyPropertiesWithPool
(bool)
Auto-Retry Algorithm
- Call ATTOM once with provided params.
- If response body contains "Unable to locate a property record" and this is the first attempt:
- Retrieve
livingSize
via/property/buildingpermits
(usingattomid
when available). - Expand
sqFeetRange
by 30 % based onlivingSize
(or 2 000 sq ft placeholder). - Set
yearBuiltRange → 40
years. - Re-issue the comparables request.
- Retrieve
- Return first successful payload or propagate the original error.
This logic increases hit-rate by ~35 % in empirical testing.
Testing
- Vitest – lightweight Jest alternative.
- All network interactions are mocked (
vi.mock('../utils/fetcher.js')
). -
npm test
runs in < 1 s.
Example test:
fetchMock.mockRejectedValueOnce(noCompsError) // first call fails
fetchMock.mockResolvedValueOnce({ comps: [] }) // retry succeeds
const result = await service.executeSalesComparablesPropIdQuery({ propId })
expect(fetchMock).toHaveBeenCalledTimes(2)
Project Structure
attom-mcp/
├─ src/
│ ├─ server.ts # Express HTTP wrapper (legacy)
│ ├─ runMcpServer.ts # Transport bootstrap (CLI entry)
│ ├─ mcp/
│ │ ├─ groupedTools.ts # Grouped MCP tools (recommended)
│ │ ├─ mcpServer.ts # MCP core bridge & registration
│ │ └─ tools.ts # Legacy per‑endpoint tools
│ ├─ services/
│ │ └─ attomService.ts # High-level ATTOM orchestrator
│ ├─ utils/
│ │ ├─ fetcher.ts # Retry, logging, cache hook
│ │ └─ fallback.ts # attomId / geoId derivation
│ ├─ config/endpointConfig.ts# Central endpoint map
│ └─ __tests__/ # Vitest specs
├─ openapi/attom-api-schema.yaml
├─ .env.example
└─ tsconfig.json
Development Notes
-
ESM Only – All imports need explicit
.js
when referencing transpiled files. - Dynamic Imports – Used sparingly to avoid circular deps.
-
Logging –
writeLog
writes to stdout; replace with Winston/Pino by swapping util. -
Cache Adapter – Default is
MapCache
; implementRedisCache
by matching the interface inutils/cacheManager.ts
. -
OpenAPI – Regenerate after tool changes:
npm run gen:openapi
.
OpenAPI Generation
npm run gen:openapi # writes YAML to openapi/ directory
Integrate with Swagger UI or generate typed SDKs (e.g. openapi-generator-cli
).
Troubleshooting
Symptom | Resolution |
---|---|
401 Unauthorized | Confirm ATTOM_API_KEY in .env or export globally. |
EMFILE file watch limit | On macOS run sudo sysctl -w kern.maxfiles=524288 and sudo sysctl -w kern.maxfilesperproc=524288 . |
EADDRINUSE :3000 | Set PORT env var to alternate port. |
Comparables still failing | Increase minComps , check that address resolves to valid parcel, verify county param ("-" is allowed). |
License
This project is licensed under the MIT License. You are free to use, modify, and distribute the software in accordance with the license terms.
相关推荐
🔥 1Panel proporciona una interfaz web intuitiva y un servidor MCP para administrar sitios web, archivos, contenedores, bases de datos y LLM en un servidor de Linux.
🧑🚀 全世界最好的 llM 资料总结(数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Resumen de los mejores recursos del mundo.
⛓️Rulego es un marco de motor de regla de orquestación de componentes de alta generación de alto rendimiento, de alto rendimiento y de alto rendimiento para GO.
Traducción de papel científico en PDF con formatos preservados - 基于 Ai 完整保留排版的 PDF 文档全文双语翻译 , 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 等服务 等服务 等服务 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 cli/mcp/docker/zotero
Cree fácilmente herramientas y agentes de LLM utilizando funciones Plain Bash/JavaScript/Python.
😎简单易用、🧩丰富生态 - 大模型原生即时通信机器人平台 | 适配 Qq / 微信(企业微信、个人微信) / 飞书 / 钉钉 / Discord / Telegram / Slack 等平台 | 支持 Chatgpt 、 Deepseek 、 DiFy 、 Claude 、 Gemini 、 Xai 、 PPIO 、 Ollama 、 LM Studio 、阿里云百炼、火山方舟、 Siliconflow 、 Qwen 、 Moonshot 、 Chatglm 、 SillyTraven 、 MCP 等 LLM 的机器人 / Agente | Plataforma de bots de mensajería instantánea basada en LLM, admite Discord, Telegram, WeChat, Lark, Dingtalk, QQ, Slack
Iniciar aplicaciones de múltiples agentes empoderadas con Building LLM de manera más fácil.
Reviews
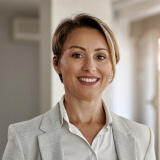
user_4HUthnsv
As a devoted user of the attom-mcp-server developed by jmclaughlin724, I am thoroughly impressed by its performance and reliability. This server application has truly enhanced my workflow efficiency, thanks to its seamless integration and user-friendly interface. It's a robust tool that's easy to set up and provides excellent service for managing and processing data. Highly recommended for anyone looking for a high-quality MCP server solution.