🧑🚀 全世界最好的 llM 资料总结(数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Resumen de los mejores recursos del mundo.
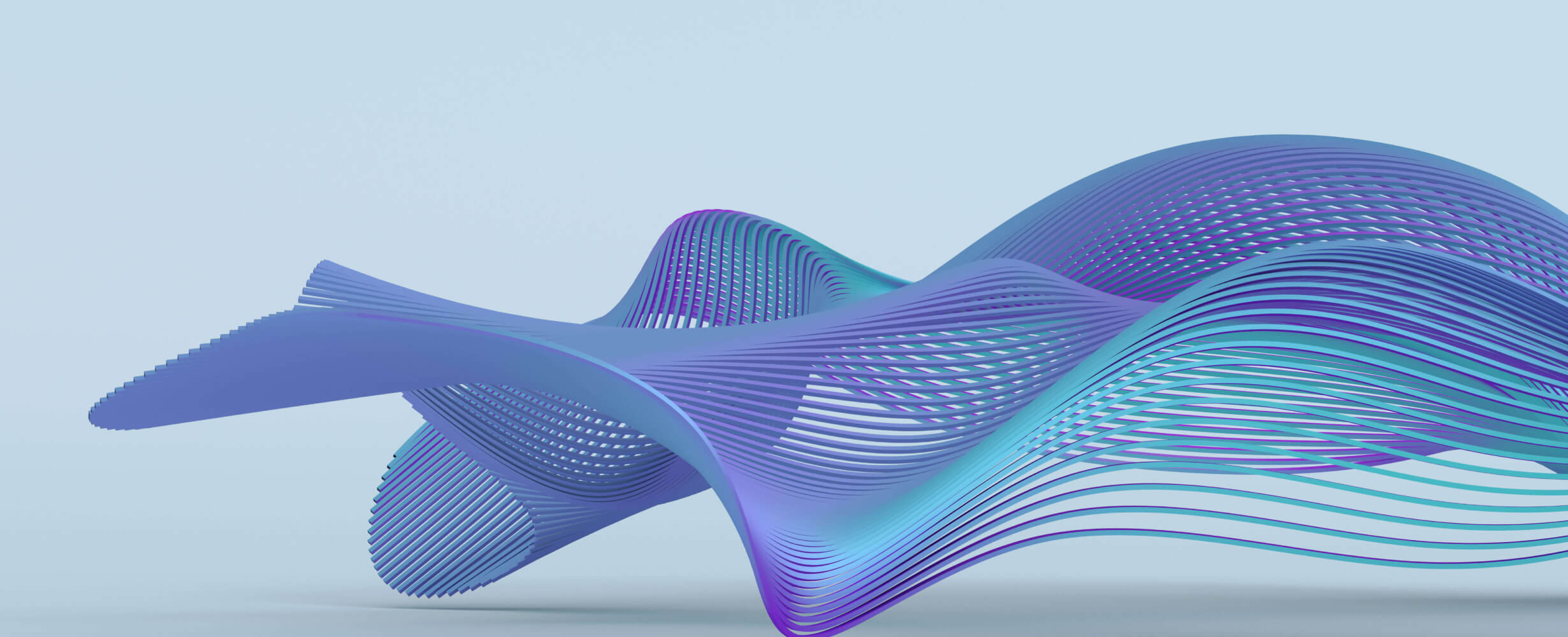
Spotify-MCP-server
Un servidor de protocolo de contexto modelo (MCP) que conecta a los asistentes de IA con la API web de Spotify. Permite el acceso del lenguaje natural a la reproducción de Spotify, la búsqueda, las listas de reproducción y los datos de perfil de usuario, ideal para construir asistentes musicales integrados en LLM.
5
Github Watches
0
Github Forks
5
Github Stars
Kotlin MCP Server for Spotify
A Kotlin implementation of a Model Context Protocol (MCP) server that integrates with the Spotify Web API. This server provides tools for controlling Spotify playback, managing playlists, and retrieving user information through a standardized interface.
Features
- Spotify Authentication: Manages Spotify API tokens and authentication
- Playback Control: Play, pause, skip tracks, seek to position, set volume, and control repeat mode
- Playlist Management: Create playlists, add/remove tracks, and retrieve playlist information
- User Profile: Retrieve user profile information
- MCP Integration: Implements the Model Context Protocol for standardized tool interactions
Prerequisites
- JDK 20 or higher
- Kotlin 2.1.10
- Gradle (wrapper included)
- Spotify Developer Account (for API access)
Installation and Setup
-
Clone the repository:
git clone https://github.com/yourusername/kotlin-mcp-server.git cd kotlin-mcp-server
-
Set up Spotify Developer credentials:
- Go to the Spotify Developer Dashboard
- Log in with your Spotify account or create a new one
- Click "Create an App"
- Fill in the app name and description, then click "Create"
- From your app's dashboard, note your Client ID and Client Secret
- Create a
.env
file in the project root with the following content:
For example:SPOTIFY_CLIENT_ID = "your-client-id" SPOTIFY_CLIENT_SECRET = "your-client-secret"
SPOTIFY_CLIENT_ID = "d4k32j4kl32j4k23j4k23j4k32if" SPOTIFY_CLIENT_SECRET = "5gd6f56fdsd6g5a6d7sd5656cvbx"
Usage
The server can be run in two modes:
-
Standard I/O Mode (default): Uncomment the appropriate line in
Main.kt
to run the server using Ktor with stdio:// In Main.kt fun main() { runMcpServerUsingStdio() // runSseMcpServerUsingKtorPlugin(8080) }
- Run the following command to generate the executable:
This will generate an executable at:./gradlew installDist
build/install/kotlin-mcp-server/bin/kotlin-mcp-server
Connecting with VS Code's Copilot: VS Code's Copilot has a built-in MCP client that can connect to the server when running in Standard I/O mode. You can use other mcp clients like claude desktop but Code's Copilot is the easiest that I found to use. To use it:
- Run the following command to generate the executable:
-
Configure VS Code to connect to the MCP server:
- Open VS Code Settings (Settings > Settings again )
- Search for "mcp" in the settings search bar
- Click on "Edit in settings.json"
- Add the following configuration (adjust the path to match your installation):
{ "security.workspace.trust.untrustedFiles": "open", "terminal.integrated.fontFamily": "MesloLGS Nerd Font", "mcp": { "inputs": [], "servers": { "spotify-mcp-server": { "command": "/Users/karis/IdeaProjects/kotlin-mcp-server/build/install/kotlin-mcp-server/bin/kotlin-mcp-server", "args": [], "env": {} } } }, "git.autofetch": true }
-
In VS Code, open the Copilot chat panel and pick Agent Mode.
-
Copilot will automatically detect and connect to the MCP server
-
You can now interact with the Spotify tools through the Copilot interface
-
Server-Sent Events (SSE) Mode: Uncomment the appropriate line in
Main.kt
to run the server using Ktor with SSE:// In Main.kt fun main() { // runMcpServerUsingStdio() runSseMcpServerUsingKtorPlugin(8080) }
Then run the application and connect to
http://localhost:8080/sse
using an MCP inspector.
Project Structure
-
src/main/kotlin/mcpserver/spotify/
: Core Spotify API integration-
auth/
: Authentication and token management -
services/
: Service implementations for Spotify API endpoints -
utils/
: Utility functions and error handling
-
-
src/main/kotlin/mcpserver/spotifymcp/
: MCP server implementation-
tools/
: Tool implementations for the MCP server
-
Available Tools
The server provides the following tools for interacting with Spotify:
Playback Control
- Pause playback
- Resume playback
- Skip to next track
- Skip to previous track
- Seek to position
- Set volume
- Set repeat mode
- Get current queue
Playlist Management
- Get user playlists
- Get playlist items
- Create playlist
- Add tracks to playlist
- Remove tracks from playlist
User Information
- Get user profile
Development Guidelines
Error Handling
The project uses a custom SpotifyResult<T, E>
sealed class for error handling:
-
SpotifyResult.Success<T>
: Contains successful response data -
SpotifyResult.Failure<E>
: Contains an exception with error details
Use the safeSpotifyApiCall
function to make API calls that return SpotifyResult
objects:
val result = safeSpotifyApiCall<ResponseType, ErrorType> {
// API call code here
}
Adding New Tools
To add a new tool to the MCP server:
- Create a new Kotlin file in the
mcpserver/spotifymcp/tools
package - Define a function that takes a
Server
instance and any required services - Use
server.addTool()
to register the tool with a name, description, and input schema - Implement the tool's functionality in the callback
Testing
Running Tests
# Run all tests
./gradlew test
# Run a specific test class
./gradlew test --tests "mcpserver.spotify.services.playerservice.SpotifyPlayerServiceImplTest"
# Run a specific test method
./gradlew test --tests "mcpserver.spotify.services.playerservice.SpotifyPlayerServiceImplTest.playTrack should return success when API call succeeds"
Writing Tests
The project uses JUnit 5 for testing. Tests should be placed in the src/test/kotlin
directory, mirroring the structure
of the main source code.
For HTTP client mocking, use Ktor's MockEngine
to simulate HTTP responses. When testing services that depend on
external APIs, create mock implementations of dependencies and test both success and failure scenarios.
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
相关推荐
🔥 1Panel proporciona una interfaz web intuitiva y un servidor MCP para administrar sitios web, archivos, contenedores, bases de datos y LLM en un servidor de Linux.
⛓️Rulego es un marco de motor de regla de orquestación de componentes de alta generación de alto rendimiento, de alto rendimiento y de alto rendimiento para GO.
AI's query engine - Platform for building AI that can answer questions over large scale federated data. - The only MCP Server you'll ever need
Reviews
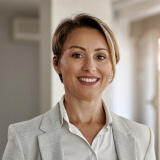
user_TsJDlqrJ
As a dedicated user of MCP applications, I must say spotify-mcp-server by Carrieukie is an exceptional tool. It seamlessly integrates with my multimedia projects and runs efficiently every time. The setup process is straightforward, and the server's performance is top-notch. Highly recommended for anyone seeking a reliable audio management solution!
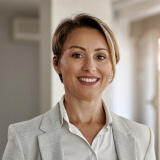
user_8mX7icHP
Spotify-mcp-server by Carrieukie is a game-changer for music enthusiasts like me who love personalized experiences. Its seamless integration and user-friendly interface make managing my playlists and tracks a breeze. Highly recommend it to anyone looking to enhance their Spotify experience!
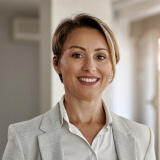
user_RCktLNcC
As a dedicated MCP application user, I absolutely love the spotify-mcp-server by Carrieukie! It seamlessly integrates with my existing setup and offers a smooth, reliable performance. The server is incredibly easy to configure and the documentation provided is clear and concise. If you're looking for a robust solution to enhance your MCP experience, this is the product you need. Highly recommended!
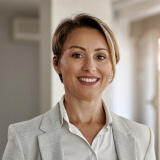
user_XdaLtkqr
As a devoted user of the Spotify-MCP-Server by Carrieukie, I am thoroughly impressed. This innovative server seamlessly integrates with my Minecraft Control Panel, enhancing my gaming experience with immersive music. It is incredibly user-friendly and installation was a breeze. This product truly stands out for anyone looking to merge their Minecraft server with high-quality music streaming. Bravo, Carrieukie!
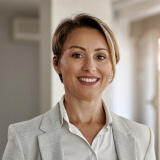
user_GkKIutRy
I've been using spotify-mcp-server by Carrieukie and it's been a game-changer for managing my music streaming experience. The server's seamless integration and ease of use make it perfect for any Spotify enthusiast. Highly recommended!
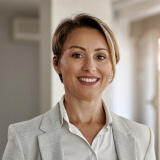
user_QSkjxtsn
I've been using the spotify-mcp-server by Carrieukie and it's simply amazing! The seamless integration with Spotify's API and easy setup process saved me so much time. The server is very stable, and the documentation is clear and concise. If you're looking for a reliable solution for managing your Spotify playlists programmatically, I highly recommend this product!
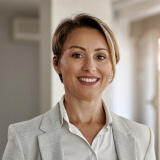
user_4sgKeQr1
I've been using the spotify-mcp-server by Carrieukie and it has significantly improved my music streaming experience. The seamless integration and user-friendly interface make it a standout server. Perfect for anyone looking to enhance their Spotify usage. Highly recommend!
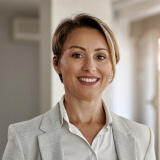
user_OnFR1huY
I am highly impressed with the spotify-mcp-server by Carrieukie! It's a fantastic server-side application that seamlessly integrates Spotify features while maintaining excellent performance. The user experience is top-notch, and it has significantly enhanced my music streaming activities. Highly recommend for any Spotify enthusiast!
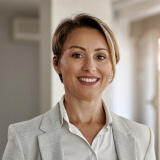
user_DE6KtJTs
As a dedicated user of the MCP application, the spotify-mcp-server by Carrieukie has been phenomenal. It's incredibly efficient and has changed how I experience Spotify. Seamless integration and a user-friendly interface make managing and playing my music a breeze. Highly recommended!
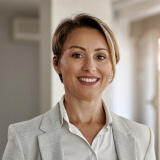
user_2WS80dTP
I have been using spotify-mcp-server by Carrieukie and it has been a game-changer for my music streaming experience. It's seamless, reliable, and enhances my Spotify server functionalities without any hassle. Highly recommend it to anyone looking to elevate their Spotify usage with an efficient and user-friendly solution.
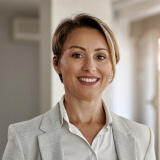
user_Cf206nG0
I am truly impressed with the spotify-mcp-server by Carrieukie! This application is incredibly user-friendly and integrates seamlessly with my music server needs. The setup process was straightforward, and it has significantly optimized my workflow. Highly recommend it to anyone looking for an efficient MCP solution.