Create and Publish Business Websites in seconds. AI will gather all the details about your website and generate link to your website.
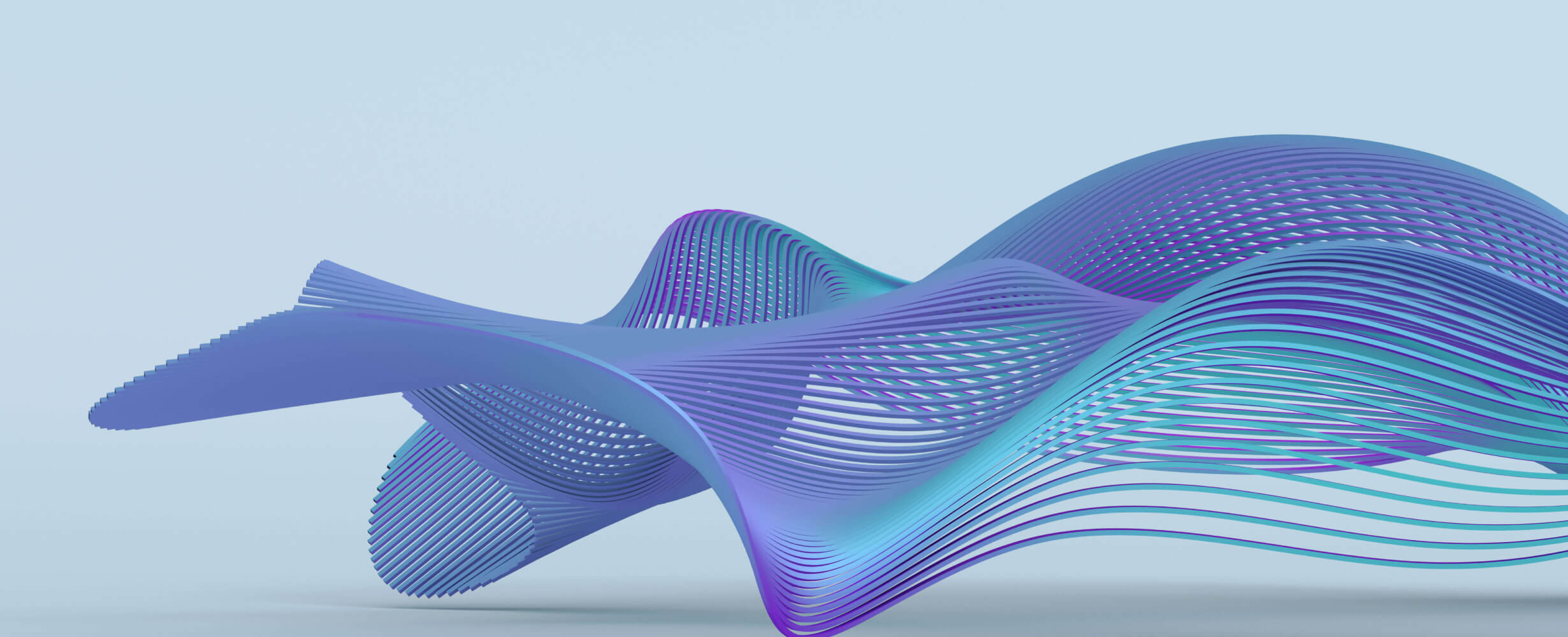
AD-POS-MCP-Server
Aamardokan Pos MCP Server
0
Github Watches
0
Github Forks
0
Github Stars
Aamar Dokan POS MCP Server
A Model Context Protocol (MCP) server for Aamar Dokan POS system, providing a secure middleware connection to MongoDB with analytical capabilities, order management, and AI-assisted prompt generation.
Table of Contents
- Features
- Setup Instructions
- Architecture
- API Documentation
- Development Guide
- Troubleshooting
- Changelog
- License
Features
- 🔒 Secure MongoDB connection
- 📊 Read-only aggregation pipelines with security validation
- 📦 Order lifecycle management
- 🤖 AI-assisted prompt generation for sales operations
- 📈 Business analytics capabilities
- 🔍 Collection exploration and schema discovery
- 📱 Integration with Aamar Dokan POS system
- 🛡️ Type-safe implementation with TypeScript
Setup Instructions
Prerequisites
- Node.js v18+
- npm v9+
- MongoDB v6+
- TypeScript v5+
- MCP SDK v1.9.0
Installation
# Clone repository
git clone https://github.com/manishankarvakta/ad-pos-mcp-server.git
cd ad-pos-mcp-server
# Install dependencies
npm install
# Create environment file
cp .env.example .env
Environment Configuration
Configure your environment variables in the .env
file:
MONGO_URI=mongodb://username:password@hostname:port/database?authSource=admin
Architecture
Project Structure
ad-pos-mcp-server/
├── src/
│ ├── config/
│ │ ├── env.ts # Environment variables
│ │ └── constants.ts # Application constants
│ ├── handlers/
│ │ ├── resource.handler.ts # Collection and resource handlers
│ │ ├── tool.handler.ts # Tool method implementations
│ │ └── prompt.handler.ts # AI prompt generation
│ ├── services/
│ │ └── database.services.ts # MongoDB connection
│ ├── types/
│ │ └── index.ts # TypeScript interfaces
│ ├── global.d.ts # Global type declarations
│ └── index.ts # Entry point
├── .env # Environment variables
├── tsconfig.json # TypeScript configuration
├── package.json # Dependencies and scripts
└── README.md # Documentation
MCP Protocol
The MCP (Model Context Protocol) server implements a standardized interface for AI models to interact with your application data. It provides:
- Resources: Data sources and collections
- Tools: Function-like capabilities for data manipulation
- Prompts: Context-aware template generation
API Documentation
Resource Methods
listCollections
Returns a list of all collections in the connected MongoDB database.
- Parameters: None
- Returns: Array of collection information objects
-
Example:
const collections = await listCollections(); // Returns: [{ name: "orders", ... }, { name: "products", ... }]
getCollectionSchema
Returns a sample document from a collection to help identify its schema.
-
Parameters:
-
collectionName
(string): Name of the collection
-
- Returns: A sample document from the collection
-
Example:
const schema = await getCollectionSchema("orders"); // Returns: { _id: "...", customerId: "...", products: [...], ... }
Tool Methods
createOrder
Creates a new order in the database.
-
Parameters:
-
orderData
(OrderData): Order information including:-
customerId
(string): Customer identifier -
products
(array): Array of products with:-
productId
(string): Product identifier -
quantity
(number): Quantity ordered -
price
(number, optional): Product price
-
-
total
(number, optional): Order total
-
-
- Returns: Created order object with generated orderId and timestamp
-
Example:
const order = await createOrder({ customerId: "CUST-001", products: [ { productId: "PROD-001", quantity: 2, price: 10.99 } ] }); // Returns: { orderId: "ORD-1619364578245", customerId: "CUST-001", ... }
runAggregation
Executes a MongoDB aggregation pipeline on a collection with security validation.
-
Parameters:
-
collection
(string): Name of the collection to query -
pipeline
(array): MongoDB aggregation pipeline stages
-
- Returns: Results of the aggregation operation
-
Security: Blocks dangerous operations like
$out
,$merge
, and$geoNear
-
Example:
const results = await runAggregation("orders", [ { $match: { customerId: "CUST-001" } }, { $group: { _id: "$customerId", total: { $sum: "$total" } } } ]); // Returns: [{ _id: "CUST-001", total: 245.87 }]
Prompt Capabilities
create_sales_order
Generates a prompt for creating a sales order.
-
Parameters:
-
customerId
(string): Customer identifier -
products
(array): Array of products to include in the order
-
- Returns: A prompt message structure
-
Example:
const prompt = await create_sales_order({ customerId: "CUST-001", products: [{ productId: "PROD-001", quantity: 2 }] });
Development Guide
Available Scripts
-
npm run build
- Compile TypeScript to JavaScript -
npm run start
- Start the production server -
npm run dev
- Start development server with hot-reload -
npm run test
- Run test suite
Adding New Methods
To add a new method to the MCP server:
-
Define Types: Add the necessary interfaces in
src/types/index.ts
- Implement the Method: Create implementation in the appropriate handler file
-
Register the Method: Add it to the server in
src/index.ts
Example of adding a new tool method:
// 1. Define the type
interface ProductData {
name: string;
price: number;
category: string;
}
// 2. Implement the method
const createProduct = async (productData: ProductData) => {
const db = await getDatabase();
const result = await db.collection('products').insertOne({
...productData,
createdAt: new Date()
});
return { ...productData, _id: result.insertedId };
};
// 3. Register in index.ts
mcpServer.method('createProduct', createProduct);
Testing
Run tests with npm test
. Add new tests in the test/
directory.
Example test:
describe('createOrder', () => {
it('should create an order with valid data', async () => {
const order = await createOrder({
customerId: 'test',
products: [{ productId: 'p1', quantity: 1 }]
});
expect(order).toHaveProperty('orderId');
expect(order.customerId).toBe('test');
});
});
Coding Standards
- Follow TypeScript best practices with strict type checking
- Use async/await for all database operations
- Add JSDoc comments for all public methods
- Follow the existing project structure
- Validate all inputs before executing database operations
Troubleshooting
Common Issues
- MongoDB Connection Failures: Verify your MongoDB URI and network settings
- Type Errors: Ensure all interfaces are properly defined and used
- MCP SDK Version Conflicts: This project uses MCP SDK v1.9.0
Debug Mode
Set DEBUG=true
in your .env
file for additional logging.
Changelog
v1.0.0 (2024-04-22)
- Initial release with core MCP server functionality
- MongoDB integration for database operations
- Basic resource handlers for collection operations
- Tool handlers for creating orders and running aggregations
- Prompt generation for sales orders
License
ISC License
Copyright (c) 2024 Aamar Dokan
Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies.
相关推荐
Text your favorite pet, after answering 10 questions about their everyday lives!
Carbon footprint calculations breakdown and advices on how to reduce it
You're in a stone cell – can you get out? A classic choose-your-adventure interactive fiction game, based on a meticulously-crafted playbook. With a medieval fantasy setting, infinite choices and outcomes, and dice!
Evaluates language quality of texts, responds with a numerical score between 50-150.
Best-in-class AI domain names scoring engine and availability checker. Brandability, domain worth, root keywords and more.
🧑🚀 全世界最好的 LLM 资料总结 (数据处理、模型训练、模型部署、 O1 模型、 MCP 、小语言模型、视觉语言模型) | Résumé des meilleures ressources LLM du monde.
🔥 1Panel fournit une interface Web intuitive et un serveur MCP pour gérer des sites Web, des fichiers, des conteneurs, des bases de données et des LLM sur un serveur Linux.
⛓️RULEGO est un cadre de moteur de règle d'orchestration des composants de nouvelle génération légère, intégrée, intégrée et de nouvelle génération pour GO.
PDF Traduction de papier scientifique avec formats conservés - 基于 AI 完整保留排版的 PDF 文档全文双语翻译 , 支持 Google / Deepl / Olllama / Openai 等服务 , 提供 CLI / GUI / MCP / DOCKER / ZOTERO
Exécuter des serveurs STDIO de contexte de modèle existant (MCP) dans les fonctions AWS Lambda
Une passerelle basée sur un plugin qui orchestre d'autres MCP et permet aux développeurs de s'appuyer sur des agents de qualité d'entreprise informatiques.
Reviews
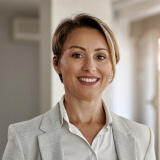
user_BPiBarmr
I’ve been using the AD-POS-MCP-SERVER by manishankarvakta for a while now and it has completely revolutionized our point-of-sale system. The server's seamless performance and reliable features have greatly enhanced our operational efficiency. Highly recommend this robust MCP application for any business looking to streamline their POS operations!
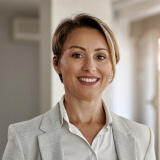
user_65dyBr8L
The AD-POS-MCP-SERVER by manishankarvakta truly stands out in its functionality and ease of use. As a loyal MCP application user, I appreciate its seamless integration and efficient performance. It has greatly simplified my workflow and enhanced productivity. Highly recommended for anyone needing a reliable and robust server solution!
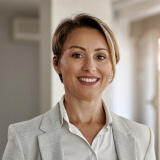
user_5z8CeBPs
As a loyal user of MCP applications, I am highly impressed with the AD-POS-MCP-SERVER by manishankarvakta. The server's robust performance and seamless integration have significantly enhanced our POS system's efficiency. Its user-friendly interface and reliable support make it an invaluable tool for our business operations. Highly recommended!
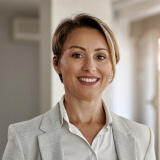
user_vOtdLrVj
I've been extensively using the AD-POS-MCP-SERVER by manishankarvakta, and it has significantly improved our point-of-sale operations. The server is reliable, efficient, and easy to integrate with our existing systems. I'm impressed with its performance and the seamless experience it provides. Highly recommend it to businesses looking for a robust POS solution!