I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
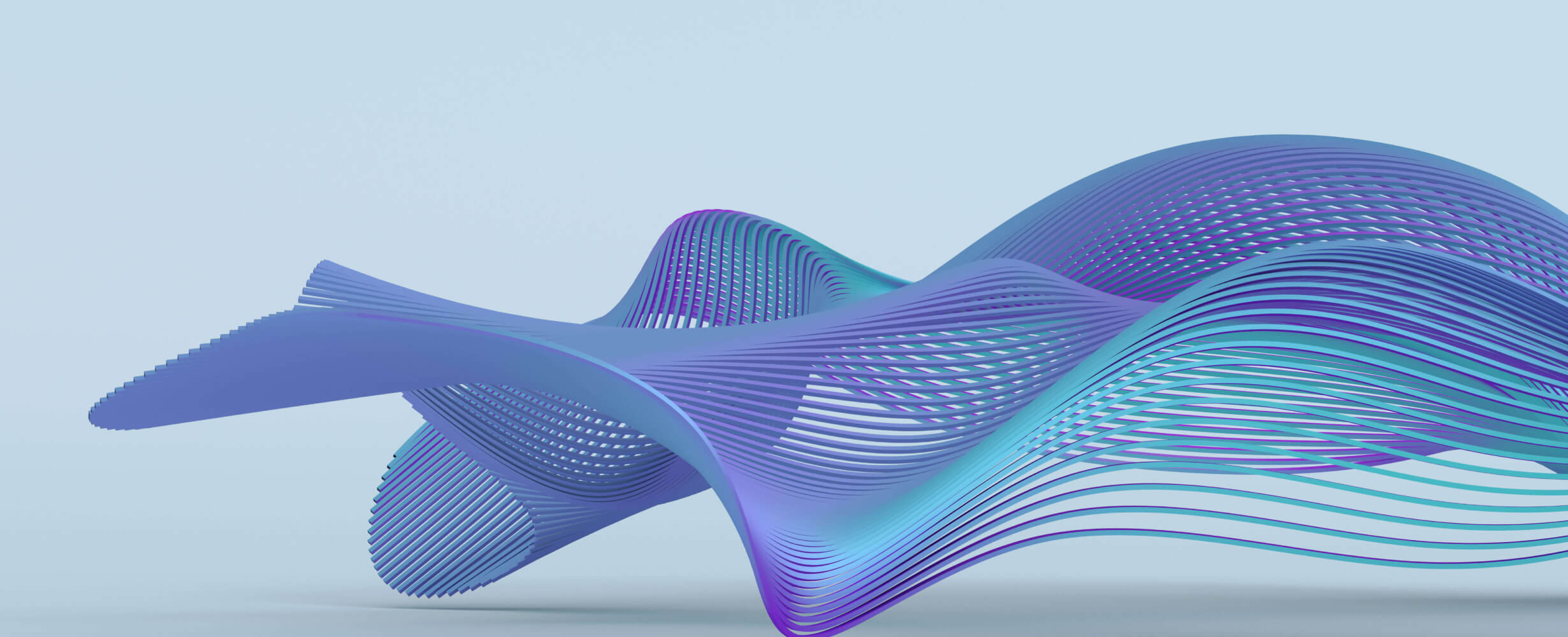
Golang-MCP-Server-SDK
3 years
Works with Finder
1
Github Watches
1
Github Forks
3
Github Stars
Golang MCP Server SDK
Table of Contents
- Overview
- Installation
- Quickstart
- What is MCP?
- Core Concepts
- Running Your Server
- Examples
- Package Structure
- Contributing
- License
Overview
The Model Context Protocol allows applications to provide context for LLMs in a standardized way, separating the concerns of providing context from the actual LLM interaction. This Golang SDK implements the full MCP specification, making it easy to:
- Build MCP servers that expose resources and tools
- Use standard transports like stdio and Server-Sent Events (SSE)
- Handle all MCP protocol messages and lifecycle events
- Follow Go best practices and clean architecture principles
Note: This SDK is always updated to align with the latest MCP specification from spec.modelcontextprotocol.io/latest
Installation
go get github.com/FreePeak/golang-mcp-server-sdk
Quickstart
Let's create a simple MCP server that exposes an echo tool:
package main
import (
"context"
"fmt"
"log"
"os"
"github.com/FreePeak/golang-mcp-server-sdk/pkg/server"
"github.com/FreePeak/golang-mcp-server-sdk/pkg/tools"
)
func main() {
// Create the server
mcpServer := server.NewMCPServer("Echo Server Example", "1.0.0")
// Create an echo tool
echoTool := tools.NewTool("echo",
tools.WithDescription("Echoes back the input message"),
tools.WithString("message",
tools.Description("The message to echo back"),
tools.Required(),
),
)
// Add the tool to the server with a handler
ctx := context.Background()
err := mcpServer.AddTool(ctx, echoTool, handleEcho)
if err != nil {
log.Fatalf("Error adding tool: %v", err)
}
// Start the server
fmt.Println("Starting Echo Server...")
fmt.Println("Send JSON-RPC messages via stdin to interact with the server.")
// Serve over stdio
if err := mcpServer.ServeStdio(); err != nil {
fmt.Fprintf(os.Stderr, "Error: %v\n", err)
os.Exit(1)
}
}
// Echo tool handler
func handleEcho(ctx context.Context, request server.ToolCallRequest) (interface{}, error) {
// Extract the message parameter
message, ok := request.Parameters["message"].(string)
if !ok {
return nil, fmt.Errorf("missing or invalid 'message' parameter")
}
// Return the echo response in the format expected by the MCP protocol
return map[string]interface{}{
"content": []map[string]interface{}{
{
"type": "text",
"text": message,
},
},
}, nil
}
What is MCP?
The Model Context Protocol (MCP) is a standardized protocol that allows applications to provide context for LLMs in a secure and efficient manner. It separates the concerns of providing context and tools from the actual LLM interaction. MCP servers can:
- Expose data through Resources (read-only data endpoints)
- Provide functionality through Tools (executable functions)
- Define interaction patterns through Prompts (reusable templates)
- Support various transport methods (stdio, HTTP/SSE)
Core Concepts
Server
The MCP Server is your core interface to the MCP protocol. It handles connection management, protocol compliance, and message routing:
// Create a new MCP server
mcpServer := server.NewMCPServer("My App", "1.0.0")
Tools
Tools let LLMs take actions through your server. Unlike resources, tools are expected to perform computation and have side effects:
// Define a calculator tool
calculatorTool := tools.NewTool("calculator",
tools.WithDescription("Performs basic arithmetic"),
tools.WithString("operation",
tools.Description("The operation to perform (add, subtract, multiply, divide)"),
tools.Required(),
),
tools.WithNumber("a",
tools.Description("First number"),
tools.Required(),
),
tools.WithNumber("b",
tools.Description("Second number"),
tools.Required(),
),
)
// Add tool to server with a handler
mcpServer.AddTool(ctx, calculatorTool, handleCalculator)
Resources
Resources are how you expose data to LLMs. They're similar to GET endpoints in a REST API - they provide data but shouldn't perform significant computation or have side effects:
// Create a resource (Currently using the internal API)
resource := &domain.Resource{
URI: "sample://hello-world",
Name: "Hello World Resource",
Description: "A sample resource for demonstration purposes",
MIMEType: "text/plain",
}
// Note: Resource support is being updated in the public API
Prompts
Prompts are reusable templates that help LLMs interact with your server effectively:
// Create a prompt (Currently using the internal API)
codeReviewPrompt := &domain.Prompt{
Name: "review-code",
Description: "A prompt for code review",
Template: "Please review this code:\n\n{{.code}}",
Parameters: []domain.PromptParameter{
{
Name: "code",
Description: "The code to review",
Type: "string",
Required: true,
},
},
}
// Note: Prompt support is being updated in the public API
Running Your Server
MCP servers in Go can be connected to different transports depending on your use case:
stdio
For command-line tools and direct integrations:
// Start a stdio server
if err := mcpServer.ServeStdio(); err != nil {
fmt.Fprintf(os.Stderr, "Error: %v\n", err)
os.Exit(1)
}
HTTP with SSE
For web applications, you can use Server-Sent Events (SSE) for real-time communication:
// Configure the HTTP address
mcpServer.SetAddress(":8080")
// Start an HTTP server with SSE support
if err := mcpServer.ServeHTTP(); err != nil {
log.Fatalf("HTTP server error: %v", err)
}
// For graceful shutdown
ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second)
defer cancel()
if err := mcpServer.Shutdown(ctx); err != nil {
log.Fatalf("Server shutdown error: %v", err)
}
Multi-Protocol
You can also run multiple protocol servers simultaneously:
// Configure server for both HTTP and stdio
mcpServer := server.NewMCPServer("Multi-Protocol Server", "1.0.0")
mcpServer.SetAddress(":8080")
mcpServer.AddTool(ctx, echoTool, handleEcho)
// Start HTTP server in a goroutine
go func() {
if err := mcpServer.ServeHTTP(); err != nil {
log.Fatalf("HTTP server error: %v", err)
}
}()
// Start stdio server in the main thread
if err := mcpServer.ServeStdio(); err != nil {
log.Fatalf("Stdio server error: %v", err)
}
Testing and Debugging
For testing your MCP server, you can use the MCP Inspector or send JSON-RPC messages directly:
# Test an echo tool with stdio
echo '{"jsonrpc":"2.0","id":1,"method":"tools/call","params":{"name":"echo","parameters":{"message":"Hello, World!"}}}' | go run your_server.go
Examples
Check out the examples
directory for complete example servers:
Echo Server
A simple echo server example is available in examples/echo_server.go
:
# Run the example
go run examples/echo_server.go
Calculator Server
A more advanced calculator example with both HTTP and stdio modes is available in examples/calculator/
:
# Run in HTTP mode
go run examples/calculator/main.go --mode http
# Run in stdio mode
go run examples/calculator/main.go --mode stdio
Package Structure
The SDK is organized following clean architecture principles:
golang-mcp-server-sdk/
├── pkg/ # Public API (exposed to users)
│ ├── builder/ # Public builder pattern for server construction
│ ├── server/ # Public server implementation
│ ├── tools/ # Utilities for creating MCP tools
│ └── types/ # Shared types and interfaces
├── internal/ # Private implementation details
├── examples/ # Example code snippets and use cases
└── cmd/ # Example MCP server applications
The pkg/
directory contains all publicly exposed APIs that users of the SDK should interact with.
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
License
This project is licensed under the MIT License - see the LICENSE file for details.
📧 Support & Contact
- For questions or issues, email mnhatlinh.doan@gmail.com
- Open an issue directly: Issue Tracker
- If Golang MCP Server SDK helps your work, please consider supporting:
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Descubra la colección más completa y actualizada de servidores MCP en el mercado. Este repositorio sirve como un centro centralizado, que ofrece un extenso catálogo de servidores MCP de código abierto y propietarios, completos con características, enlaces de documentación y colaboradores.
Manipulación basada en Micrypthon I2C del expansor GPIO de la serie MCP, derivada de AdaFruit_MCP230xx
Una puerta de enlace de API unificada para integrar múltiples API de explorador de blockchain similar a Esterscan con soporte de protocolo de contexto modelo (MCP) para asistentes de IA.
Espejo dehttps: //github.com/agentience/practices_mcp_server
Espejo de https: //github.com/bitrefill/bitrefill-mcp-server
Servidor MCP para obtener contenido de la página web con el navegador sin cabeza de dramaturgo.
Reviews
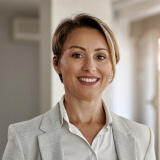
user_IYZFupjl
Fetch MCP Server by MaartenSmeets is a highly reliable and efficient server that I have been using for several months now. The seamless integration and user-friendly interface make it easy to manage my applications effortlessly. Its robust performance and stability have greatly improved my workflow. Highly recommended for anyone looking for a powerful MCP server solution! Check it out at https://mcp.so/server/mcp-server-fetch/MaartenSmeets.