Create and Publish Business Websites in seconds. AI will gather all the details about your website and generate link to your website.
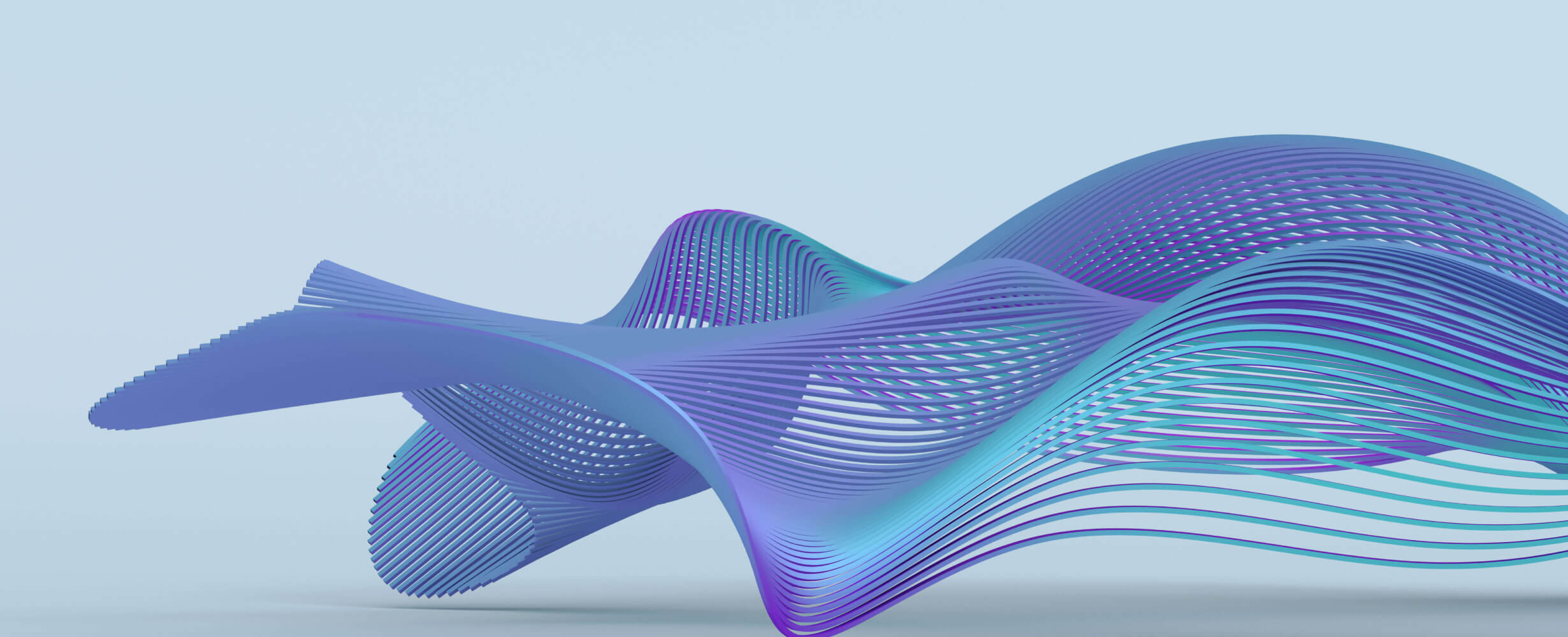
KOI-MCP Integration
A bridging framework that integrates the Knowledge Organization Infrastructure (KOI) with the Model Context Protocol (MCP), enabling autonomous agents to exchange rich personality traits and expose capabilities as standardized tools.
Quick Start
Prerequisites
Installation
# Clone the repository
git clone https://github.com/block-science/koi-mcp.git
cd koi-mcp
# Create and activate virtual environment
uv venv --python 3.12
source .venv/bin/activate # On Windows: .venv\Scripts\activate
# Install the package with development dependencies
uv pip install -e ".[dev]"
Running the Demo
The quickest way to see KOI-MCP in action is to run the demo:
python scripts/demo.py
This provides a rich interactive console with detailed event logging and component status displays.
Alternatively, you can run a simplified demo using the main module:
# Run demo (starts coordinator and two example agents)
python -m koi_mcp.main demo
This starts a coordinator node and two agent nodes with different personality traits. You can then visit:
- Coordinator Registry: http://localhost:9000/resources/list
- Helpful Agent Tools: http://localhost:8101/tools/list
- Creative Agent Tools: http://localhost:8102/tools/list
Running Components Individually
You can also run the components separately:
# Run coordinator node
python -m koi_mcp.main coordinator
# Run agent nodes
python -m koi_mcp.main agent --config configs/agent1.json
python -m koi_mcp.main agent --config configs/agent2.json
Architecture
The KOI-MCP integration follows a Coordinator-Adapter pattern:
flowchart TD
subgraph "Coordinator-Adapter Node"
CN[KOI Coordinator Node]
AD[MCP Adapter]
MC[MCP Context Registry]
end
subgraph "Agent Node A"
A1[KOI Agent Node]
A2[Personality Bundle]
A3[MCP Server]
end
subgraph "Agent Node B"
B1[KOI Agent Node]
B2[Personality Bundle]
B3[MCP Server]
end
CN <-->|Node Discovery| A1
CN <-->|Node Discovery| B1
A1 -->|Personality Broadcast| CN
B1 -->|Personality Broadcast| CN
CN --> AD
AD --> MC
MC -->|Agent Registry| C[LLM Clients]
A3 -->|Tools/Resources| C
B3 -->|Tools/Resources| C
- KOI Coordinator Node: Acts as a central hub for the KOI network, handling agent discovery and state synchronization
- MCP Adapter: Converts KOI personality bundles into MCP-compatible resources and tools
- Agent Nodes: Individual agents with personalities that broadcast their traits to the network
- MCP Registry Server: Exposes the adapter's registry as MCP-compatible endpoints
- MCP Agent Servers: Individual servers for each agent that expose their specific traits as endpoints
Agent Personality Model
Agents express their capabilities through a trait-based personality model:
# Example agent configuration
{
"agent": {
"name": "helpful-agent",
"version": "1.0",
"traits": {
"mood": "helpful",
"style": "concise",
"interests": ["ai", "knowledge-graphs"],
"calculate": {
"description": "Performs simple calculations",
"is_callable": true
}
}
}
}
Each trait can be:
- A simple value (string, number, boolean, list)
- A complex object with metadata (description, type, is_callable)
- A callable tool that can be invoked by LLM clients
Implementation Details
Agent Personality RID
The system extends KOI's Resource Identifier (RID) system with a dedicated AgentPersonality
type:
class AgentPersonality(ORN):
namespace = "agent.personality"
def __init__(self, name, version):
self.name = name
self.version = version
@property
def reference(self):
return f"{self.name}/{self.version}"
Personality Profile Schema
Agent personalities are structured using Pydantic models:
class PersonalityProfile(BaseModel):
rid: AgentPersonality
node_rid: KoiNetNode
base_url: Optional[str] = None
mcp_url: Optional[str] = None
traits: List[PersonalityTrait] = Field(default_factory=list)
Knowledge Processing Pipeline
The system integrates with KOI's knowledge processing pipeline through specialized handlers:
@processor.register_handler(HandlerType.Bundle, rid_types=[AgentPersonality])
def personality_bundle_handler(proc: ProcessorInterface, kobj: KnowledgeObject):
"""Process agent personality bundles."""
try:
# Validate contents as PersonalityProfile
profile = PersonalityProfile.model_validate(kobj.contents)
# Register with MCP adapter if available
if mcp_adapter is not None:
mcp_adapter.register_agent(profile)
return kobj
except ValidationError:
return STOP_CHAIN
MCP Endpoint Integration
The integration provides MCP-compatible REST endpoints:
Coordinator Registry Endpoints
-
GET /resources/list
: List all known agent resources -
GET /resources/read/{resource_id}
: Get details for a specific agent -
GET /tools/list
: List all available agent tools
Agent Server Endpoints
-
GET /resources/list
: List this agent's personality as a resource -
GET /resources/read/agent:{name}
: Get this agent's personality details -
GET /tools/list
: List this agent's callable traits as tools -
POST /tools/call/{trait_name}
: Call a specific trait as a tool
Configuration
Coordinator Configuration
{
"coordinator": {
"name": "koi-mcp-coordinator",
"base_url": "http://localhost:9000/koi-net",
"mcp_registry_port": 9000
}
}
Agent Configuration
{
"agent": {
"name": "helpful-agent",
"version": "1.0",
"base_url": "http://localhost:8100/koi-net",
"mcp_port": 8101,
"traits": {
"mood": "helpful",
"style": "concise",
"interests": ["ai", "knowledge-graphs"],
"calculate": {
"description": "Performs simple calculations",
"is_callable": true
}
}
},
"network": {
"first_contact": "http://localhost:9000/koi-net"
}
}
Advanced Usage
Updating Traits at Runtime
Agents can update their traits dynamically:
agent = KoiAgentNode(...)
agent.update_traits({
"mood": "enthusiastic",
"new_capability": {
"description": "A new capability added at runtime",
"is_callable": True
}
})
Custom Knowledge Handlers
You can register custom handlers for personality processing:
@processor.register_handler(HandlerType.Network, rid_types=[AgentPersonality])
def my_custom_network_handler(proc: ProcessorInterface, kobj: KnowledgeObject):
# Custom logic for determining which nodes should receive personality updates
# ...
return kobj
Development
Running Tests
# Run all tests
pytest
# Run tests with coverage report
pytest --cov=koi_mcp
Project Structure
koi-mcp/
├── configs/ # Configuration files for nodes
├── docs/ # Documentation and design specs
├── scripts/ # Utility scripts
├── src/ # Source code
│ └── koi_mcp/
│ ├── koi/ # KOI integration components
│ │ ├── handlers/ # Knowledge processing handlers
│ │ └── node/ # Node implementations
│ ├── personality/ # Personality models
│ │ ├── models/ # Data models for traits and profiles
│ │ └── rid.py # Agent personality RID definition
│ ├── server/ # MCP server implementations
│ │ ├── adapter/ # KOI-to-MCP adapter
│ │ ├── agent/ # Agent server
│ │ └── registry/ # Registry server
│ ├── utils/ # Utility functions
│ ├── config.py # Configuration handling
│ └── main.py # Main entry point
└── tests/ # Test suite
License
This project is licensed under the MIT License - see the LICENSE file for details.
Acknowledgments
- Built on the KOI-Net library for distributed knowledge organization
- Compatible with the emerging Model Context Protocol (MCP) standard for LLM tool integration
相关推荐
You're in a stone cell – can you get out? A classic choose-your-adventure interactive fiction game, based on a meticulously-crafted playbook. With a medieval fantasy setting, infinite choices and outcomes, and dice!
Text your favorite pet, after answering 10 questions about their everyday lives!
Carbon footprint calculations breakdown and advices on how to reduce it
Best-in-class AI domain names scoring engine and availability checker. Brandability, domain worth, root keywords and more.
Evaluates language quality of texts, responds with a numerical score between 50-150.
Discover A More Robust Business: Craft tailored value proposition statements, develop a comprehensive business model canvas, conduct detailed PESTLE analysis, and gain strategic insights on enhancing business model elements like scalability, cost structure, and market competition strategies. (v1.18)
🧑🚀 全世界最好的 llM 资料总结(数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Resumen de los mejores recursos del mundo.
Este repositorio es para el desarrollo del servidor Azure MCP, llevando el poder de Azure a sus agentes.
🔥 1Panel proporciona una interfaz web intuitiva y un servidor MCP para administrar sitios web, archivos, contenedores, bases de datos y LLM en un servidor de Linux.
Este proyecto fue creado para demostrar cómo podemos conectarnos con diferentes protocolos de contexto del modelo (MCP).
⛓️Rulego es un marco de motor de regla de orquestación de componentes de alta generación de alto rendimiento, de alto rendimiento y de alto rendimiento para GO.
Traducción de papel científico en PDF con formatos preservados - 基于 Ai 完整保留排版的 PDF 文档全文双语翻译 , 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 支持 等服务 等服务 等服务 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 提供 cli/mcp/docker/zotero
Reviews
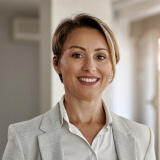
user_YCueg2YV
I've been a dedicated user of koi-mcp by BlockScience and it's genuinely impressive. This tool seamlessly integrates into my workflow, enhancing productivity and offering intuitive features. The interface is user-friendly, and the resources provided are incredibly reliable. Highly recommend for anyone looking for a robust and efficient solution!
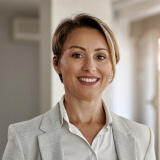
user_ItIDA6c4
As a devoted user of the koi-mcp application by BlockScience, I can say that it truly stands out in its category. The intuitive design and powerful features make managing projects seamless and efficient. Its user-friendly interface and comprehensive toolset streamline workflow like never before. Highly recommended for anyone seeking an intelligent project management solution!
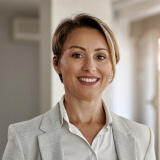
user_iprimRXu
As a devoted user of the koi-mcp application by BlockScience, I am thoroughly impressed with its functionality and performance. The seamless integration and user-friendly interface have significantly enhanced my productivity. The team's commitment to delivering top-notch quality is evident, making it an indispensable tool in my daily workflow. Highly recommended!
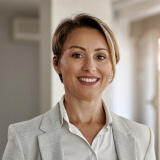
user_Pe8dVyUU
koi-mcp by BlockScience is an exceptional tool for system analysis and modeling. As a devoted user, I appreciate its intuitive interface and robust feature set. It simplifies complex tasks, making it ideal for both new and experienced users. Highly recommended for anyone in need of a reliable MCP application!
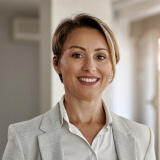
user_NzHaCk0w
Koi-MCP by BlockScience is a fantastic tool that has significantly improved my computational projects. Its intuitive interface and comprehensive features make data processing and analysis a breeze. Highly recommend it to anyone looking for a reliable MCP application.
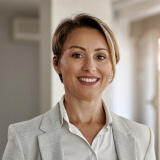
user_7N625z0T
As a dedicated user of the koi-mcp application developed by BlockScience, I must say it's a game changer. The detailed insights and seamless integration make it an indispensable tool for my projects. The user-friendly interface and comprehensive features have significantly enhanced my productivity. Highly recommend it to anyone in need of a robust MCP solution!
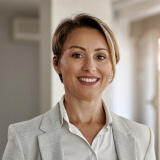
user_bTV2wfud
Koi-MCP by BlockScience is a game-changer! Its seamless integration and user-friendly interface make it indispensable for any user. The features are robust, and I’ve seen significant improvements in my workflow efficiency. Highly recommend for anyone looking to optimize their operations!
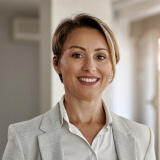
user_ZDmEZ2XD
As an avid user of the koi-mcp application by BlockScience, I am continually impressed by its robust features and seamless performance. This tool simplifies complex processes, making it indispensable for both beginners and experts alike. The user-friendly interface and exceptional support make it a standout in its category. Highly recommend!
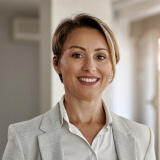
user_jjQGINuI
As a dedicated user of the koi-mcp by BlockScience, I must say this application has truly exceeded my expectations. Its user-friendly interface and seamless integration into my workflow have significantly boosted my productivity. The developers have done an excellent job in creating a tool that is both powerful and easy to use. Highly recommend it for anyone looking to enhance their project management capabilities!
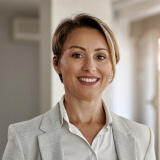
user_Bq7Sp2tN
As a dedicated user of koi-mcp by BlockScience, I find it incredibly useful and efficient. The user interface is intuitive, and the functionality it offers is top-notch. It's clear that a lot of thought has gone into its design, making it a pleasure to use. Highly recommend!
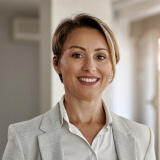
user_tZgSn8pL
As a dedicated user of koi-mcp by BlockScience, I am thoroughly impressed with its robust performance and intuitive design. This application not only simplifies complex project management tasks but also enhances productivity with its seamless interface. Highly recommended for anyone looking to streamline their workflow effectively!
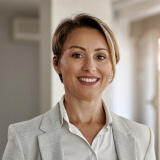
user_ankLlERh
As a dedicated user of koi-mcp by BlockScience, I am thoroughly impressed with its capabilities and performance. The seamless integration and user-friendly interface make it a joy to use. It has significantly improved my workflow, and I highly recommend it to anyone looking for a reliable and efficient MCP application. Well done, BlockScience!