I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
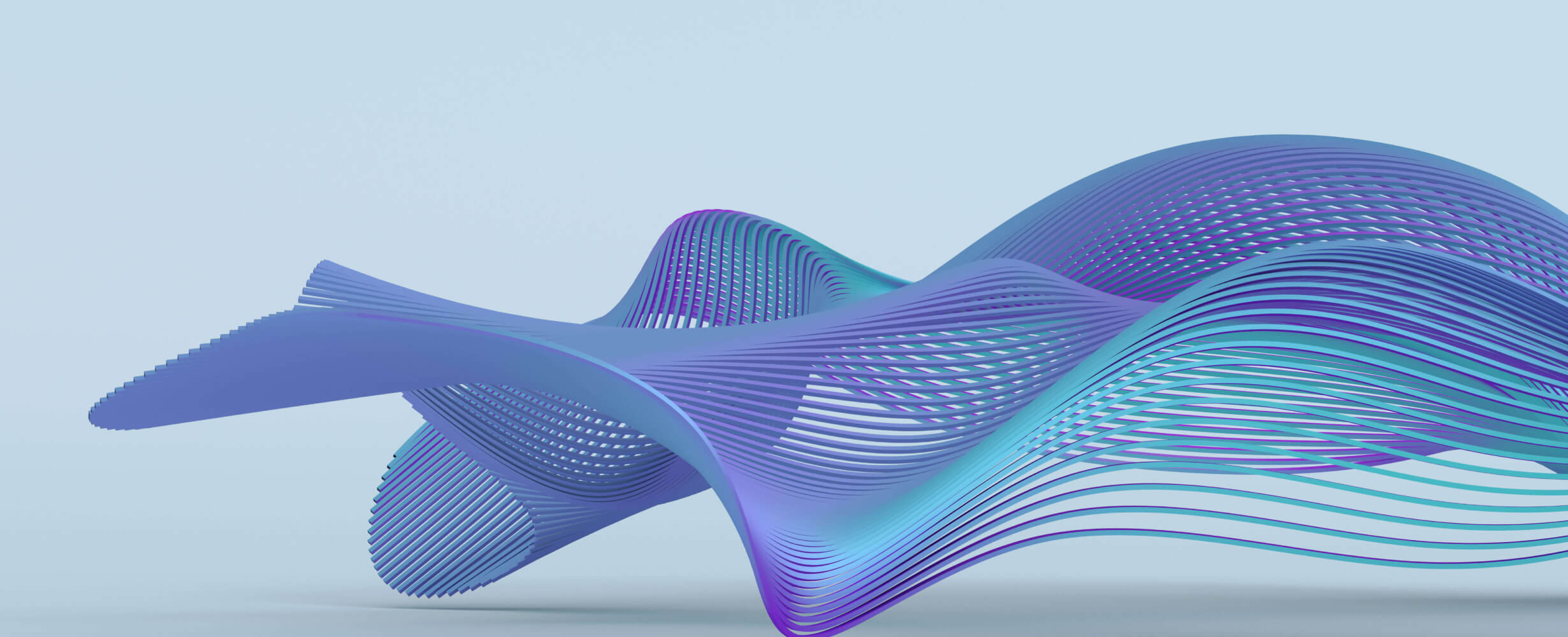
MCP-Go-SDK
MCP Server SDK in Go und einige Server, die ich selbst benutze
3 years
Works with Finder
1
Github Watches
1
Github Forks
3
Github Stars
MCP-Go SDK
A Go SDK for building Model Communication Protocol (MCP) tools and servers. This SDK provides the building blocks for implementing MCP-compliant tools that can be used with AI applications like Cursor IDE.
Quick Start
Check out the example server in servers/example
to see a minimal implementation of an MCP tool that echoes back messages:
package main
import (
"encoding/json"
"mcp-go/server"
"mcp-go/transport"
)
// EchoTool implements a simple echo tool
type EchoTool struct{}
func (t *EchoTool) Name() string {
return "echo"
}
func (t *EchoTool) Description() string {
return "A simple echo tool that returns the input message"
}
func (t *EchoTool) Schema() json.RawMessage {
return json.RawMessage(`{
"type": "object",
"properties": {
"message": {
"type": "string",
"description": "The message to echo back"
}
},
"required": ["message"]
}`)
}
func (t *EchoTool) Execute(params json.RawMessage) (interface{}, error) {
var input struct {
Message string `json:"message"`
}
if err := json.Unmarshal(params, &input); err != nil {
return nil, err
}
return map[string]interface{}{
"content": []map[string]interface{}{
{
"type": "text",
"text": input.Message,
},
},
"metadata": map[string]interface{}{
"length": len(input.Message),
},
}, nil
}
func main() {
// Create a new server with stdin/stdout transport
srv := server.NewServer(transport.NewStdioTransport())
// Register your tool
if err := srv.RegisterTool(&EchoTool{}); err != nil {
panic(err)
}
// Start the server
if err := srv.Start(); err != nil {
panic(err)
}
}
## Core Concepts
### 1. Tools
A Tool in MCP is a service that can be called by AI applications. Each tool must implement the `mcp.Tool` interface:
```go
type Tool interface {
// Name returns the unique identifier for this tool
Name() string
// Description returns a human-readable description
Description() string
// Schema returns the JSON schema for the tool's parameters
Schema() json.RawMessage
// Execute runs the tool with the given parameters
Execute(params json.RawMessage) (interface{}, error)
}
2. Response Format
Tools should return responses in the MCP format:
{
"content": [
{
"type": "text",
"text": "Your response text"
}
],
"metadata": {
// Optional metadata about the response
}
}
3. Transport Layer
The SDK provides a flexible transport layer through the Transport
interface:
type Transport interface {
Send(data interface{}) error
Receive() ([]byte, error)
Close() error
}
By default, the SDK includes a stdio transport (transport.NewStdioTransport()
) for command-line tools.
4. Configuration
To use your MCP tool with Cursor IDE, create a .cursor/mcp.json
in your project root:
{
"mcpServers": {
"mytool": {
"command": "mytool",
"args": [],
"env": {}
}
}
}
Contributing
- Fork the repository
- Create a feature branch
- Add tests for new functionality
- Submit a pull request
Please ensure your code:
- Follows Go best practices
- Includes appropriate documentation
- Has test coverage
- Handles errors appropriately
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
Confidential guide on numerology and astrology, based of GG33 Public information
A geek-themed horoscope generator blending Bitcoin prices, tech jargon, and astrological whimsy.
Converts Figma frames into front-end code for various mobile frameworks.
Therapist adept at identifying core issues and offering practical advice with images.
Advanced software engineer GPT that excels through nailing the basics.
Entdecken Sie die umfassendste und aktuellste Sammlung von MCP-Servern auf dem Markt. Dieses Repository dient als zentraler Hub und bietet einen umfangreichen Katalog von Open-Source- und Proprietary MCP-Servern mit Funktionen, Dokumentationslinks und Mitwirkenden.
Ein einheitliches API-Gateway zur Integration mehrerer Ethercan-ähnlicher Blockchain-Explorer-APIs mit Modellkontextprotokoll (MCP) für AI-Assistenten.
Mirror ofhttps: //github.com/bitrefill/bitrefill-mcp-server
Reviews
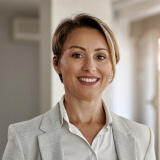
user_jcsiTTo7
As a dedicated user of MCP applications, I recently used Neva developed by RomanEmreis. It's an exceptional tool that enhances productivity with its intuitive interface and robust features. The seamless integration and user-friendly design make it a must-have for anyone looking to optimize their workflow. Highly recommended!